Prepare for your upcoming interview with confidence using our comprehensive DSA Revision Checklist Crash Course. This DSA Crash Course not only helps you brush up on key DSA topics but also includes valuable insights for acing technical interviews. Elevate your technical skills and enhance your interview performance with this essential DSA Crash Course.
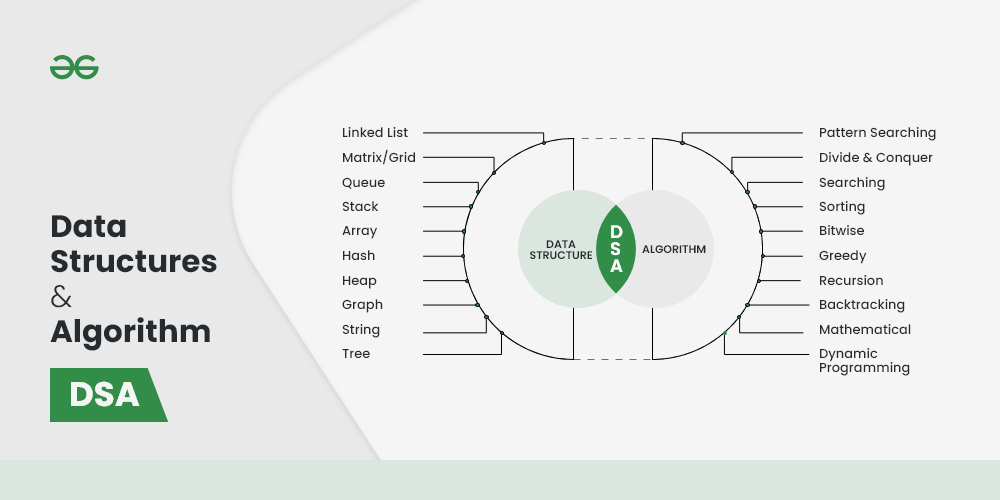
DSA Crash Course
This comprehensive resource offers a meticulous review of crucial Data Structures and Algorithms concepts, serving as the perfect pre-interview refresher. From fundamental data structures to advanced algorithms, this DSA Revision Checklist ensures you’re well-prepared for the technical challenges that lie ahead.
Below are the topics we will be covering in this article:
Data Structures Last Minute Notes | DSA Crash Course
Let us begin with the Data Structures in our DSA Revision Checklist:
An array is a collection of items of same data type stored at contiguous memory locations.
Apart from definitions there are other important topics on Array that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must do problems on Array for this DSA Crash Course:
Apart from these, there are other interview questions on Array that you should know about.
Strings are defined as an array of characters. The difference between a character array and a string is the string is terminated with a special character ‘\0’.
Apart from definitions, there are other important topics on String that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on String for this DSA Crash Course:
Apart from these, there are other interview questions on String that you should know about.
Top Interview Coding Question
A linked list is a linear data structure, Unlike arrays, linked list elements are not stored at a contiguous location. it is basically chains of nodes, each node contains information such as data and a pointer to the next node in the chain. In the linked list there is a head pointer, which points to the first element of the linked list, and if the list is empty then it simply points to null or nothing.
Apart from definitions, there are other important topics on LinkedList that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on the Linked list for this DSA Crash Course:
Apart from these, there are other interview questions on Linked list that you should know about.
Top Interview Coding Question
Stack is a linear data structure in which insertion and deletion are done at one end this end is generally called the top. It works on the principle of Last In First Out (LIFO) or First in Last out (FILO). LIFO means the last element inserted inside the stack is removed first. FILO means, the last inserted element is available first and is the first one to be deleted.
Apart from definitions, there are other important topics on Stack that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Stack for this DSA Crash Course:
Apart from these, there are other interview questions on Stack that you should know about.
Top Interview Coding Question
A Queue is a linear structure that follows a particular order in which the operations are performed. The order is First In First Out (FIFO). It is similar to the ticket queue outside a cinema hall, where the first person entering the queue is the first person who gets the ticket.
Apart from definitions there are other important topics on Queue that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Queue for this DSA Crash Course:
Apart from these, there are other interview questions on Queue that you should know about.
Top Interview Coding Question
A tree is non-linear and a hierarchical data structure consisting of a collection of nodes such that each node of the tree stores a value and a list of references to other nodes (the “children”).
Apart from definitions there are other important topics on Tree that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on tree for this DSA Crash Course:
Binary Search Tree is a node-based binary tree data structure which has the following properties:
- The left subtree of a node contains only nodes with keys lesser than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- The left and right subtree each must also be a binary search tree.
Apart from definitions, there are other important topics on Binary Search Tree that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Binary search tree for this DSA Crash Course:
A Graph is a non-linear data structure consisting of nodes and edges. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph. More formally a Graph can be defined as, A Graph consisting of a finite set of vertices(or nodes) and a set of edges that connect a pair of nodes.
Apart from definitions there are other important topics on Graph that you must prepare/revise before the technical Coding round, such as:
Some important Graph Algorithm:
Along with these, below are a list of must-do problems on Graph for this DSA Crash Course:
Apart from these, there are other interview questions on Array that you should know about.
Top Interview Coding Question
Trie data structure is defined as a Tree based data structure that is used for storing some collection of strings and performing efficient search operations on them. The word Trie is derived from retrieval, which means finding something or obtaining it.
Trie follows some property that If two strings have a common prefix then they will have the same ancestor in the trie. A trie can be used to sort a collection of strings alphabetically as well as search whether a string with a given prefix is present in the trie or not.
Apart from definitions, there are other important topics on Trie that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Trie for this DSA Crash Course:
A Heap is a special Tree-based data structure in which the tree is a complete binary tree.
Apart from definitions, there are other important topics on Heap that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Heap for this DSA Crash Course:
Hashing refers to the process of generating a fixed-size output from an input of variable size using the mathematical formulas known as hash functions. This technique determines an index or location for the storage of an item in a data structure.
Apart from definitions there are other important topics on Hash that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Hash for this DSA Crash Course:
Apart from these, there are other interview questions on Heap that you should know about.
Top Interview Coding Question
Algorithms Last Minute Notes | DSA Crash Course
Let us now dive into the Algorithms in our DSA Revision Checklist:
The process in which a function calls itself directly or indirectly is called recursion and the corresponding function is called a recursive function.
Apart from definitions, there are other important topics on Recursion that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Recursion for this DSA Crash Course:
Backtracking can be defined as a general algorithmic technique that considers searching every possible combination in order to solve a computational problem.
Apart from definitions, there are other important topics on Backtracking that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems on Backtracking for this DSA Crash Course:
Apart from these, there are other interview questions on Backtracking that you should know about.
Top Interview Coding Question
Dynamic Programming (DP) is defined as a technique that solves some particular type of problems in Polynomial Time. Dynamic Programming solutions are faster than the exponential brute method and can be easily proved their correctness.
Apart from definitions, there are other important topics on Dynamic Programming that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems in Dynamic programming for this DSA Crash Course:
Apart from these, there are other interview questions on Dynamic Programming that you should know about.
Top Interview Coding Question
Greedy Algorithm is defined as a method for solving optimization problems by taking decisions that result in the most evident and immediate benefit irrespective of the final outcome. It works for cases where minimization or maximization leads to the required solution.
Apart from definitions, there are other important topics on Greedy Algorithms that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems in Greedy Algorithms for this DSA Crash Course:
Apart from these, there are other interview questions on Greedy Algorithms that you should know about.
Top Interview Coding Question
A Sorting Algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. The comparison operator is used to decide the new order of elements in the respective data structure. Whereas searching is an algorithm to search some elements in the list of elements.
Apart from definitions, there are other important topics on Sorting and Searching that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems in Sorting and Searching for this DSA Crash Course:
Pattern Searching algorithms are used to find a pattern or substring from another bigger string. There are different algorithms. The main goal to design these type of algorithms to reduce the time complexity. The traditional approach may take lots of time to complete the pattern searching task for a longer text.
Apart from definitions, there are other important topics on Pattern Searching that you must prepare/revise before the technical Coding round, such as:
- Algorithms based on character comparison
- Algorithms based on Bit (parallelism method)
- Hashing-string matching algorithms
Along with these, below are a list of must-do problems in Pattern Searching for this DSA Crash Course:
Divide and Conquer is an algorithmic paradigm in which the problem is solved using the Divide, Conquer, and Combine strategy.
Apart from definitions, there are other important topics on Divide and Conquer that you must prepare/revise before the technical Coding round, such as:
Apart from definitions, there are other important topics on Divide and Conquer that you must prepare/revise before the technical Coding round, such as:
Number theory is a branch of pure mathematics devoted to the study of the natural numbers and the integers. It is the study of the set of positive whole numbers which are usually called the set of natural numbers. As it holds the foundational place in the discipline, Number theory is also called “The Queen of Mathematics”.
Apart from definitions, there are other important topics on Number Theory that you must prepare/revise before the technical Coding round, such as:
Along with these, below are a list of must-do problems in Number Theory for this DSA Crash Course:
The Bitwise Algorithms is used to perform operations at the bit-level or to manipulate bits in different ways. The bitwise operations are found to be much faster and are sometimes used to improve the efficiency of a program.
Apart from definitions, there are other important topics on Bit Manipulation that you must prepare/revise before the technical Coding round, such as:
- Knowing all about Bit operators
Along with these, below are a list of must-do problems in Bit Manipulation for this DSA Crash Course:
Related posts:
Some other important Tutorials:
Share your thoughts in the comments
Please Login to comment...