Java Exercises – Basic to Advanced Java Practice Programs with Solutions
Last Updated :
18 Apr, 2024
Test your Java skills with our topic-wise Java exercises. As we know Java is one of the most popular languages because of its robust and secure nature. But, programmers often find it difficult to find a platform for Java Practice Online. In this article, we have provided Java Practice Programs. That covers various Java Core Topics that can help users with Java Practice.
Take a look at our free Java Exercises to practice and develop your Java programming skills. Our Java programming exercises Practice Questions from all the major topics like loops, object-oriented programming, exception handling, and many more.
-768.webp)
Java Practice Programs
This Java exercise is designed to deepen your understanding and refine your Java coding skills, these programs offer hands-on experience in solving real-world problems, reinforcing key concepts, and mastering Java programming fundamentals. Whether you’re a beginner who looking to build a solid foundation or a professional developer aiming to sharpen your expertise, our Java practice programs provide an invaluable opportunity to sharpen your craft and excel in Java programming language.
1. Write Hello World Program in Java
The solution to the Problem is mentioned below:
Java
// Java Program to Print
// Hello World
import java.io.*;
// Driver Class
class GFG {
// main function
public static void main(String[] args)
{
// Printing Hello World
System.out.println("Hello World!");
}
}
2. Write a Program in Java to Add two Numbers.
Input: 2 3
Output: 5
3. Write a Program to Swap Two Numbers
Input: a=2 b=5
Output: a=5 b=2
4. Write a Java Program to convert Integer numbers and Binary numbers.
Input: 9
Output: 1001
5. Write a Program to Find Factorial of a Number in Java.
Input: 5
Output: 120
6. Write a Java Program to Add two Complex Numbers.
Input: 1+2i
4+5i
Output: 5+7i
7. Write a Program to Calculate Simple Interest in Java
Input : P = 10000 R = 5 T = 5
Output : 2500
8. Write a Program to Print the Pascal’s Triangle in Java
Input : N = 5
Output:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
9. Write a Program to Find Sum of Fibonacci Series Number
Input: n = 4
Output: 33
Explaination: Sum of numbers at even indexes = 0 + 1 + 3 + 8 + 21 = 33.
Pattern Programs in Java
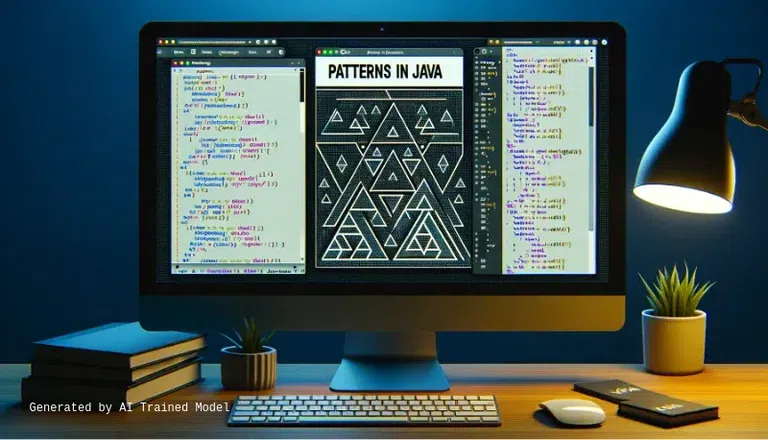
10. Write a Program to Print Pyramid Number Pattern in Java.
*
***
*****
*******
11. Write a Java Program to Print Pattern.
* * * * * *
* *
* *
* *
* *
* * * * * *
12. Write a Java Program to Print Pattern.
1
2 1 2
3 2 1 2 3
4 3 2 1 2 3 4
5 4 3 2 1 2 3 4 5
6 5 4 3 2 1 2 3 4 5 6
13. Java Program to Print Patterns.
*
***
*****
*******
*********
***********
*********
*******
*****
***
*
Array Programs in Java
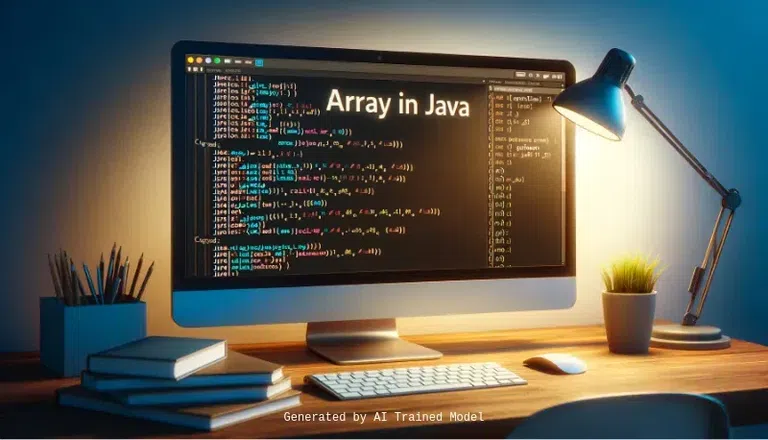
14. Write a Java Program to Compute the Sum of Array Elements.
Input: [ 2, 4, 6, 7, 9]
Output: 28
15. Write a Java Program to Find the Largest Element in Array
Input: [ 7, 2, 5, 1, 4]
Output: 7
16. Write Java Program to Find the Tranpose of Matrix
Input:
[ [ 1, 2, 3 ]
[ 4, 5, 6 ]
[ 7, 8, 9 ] ]
Output:
[ [ 1, 4, 7]
[ 2, 5, 8]
[ 3, 6, 9] ]
17. Java Array Program For Array Rotation
Input: arr[] = {1, 2, 3, 4, 5, 6, 7}, d = 2
Output:Â 3 4 5 6 7 1 2
Explaination: d=2 so 2 elements are rotated to the end of the array. So, 1 2 is rotated back
So, Final result: 3, 4, 5, 6, 7, 1, 2
18. Java Array Program to Remove Duplicate Elements From an Array
Input: [ 1, 2, 2, 3, 3, 3, 4, 5 ]
Output: [ 1, 2, 3, 4, 5 ]
19. Java Array Program to Remove All Occurrences of an Element in an Array
Input: array = [ 1, 2, 1, 3, 5, 1 ] , key = 1
Output: [2, 3, 5]
Explaination: all the occurrences of element 1 is removed from the array So, array becomes from
[ 1, 2, 1, 3, 5, 1 ] to
Final result: [2, 3, 5]
String Programs in Java
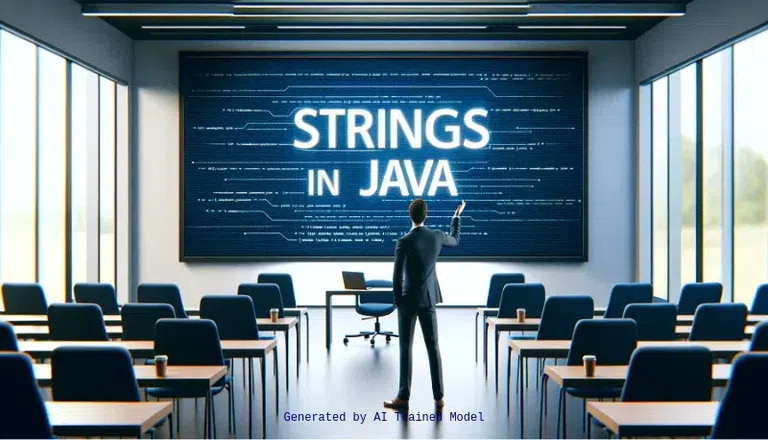
20. Java program to check whether a string is a Palindrome
Input: "racecar"
Output: Yes
Explaination: As racerar after reversing becomes racecar. As Reverse of String is same as String so it is Palindrome
21. Java String Program to Check Anagram
Input: str1 = "Silent"
str2 ="Listen"
Output: Strings are Anagram
Explaination: As all the elements in str1 are exact same as to create str2 .
i.e., we can create str2 using elements of str1 without removing any element or removing any extra element.
22. Java String Program to Reverse a String
Input: str= "Geeks"
Output: "skeeG"
23. Java String Program to Remove leading zeros
Input :Â 00000123569
Output :Â 123569
Java Practice Problems for Searching Algorithms
-768.webp)
24. Write a Java Program for Linear Search.
Time Complexity: O(N)
Space Complexity: O(N)
25. Write a Binary Search Program in Java.
Time Complexity: O(logN)
Space Complexity: O(N)
Practice Problems in Java Sorting Algorithms
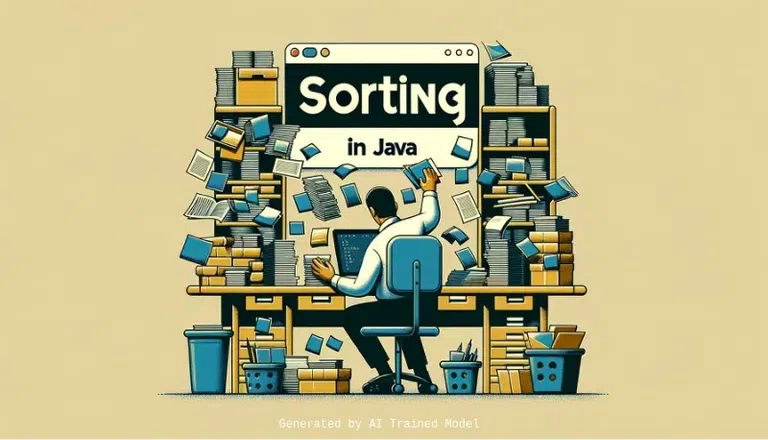
26. Java Program for Bubble Sort.
Time Complexity: O(N2)
Space Complexity: O(1)
27. Write a Program for Insertion Sort in Java.
Time Complexity: O(N2)
Space Complexity: O(1)
28. Java Program for Selection Sort.
Time Complexity: O(N2)
Space Complexity: O(1)
29. Java Program for Merge Sort.
Time Complexity: O(N logN)
Space Complexity: O(N)
30. Java Program for QuickSort.
Time Complexity: O(N logN)
Space Complexity: O(1)
Conclusion
After completing these Java exercises you are a step closer to becoming an advanced Java programmer. We hope these exercises have helped you understand Java better and you can solve beginner to advanced-level questions on Java programming.
Solving these Java programming exercise questions will not only help you master theory concepts but also grasp their practical applications, which is very useful in job interviews.
More Java Practice Exercises
FAQ in Java Exercise
1. How to do Java projects for beginners?
To do Java projects you need to know the fundamentals of Java programming. Then you need to select the desired Java project you want to work on. Plan and execute the code to finish the project. Some beginner-level Java projects include:
- Reversing a String
- Number Guessing Game
- Creating a Calculator
- Simple Banking Application
- Basic Android Application
2. Is Java easy for beginners?
As a programming language, Java is considered moderately easy to learn. It is unique from other languages due to its lengthy syntax. As a beginner, you can learn beginner to advanced Java in 6 to 18 months.
3. Why Java is used?
Java provides many advantages and uses, some of which are:
- Platform-independent
- Robust and secure
- Object-oriented
- Popular & in-demand
- Vast ecosystem
Share your thoughts in the comments
Please Login to comment...