Rotate a Linked List
Last Updated :
20 Feb, 2023
Given a singly linked list, The task is to rotate the linked list counter-clockwise by k nodes.
Examples:
Input: linked list = 10->20->30->40->50->60, k = 4
Output: 50->60->10->20->30->40.
Explanation: k is smaller than the count of nodes in a linked list so (k+1 )th node i.e. 50 becomes the head node and 60’s next points to 10
Input: linked list = 30->40->50->60, k = 2
Output: 50->60->30->40.
Approach: Below is the idea to solve the problem:
To rotate the linked list, we need to change the next pointer of kth node to NULL, the next pointer of the last node should point to the previous head node, and finally, change the head to (k+1)th node. So we need to get hold of three nodes: kth node, (k+1)th node, and last node.
Traverse the list from the beginning and stop at kth node. store k’s next in a tem pointer and point k’s next to NULL then start traversing from tem and keep traversing till the end and point end node’s next to start node and make tem as the new head.
Follow the below steps to implement the idea:
- Initialize a count variable with 0 and pointer kthnode pointing to Null and current pointing to head node.
- Move from current till k-1 and point kthnode to current’s next and current’s next to NULL.
- Move current from kth node to end node and point current’s next to head.
Below image shows how to rotate function works in the code :
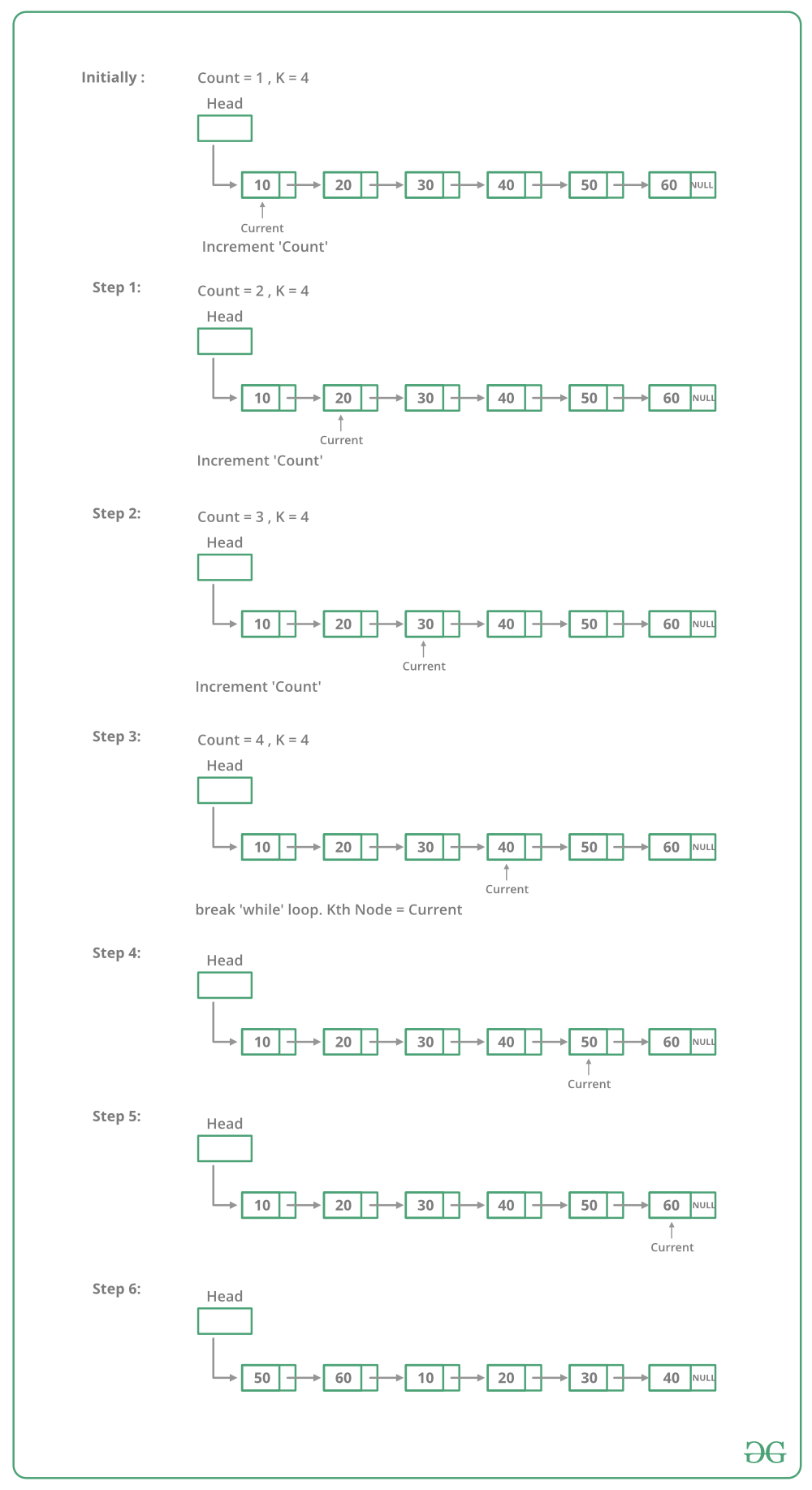
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
class Node {
public :
int data;
Node* next;
};
void rotate(Node** head_ref, int k)
{
if (k == 0)
return ;
Node* current = *head_ref;
int count = 1;
while (count < k && current != NULL) {
current = current->next;
count++;
}
if (current == NULL)
return ;
Node* kthNode = current;
while (current->next != NULL)
current = current->next;
current->next = *head_ref;
*head_ref = kthNode->next;
kthNode->next = NULL;
}
void push(Node** head_ref, int new_data)
{
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList(Node* node)
{
while (node != NULL) {
cout << node->data << " " ;
node = node->next;
}
}
int main( void )
{
Node* head = NULL;
for ( int i = 60; i > 0; i -= 10)
push(&head, i);
cout << "Given linked list \n" ;
printList(head);
rotate(&head, 4);
cout << "\nRotated Linked list \n" ;
printList(head);
return (0);
}
|
C
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void rotate( struct Node** head_ref, int k)
{
if (k == 0)
return ;
struct Node* current = *head_ref;
int count = 1;
while (count < k && current != NULL) {
current = current->next;
count++;
}
if (current == NULL)
return ;
struct Node* kthNode = current;
while (current->next != NULL)
current = current->next;
current->next = *head_ref;
*head_ref = kthNode->next;
kthNode->next = NULL;
}
void push( struct Node** head_ref, int new_data)
{
struct Node* new_node
= ( struct Node*) malloc ( sizeof ( struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList( struct Node* node)
{
while (node != NULL) {
printf ( "%d " , node->data);
node = node->next;
}
}
int main( void )
{
struct Node* head = NULL;
for ( int i = 60; i > 0; i -= 10)
push(&head, i);
printf ( "Given linked list \n" );
printList(head);
rotate(&head, 4);
printf ( "\nRotated Linked list \n" );
printList(head);
return (0);
}
|
Java
class LinkedList {
Node head;
class Node {
int data;
Node next;
Node( int d)
{
data = d;
next = null ;
}
}
void rotate( int k)
{
if (k == 0 )
return ;
Node current = head;
int count = 1 ;
while (count < k && current != null ) {
current = current.next;
count++;
}
if (current == null )
return ;
Node kthNode = current;
while (current.next != null )
current = current.next;
current.next = head;
head = kthNode.next;
kthNode.next = null ;
}
void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
System.out.print(temp.data + " " );
temp = temp.next;
}
System.out.println();
}
public static void main(String args[])
{
LinkedList llist = new LinkedList();
for ( int i = 60 ; i >= 10 ; i -= 10 )
llist.push(i);
System.out.println( "Given list" );
llist.printList();
llist.rotate( 4 );
System.out.println( "Rotated Linked List" );
llist.printList();
}
}
|
Python
class Node:
def __init__( self , data):
self .data = data
self . next = None
class LinkedList:
def __init__( self ):
self .head = None
def push( self , new_data):
new_node = Node(new_data)
new_node. next = self .head
self .head = new_node
def printList( self ):
temp = self .head
while (temp):
print temp.data,
temp = temp. next
def rotate( self , k):
if k = = 0 :
return
current = self .head
count = 1
while (count < k and current is not None ):
current = current. next
count + = 1
if current is None :
return
kthNode = current
while (current. next is not None ):
current = current. next
current. next = self .head
self .head = kthNode. next
kthNode. next = None
llist = LinkedList()
for i in range ( 60 , 0 , - 10 ):
llist.push(i)
print "Given linked list"
llist.printList()
llist.rotate( 4 )
print "\nRotated Linked list"
llist.printList()
|
C#
using System;
public class LinkedList {
Node head;
public class Node {
public int data;
public Node next;
public Node( int d)
{
data = d;
next = null ;
}
}
void rotate( int k)
{
if (k == 0)
return ;
Node current = head;
int count = 1;
while (count < k && current != null ) {
current = current.next;
count++;
}
if (current == null )
return ;
Node kthNode = current;
while (current.next != null )
current = current.next;
current.next = head;
head = kthNode.next;
kthNode.next = null ;
}
void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
Console.Write(temp.data + " " );
temp = temp.next;
}
Console.WriteLine();
}
public static void Main()
{
LinkedList llist = new LinkedList();
for ( int i = 60; i >= 10; i -= 10)
llist.push(i);
Console.WriteLine( "Given list" );
llist.printList();
llist.rotate(4);
Console.WriteLine( "Rotated Linked List" );
llist.printList();
}
}
|
Javascript
<script>
var head;
class Node {
constructor(val) {
this .data = val;
this .next = null ;
}
}
function rotate(k) {
if (k == 0)
return ;
var current = head;
var count = 1;
while (count < k && current != null ) {
current = current.next;
count++;
}
if (current == null )
return ;
var kthNode = current;
while (current.next != null )
current = current.next;
current.next = head;
head = kthNode.next;
kthNode.next = null ;
}
function push(new_data) {
var new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
function printList() {
var temp = head;
while (temp != null ) {
document.write(temp.data + " " );
temp = temp.next;
}
document.write( "<br/>" );
}
for (i = 60; i >= 10; i -= 10)
push(i);
document.write( "Given list<br/>" );
printList();
rotate(4);
document.write( "Rotated Linked List<br/>" );
printList();
</script>
|
Output
Given linked list
10 20 30 40 50 60
Rotated Linked list
50 60 10 20 30 40
Time Complexity: O(N), where N is the number of nodes in Linked List.
Auxiliary Space: O(1)
Another Approach: Rotate the linked list k times by placing the first element at the end.
The idea is to traverse the given list to find the last element and store it in a node. Now we need to make the next of last element as the current head, which we can do by storing head in temporary node. Repeat the process k time.
Follow the steps below to implement the above idea:
- Return head if the head is NULL or k=0.
- Initialize a node last and make it point to the last node of the given list.
- Make a temporary node pointing to head.
- while k>0 run a loop :
- make temp as last node and head point to next of head.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
class Node {
public :
int data;
Node* next;
};
Node* rotate(Node* head, int k)
{
Node* last = head;
Node* temp = head;
if (head == NULL || k == 0) {
return head;
}
while (last->next != NULL) {
last = last->next;
}
while (k) {
head = head->next;
temp->next = NULL;
last->next = temp;
last = temp;
temp = head;
k--;
}
return head;
}
void printList(Node* n)
{
while (n != NULL) {
cout << n->data << " " ;
n = n->next;
}
cout << endl;
}
void push(Node** head_ref, int new_data)
{
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
int main()
{
Node* head = NULL;
for ( int i = 60; i > 0; i -= 10)
push(&head, i);
cout << "Given linked list \n" ;
printList(head);
head = rotate(head, 4);
cout << "\nRotated Linked list \n" ;
printList(head);
return 1;
}
|
Java
public class LinkedList {
Node head;
class Node {
int data;
Node next;
Node( int d)
{
data = d;
next = null ;
}
}
void rotate( int k)
{
Node last = head;
Node temp = head;
if (head == null || k == 0 ) {
return ;
}
while (last.next != null ) {
last = last.next;
}
while (k != 0 ) {
head = head.next;
temp.next = null ;
last.next = temp;
last = temp;
temp = head;
k--;
}
}
void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
System.out.print(temp.data + " " );
temp = temp.next;
}
System.out.println();
}
public static void main(String args[])
{
LinkedList llist = new LinkedList();
for ( int i = 60 ; i >= 10 ; i -= 10 )
llist.push(i);
System.out.println( "Given list" );
llist.printList();
llist.rotate( 4 );
System.out.println( "\nRotated Linked List" );
llist.printList();
}
}
|
Python3
class Node:
def __init__( self ,data):
self .data = data
self . next = None
def rotate(head,k):
last = head
temp = head
if head = = None or k = = 0 :
return head
while last. next ! = None :
last = last. next
while k:
head = head. next
temp. next = None
last. next = temp
last = temp
temp = head
k - = 1
return head
def printList(head):
temp = head
while temp:
print (temp.data, end = ' ' )
temp = temp. next
print ()
def push(head,new_data):
new_node = Node(new_data)
new_node. next = head
head = new_node
return head
head = None
for i in range ( 60 , 0 , - 10 ):
head = push(head,i)
print ( "Given linked list: " )
printList(head)
head = rotate(head, 4 )
print ( "Rotated linked list: " )
printList(head)
|
C#
using System;
public class LinkedList {
Node head;
public class Node {
public int data;
public Node next;
public Node( int d)
{
data = d;
next = null ;
}
}
void rotate( int k)
{
Node last = head;
Node temp = head;
if (head == null || k == 0) {
return ;
}
while (last.next != null ) {
last = last.next;
}
while (k != 0) {
head = head.next;
temp.next = null ;
last.next = temp;
last = temp;
temp = head;
k--;
}
}
void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
Console.Write(temp.data + " " );
temp = temp.next;
}
Console.WriteLine( "\n" );
}
public static void Main()
{
LinkedList llist = new LinkedList();
for ( int i = 60; i >= 10; i -= 10)
llist.push(i);
Console.WriteLine( "Given list" );
llist.printList();
llist.rotate(4);
Console.WriteLine( "Rotated Linked List" );
llist.printList();
}
}
|
Javascript
var head;
class Node{
constructor(val){
this .data = val;
this .next = null ;
}
}
function rotate(k)
{
var last = head;
var temp = head;
if (head == null || k == 0) return head;
while (last.next != null )
last = last.next;
while (k != 0){
head = head.next;
temp.next = null ;
last.next = temp;
last = temp;
temp = head;
k--;
}
}
function printList() {
var temp = head;
while (temp != null ) {
document.write(temp.data + " " );
temp = temp.next;
}
console.log( "\n" );
}
function push(new_data){
var new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
for (let i = 60; i >= 10; i -= 10)
push(i);
console.log( "Given list : \n" );
printList();
rotate(4);
console.log( "Rotated Linked List : \n" );
printList();
|
Output
Given linked list
10 20 30 40 50 60
Rotated Linked list
50 60 10 20 30 40
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...