Hibernate in Java is a powerful, high-performance Object/Relational Mapping (ORM) framework that greatly simplifies the development of Java applications to interact with databases. In this article, we present the top Hibernate Interview Questions that are commonly asked in interviews.
In this article, we will cover basic, intermediate, and advanced questions for candidates with years of experience in the form of Hibernate in Java Interview Questions.
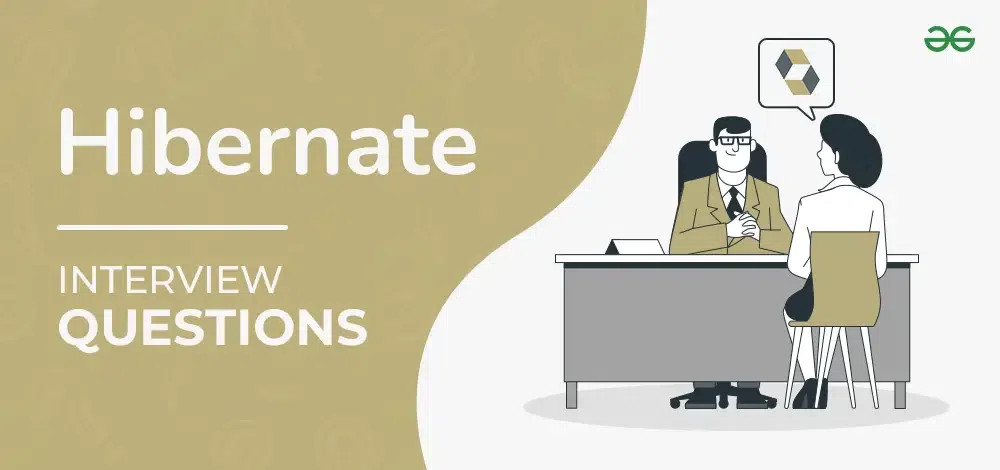
History of Hibernate
Hibernate is an open-source Java-based framework that revolutionizes the way of interaction with databases for Java developers. It was developed in 2001 by Gavin King. Hibernate is a very powerful Object-Relational Mapping (ORM) framework for Java applications that allows the developer to work with such data objects rather than hustling with raw SQL queries.
Hibernate Interview Questions For Freshers
1. What is Hibernate?
Hibernate is an open-source framework that makes it easier to store information in a relational database. It converts Java objects to corresponding database tables easily with ORM. It makes automatic database operations by CRUD (Create, Read, Update, Delete) operations. Thus no need to write SQL queries as Hibernate provides a seamless mechanism and better interacting features.
2. Advantages of using Hibernate?
- Hibernate prevents data corruption as it ensures that the changes to the database are completely applied.
- Hibernate provides its query language (HQL) which is similar to SQL.
- HQL speeds up the performance and generates automatic database tables based on the Java class structure.
3. What is ORM in Hibernate?
ORM, which stands for Object-Relational Mapping acts as a translator that converts the Java object into the relational database. It makes it easier to store and retrieve information on Java objects, by using the ORM technique which reduces a lot of manual work.
4. What are the advantages of Hibernate over JDBC?
Feature
| Hibernate
| JDBC
|
---|
Object-Relational Mapping | Automatic | Manual |
---|
Coding effort | Less | More |
---|
Database Portability | High | Low |
---|
Query Caching | Yes | No |
---|
Transaction Management | Automatic | Manual |
---|
5. What are some of the important interfaces of the Hibernate framework?
- The SessionFactory creates and manages the sessions that are occurring over a short conversation between your program and the database.
- The session object provides an interface between the application and data stored in the database. It holds the first level cache(mandatory) of data. Sessions are the most important interfaces in the hibernate framework that gives you a tool like adding the data, changing the data, or getting data from the database and once you are done with the session, you need to close this session.
- Transaction interface represents a database transaction including the committing changes to the database or the rollback.
- The query interface is used for creating and executing the queries and retrieving the information from the database.
- SessionFactoryBuilder is used to build a SessionFactory instance based on configuration settings for a new SessionFactory.
6. List some of the databases supported by Hibernate.
- MySQL
- Oracle
- PostgreSQL
- H2
- DB2
- Microsoft SQL Server
- Sybase
- SQLite
7. What is Java Persistence API (JPA)?
Java Persistence API is a collection of classes and methods to persist or store a vast amount of data in a database using ORM. JPA Persistence framework needs to follow:
- Spring Data JPA: It reduces the amount of boilerplate code needed for common database operations like GET, PUT, POST, etc.
- Spring Repository: It is an extension of Spring Repository which contains APIs for basic CRUD operations, pagination, and Sorting.
8. Explain the concept behind Hibernate Inheritance Mapping.
Inheritance Mapping in Hibernate is used for mapping object-oriented inheritance relationships into relational databases. It also ensures the structure of class hierarchies in programming while storing data in database tables. Hibernate uses the below tables for managing the mapping.
- Single Table
- Table Per Class
- Joined Table Inheritance
9. What is HQL?
HQL stands for Hibernate Query Language it allows to expression of database queries using entity and property names, rather than relying on traditional SQL that operates on tables and columns. It uses an object-oriented way to operate with Java entity classes and properties.
HQL queries could be dynamic and flexible which supports the aggregation and joins as well such as SUM, and AVG, and uses joins to combine data from the different tables. HQL supports polymorphic queries, meaning you can write a single query that operates on a superclass and retrieves instances of both the superclass and its subclasses.
10. How to create HQL Queries?
- First, create an HQL Query String including entity name, property name, and relationships in the query.
- Create a query Object by using org.hibernate.query.Query interface for creating and executing queries.
- Also, query can be created by using createQuery()
- Set Parameters using setParameter() is optional.
- Execute the Query using methods like list() to retrieve the data or listing of results.
- uniqueResult() for retrieving a single result.
Session session = sessionFactory.openSession();
try {
String hql = "FROM Employee WHERE department.name = :deptName";
Query<Employee> query = session.createQuery(hql, Employee.class);
query.setParameter("deptName", "Engineering");
List<Employee> employees = query.list();
for (Employee employee : employees) {
System.out.println("Employee Name: " + employee.getName());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
session.close();
}
11. How can we add criteria to a SQL Query?
To add criteria in hibernate we use criteria APIs that build queries by using a session object-oriented approach and directly add criteria to a query in hibernate.
Session session = sessionFactory.openSession();
try {
Criteria criteria = session.createCriteria(Product.class);
// Add criteria to filter products by category and price range
criteria.add(Restrictions.eq("category", "Programming"));
criteria.add(Restrictions.between("price", 100.0, 500.0));
// Execute the query and retrieve the list of products
List<Product> products = criteria.list();
for (Product product : products) {
System.out.println("Product Name: " + product.getName());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
session.close();
}
sessionFactory.close();
12. What is a Session in Hibernate?
Session represents the single unit of work that acts as a gateway for interacting with the databases, Hibernate session is the primary interface for working with databases. Session provides various important functions such as
- Transaction Management
- Caching
- Lazy Loading
- Data Retrieval Strategies
- Object Persistence and Retrieval
It also handles Data Relationship Handling where developers can manage and define relationships between objects, specifying fetching strategies, and overflow of behaviors.
13. What is SessionFactory?
SessionFactory in Hibernate is to create and manage the session instances that-
- configure the management by reading
- managing the configuration setting of the hibernate environment
- database connection
- mapping metadata (the data inside the data called metadata)
- caching configurations.
When you create the SessionFactory it is shared among multiple threads within the application where each thread acquires its own Session instance shared from the SessionFactory.
NOTE: SessionFactory is thread-safe but Sessions is not thread-safe.
The below which given code initializes a Hibernate SessionFactory for database connectivity configuration.
Java
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory;
static
{
try {
// Create the SessionFactory using the
// hibernate.cfg.xml configuration
sessionFactory
= new Configuration()
.configure("hibernate.cfg.xml")
.buildSessionFactory();
}
catch (Throwable ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory()
{
return sessionFactory;
}
}
14. Comment on “Session being a thread-safe object”?
The statement Session being a thread-safe object isn’t correct. In Hibernate Session instance is not thread-safe by default, but a SessionFactory is thread-safe, this means it should not share a single Session instance over multiple threads concurrently that may cause data corruption.
- The session thread is not inherently thread-safe.
- Thread should manage its own dedicated Session instance to avoid potential issues and data inconsistencies.
15. What is the difference between session.save() and session.persist() method?
Properties | session.save() | session.persist() |
---|
Return Value
| It will give a generated identifier.
| It will not generate anything.
|
---|
Identifier Generation
| It is necessary for non-assigned IDs.
| It is not necessary and can be assigned directly.
|
---|
Transient Instances
| It will be detached or transient instance.
| It should be a Transient instance.
|
---|
Cascade Operations
| It can’t cascade on its own.
| It can be cascaded.
|
---|
Managed State
| It will give the controlled instance.
| It will be rest as transient.
|
---|
Flush Immediate
| It will give you an instant SQL INSERT.
| It will not have instant SQL operation.
|
---|
Unsaved Transient State
| It is not secured with unsaved transient instances.
| It is secured with unsaved transient instances.
|
---|
Inverse Associations
| It will be cascaded to reverse end of associations.
| It will not be cascaded to the reverse end of associations.
|
---|
Database Round-Trips
| It might cause the more database inter-relations.
| It might cause the lesser database inter-relations.
|
---|
Efficiency and Performance
| It might pursue to more structured use of IDs.
| It might pursue to more structured use of IDs but only in some scenes.
|
---|
16. What is the difference between the get and load method?
Properties | get() | load() |
---|
Return Type | It will give the real object. | It will give the substitute object. |
---|
Database Access | It will continuously strike the database. | It might not continuously strike the database. |
---|
Exception on Missing Object | It will give you ‘null’ if it’s not available. | It will give you ‘ObjectNotFoundException’ if it’s not available. |
---|
Proxy Initialization | It will instantly prepare the proxy. | It will slowly prepare the proxy. |
---|
Eager Fetching | It will keep up eager collecting. | It will not have eager collecting. |
---|
Unlimited State | It will give an uninitialized proxy. | It will also give a give uninitialized proxy. |
---|
Lazy Initialization Exception | It might be given if associations have not been collected. | It might give if associations have accessed outside sessions. |
---|
Use Cases | It will be used for recovering the objects when a particular existence is not known. | It will be used for slow and structured loading. |
---|
17. What is the difference between update and merge method?
Properties | update() | merge() |
---|
Managed Object
| It should be a continuing object.
| It should be a detached or transient object.
|
---|
Return Type
| It gives a void object.
| It gives a managed object.
|
---|
Overwrite Detached
| It will reinitialize the detached object.
| It will replicate the states to structured objects.
|
---|
Unsaved Transient
| It will throw an exception for the transient.
| It will carry on the transient object.
|
---|
Copying State
| It will replicate all the states covering nulls.
| It will only replicate the null states.
|
---|
Cascade Operations
| It will be cascaded to connected entities.
| It will be cascaded through required entity mapping.
|
---|
Stale Data Handling
| It will be upgraded to its current state.
| It will control the old data conflict.
|
---|
Versioned Entities
| It will need the version property.
| It will control the version property.
|
---|
Identity Strategy
| It will only favor and encourage assigned IDs.
| It will favor and encourage assigning all the assigned IDs.
|
---|
Performance Impact
| It might create more SQL updates.
| It might give in only some SQL updates.
|
---|
18. What is the difference between first-level cache and second-level cache?
Properties | First-Level Cache | Second-Level Cache |
---|
Scope
| It will be restricted to the Hibernate Session.
| It will be divided into processes and sessions.
|
---|
Granularity
| It will give entity-level results.
| It will give query and entity-level results.
|
---|
Storage
| It will reside in memory.
| It will be configurable as memory, disk, etc.
|
---|
Concurrency
| It’s not suggested for multi-session concurrency.
| It’s mostly used for multi-session concurrency.
|
---|
Lifetime
| It will only stay for the session’s timings.
| It could have configurable lifetimes.
|
---|
Types of Objects Cached
| It has Structured(persistent) objects cache.
| It has queries, collections, structured objects, etc.
|
---|
Cache Strategies
| It has the in-built (not user-configurable) strategies.
| It has the user-defined strategies.
|
---|
Customization
| It will provide restricted customize options.
| It will provide you with more regulated and customized options.
|
---|
Cache Providers
| It has only a single for each session factory.
| It might have many session factory providers.
|
---|
Use Cases
| It will avail you of the performance enhancement inside the session.
| It will avail you the performance enhancement over processes and sessions.
|
---|
19. Can you tell the difference between getCurrentSession and openSession methods?
Properties | getCurrentSession() | openSession() |
---|
Life Management
| It will be handled by Hibernate and bind to the transaction.
| It will be handled by the application and have to be manually structured.
|
---|
Scope
| It is only restricted to current transactions.
| It is not restricted to any particular transaction.
|
---|
Automatic Closure
| It will automatically terminate at the end of the transaction.
| It should be closed by hand.
|
---|
Exception Handling
| Here hibernate controls the exception propagation.
| Here application should control the exceptions.
|
---|
Configuration
| It will have configuration-handle behavior.
| Its explicit nature depends on how it is used.
|
---|
Reusability
| It will be used again and again only in the same transactions.
| It will be used in different transactions also.
|
---|
Contextual Awareness
| It must be known in the present transaction context.
| It is not known to any of the transaction context.
|
---|
Use Cases
| It is mostly suitable for short-lived operations.
| It is mostly suitable for custom scenes or long-lived operations.
|
---|
20. Differentiate between save () and saveOrUpdate() methods in the hibernate session.
Properties | session.save() | session.saveOrUpdate() |
---|
New Entity
| It will be available for only transient entities.
| It will be available for detached as well as transient entities.
|
---|
Persistent Entity
| It will throw the exception if the entity presents in DB.
| It will modify the entity if available in DB apart from saving it.
|
---|
Entity State
| It will be the same as transient after being saved.
| It will be the same as persistent after being saved.
|
---|
Cascade Operations
| It must be cascaded to linked entities.
| It must be cascaded to entity mapping accordingly.
|
---|
Identifier Strategy
| It should always use ID creation for auto-increment.
| It supports multiple ID identifier tactics.
|
---|
Managed State
| It didn’t influence the structured state.
| It can reconnect to the detached entity.
|
---|
Unsaved Transient State
| It perseveres the transient entity.
| It perseveres the transient entity.
|
---|
Auto-generated ID
| It always needed ID creation tactics.
| The ID creation tactics may be used here.
|
---|
Use Cases
| It will generate the new entities.
| It will generate new or modify available entities.
|
---|
21. What are the states of the object in Hibernate?
In Hibernate, an object can be classified as one of the following states as follows:
I. Transient State
Whenever an object is created by using a new keyword and not connected with any of the Hibernate Session, it is called a Transient State. The object is not yet preserved in the database as hibernate is also unknown.
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
II. Persistent State
The Transient objects will be Persistent when they are linked with the Hibernate Session using the save() or persist() method. At this stage, the object is controlled by hibernate and when the transaction is committed with the database when any changes happen it will be tracked and synchronised.
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
// Persistent state
Session session = sessionFactory.openSession();
session.beginTransaction();
session.persist(employee); // Now the object is persistent
session.getTransaction().commit();
session.close();
III. Detached State
When an object was once linked with the hibernate session that becomes detached and no longer in the current session’s scope. This happens when a transaction is committed/rolled back, object is explicitly removed, or session is ejected.
Session session = sessionFactory.openSession();
session.beginTransaction();
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
session.persist(employee);
session.getTransaction().commit();
session.close(); // Now the object is detached
// Perform some changes on the detached object
employee.setAge(31);
IV. Removed/Deleted State
When an object comes in a state where it is in the removed/deleted state as once it was persistent but expelled explicitly from the database using ‘remove()’ method or ‘delete()’ method in the hibernate session. The object can’t be obtained without re-linking it to the new session and no longer associated with the database.
Session session = sessionFactory.openSession();
session.beginTransaction();
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
session.persist(employee);
session.getTransaction().commit();
// Removed state
session.beginTransaction();
session.delete(employee); // Now the object is removed
session.getTransaction().commit();
session.close();
NOTE: Developers must take care of these states precisely to avoid these issues like unnecessary database operations or detached object modifications
22. How to make an immutable class in Hibernate?
In a concurrent environment, immutable classes are generally used to show read-only data which are more predictable and thread-safe.
Some steps to create an immutable class in Hibernate as:
- Using final keyword: cannot be made further sub-class and cannot override its behavior.
- Make fields private and final: Declare your class-fields as private and final, and initialise them only through the help of the constructor. This prevents any direct modification of the fields after the object is constructed.
- No setters: As the fields are final, any setter methods to modify the field values cannot be performed and they can only be initialized during the object creation.
- Ensure immutability of referenced objects: If your code class contains references to mutable objects, make sure that references either make the referenced objects immutable or deep-copied while construction as well.
Immutable class using Hibernate:
Java
public final class ImmutableEmployee {
private final String name;
private final int age;
// Private constructor for internal use only
private ImmutableEmployee(String name, int age){
this.name = name;
this.age = age;
}
// Factory method to create instances of
// ImmutableEmployee
public static ImmutableEmployee create(String name,
int age){
return new ImmutableEmployee(name, age);
}
// Getters for fields (no setters)
public String getName() { return name; }
public int getAge() { return age; }
}
To create an ImmutableEmployee object, you can use the create factory method, which will return the class instance as:
ImmutableEmployee employee = ImmutableEmployee.create("John Doe", 30);
23. What is automatic dirty checking in Hibernate?
Automatic dirty checking, also referred to as Automatic dirty tracking, detects the changes that occurred without the call to the persistent objects to update them in the database.
Hibernate automatically traces all the changes made to its properties when any object is connected with the Hibernate Session, and during transaction commit, these modifications totally co-exist with the database which ensures that the database remains compatible with the state of the object.
Methods of automatic dirty checking done in the Hibernate:
- Persistent Object Association: Hibernate keeps track and can automatically find the changes made in its properties as when the object is fetched or loaded from the database it becomes linked with the present Hibernate Session
- Tracking Property Modifications: To detect the changes made hibernate compares modified values with the original snapshot whenever a change in the properties of the object is detected.
- Detecting Dirty State: Hibernate marks a property as dirty or modified property if the property’s value differs from the original value.
- Synchronization with the Database: By ensuring the changes are reflected in the database, hibernate automatically makes and runs SQL update statements for dirty properties during transaction commit.
Now for every alter object an explicit call for update() or saveOrUpdate() is not required as Automatic dirty checking helps to ease the Hibernate update process.
Employee class mapped to a database table:
Java
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private int age;
// Constructors, getters, setters, etc.
}
Now, let’s use this Employee class in a Hibernate transaction:
Session session = sessionFactory.openSession();
session.beginTransaction();
// Load an employee object from the database
Employee employee = session.get(Employee.class, 1L);
// Modify the employee object
employee.setAge(31);
// The automatic dirty checking detects the change in the
// age property and synchronizes the update with the
// database during the transaction commit.
session.getTransaction().commit();
session.close();
24. Is Hibernate prone to SQL injection attacks?
No, when hibernate is used properly it will not be prone to SQL injection attacks. By using proper query construction and parameter binding we get inherent protection from SQL injection attacks, Hibernate’s query mechanisms like HQL and Criteria API work here.
In a schema of risk, an app may build SQL queries using input straight from the users without allowing attackers and proper sanitization to inject malicious SQL code. Hibernate doesn’t set values directly into the SQL statement, it first binds the parameter to pass values into queries.
Thus, preventing the attackers from injecting malicious code, user-given inputs are not executed as SQL code but moreover treated as data values.
Example:
Taking insight into the last risk schema with our login page, this time using Hibernate’s HQL:
String username = request.getParameter("username");
String password = request.getParameter("password");
Query query = session.createQuery(
"FROM User WHERE username = :username AND password = :password");
query.setParameter("username", username);
query.setParameter("password", password);
List<User> users = query.list();
So, in this modified example, the attacker gives suspicious inputs like ‘admin’ OR ‘1’=’1′, In hibernate it will not take it as SQL code but instead considers it as a data value. Hence, the attacker will be unable to control the query’s behavior and it will remain secure.
25. What are the most commonly used annotations available to support hibernate mapping?
To interact with the database hibernates provided a bunch of annotations that you can use for mapping Java classes to database tables. Some common annotations in hibernate for mapping as:
I. @Entity:
It represents the database in the table and points to a Java class as an entity.
@Entity
@Table(name = "employees")
public class Employee {
}
II. @Table:
It explains the details of the table related to the entity.
@Entity
@Table(name = "employees")
public class Employee {
}
III. @Id:
@Id denotes the primary key of the entity.
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
}
IV. @GeneratedValue:
It denotes how the primary key is made.
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
V. @Column:
In the database table it plots a field to the column.
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "employee_name")
private String name;
}
VI. @OneToMany and @ManyToOne:
It settled a many-to-one or one-to-many relationship between entities.
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
}
VII. @ManyToMany:
Defines a many-to-many relationship between entities. It explains the many-to-many relationship between the entities.
@Entity
@Table(name = "courses")
public class Course {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToMany(mappedBy = "courses")
private List<Student> students;
// ...
}
@Entity
@Table(name = "students")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToMany
@JoinTable(name = "student_courses",
joinColumns =
@JoinColumn(name = "student_id"),
inverseJoinColumns =
@JoinColumn(name = "course_id"))
private List<Course> courses;
}
26. Explain Hibernate architecture.
Hibernate provides a clear architectural structure and proper segmentation of concerns in the application and is headed by a layer of architecture that has different components responsible for many tasks like
- querying the database,
- ORM (Object-Relational Mapping),
- Transactional management.
The main components of hibernate architecture as:
I. Application Layer
It is the upper-most layer where application belongs. It is linked with the Hibernate API to perform many tasks such as updating, deleting, saving and querying objects from the database.
II. Hibernate API
The Hibernate API interacts with the persistence layer by using a set of classes and interfaces of the application. Some key interfaces like ‘Session’, ‘Transaction’ and “SessionFactory’.
III. Configuration
This component can be specified through Java configuration classes or XML files and accountable for providing necessary properties and configuring hibernate for the dialects, database connections and other settings.
Configuration configuration = new Configuration();
configuration.configure("hibernate.cfg.xml");
SessionFactory sessionFactory = configuration.buildSessionFactory();
IV. Session Factory:
It is a weighted object which is created only one instance per application and also responsible for governing hibernate sessions and creating instances.
SessionFactory sessionFactory = new Configuration()
.configure("hibernate.cfg.xml")
.buildSessionFactory();
v. Session:
It is a small lifespan object that shows a conversation between database and the application and is also responsible for directing the lifecycle of objects, providing transaction boundaries and performing CRUD operations.
Session session = sessionFactory.openSession();
Employee employee = session.get(Employee.class, 1L);
session.saveOrUpdate(employee);
session.close();
vi. Transaction:
This interface provides methods to commit, rollback and begin the transactions and also used to handle the transactions in hibernate.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
try {
transaction.commit();
}
catch (Exception e) {
transaction.rollback();
}
finally {
session.close();
}
vii. Mapping Metadata:
It instructs the hibernate how to change the java objects into database records and vice versa. It is also noted through XML files or annotations and responsible for the mapping between database tables and Java classes.
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
}
viii. Object-Relational Mapping (ORM):
It is the core or main functionality in the hibernate which guarantees that the data is correctly updated, stored and retrieved in the database while underlying SQL operations and also responsible for changing database records to Java objects and vice versa.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Employee employee = new Employee();
employee.setName("John Doe");
employee.setAge(30);
session.save(employee);
transaction.commit();
session.close();
27. What is the criteria API in Hibernate?
In hibernate it is a type-safe and programmatic way to make a database using Java code instead of native SQL queries or writing raw HQL (Hibernate Query Language) and helpful for making queries with dynamic sorting, projections and difficult conditions.
It also provides a smooth object-oriented approach to develop queries dynamically at runtime. Some advantages of using it includes enhanced readability, minimising risk of syntax error in queries and compile-time type checking. An explanation and example to how to use this in hibernate.
Example Scenario:
List of employees with specific salary and age greater than a particular value.
Using HQL:
String hql = "FROM Employee WHERE age = :ageParam AND salary > :salaryParam";
Query query = session.createQuery(hql);
query.setParameter("ageParam", 30);
query.setParameter("salaryParam", 50000);
List<Employee> employees = query.list();
Using Criteria API:
CriteriaBuilder criteriaBuilder = session.getCriteriaBuilder();
CriteriaQuery<Employee> criteriaQuery = criteriaBuilder.createQuery(Employee.class);
Root<Employee> root = criteriaQuery.from(Employee.class);
Predicate agePredicate = criteriaBuilder.equal(root.get("age"), 30);
Predicate salaryPredicate = criteriaBuilder.greaterThan(root.get("salary"), 50000);
criteriaQuery.where(criteriaBuilder.and(agePredicate,salaryPredicate));
List<Employee> employees = session.createQuery(criteriaQuery).getResultList();
- CriteriaBuilder: This works as a starting point for our API and provides methods to make various query elements like sorting, predicates and expressions.
- CriteriaQuery: It defines the result type and works as the main query (in this case, ‘Employee’).
- criteriaBuilder.equal: It made equality predicate for age attribute.
- criteriaBuilder.greaterThan: It creates a predicate for salary attribute if it is larger than a given limit of value.
28. What does session.lock() method in Hibernate do?
In hibernate, locking mechanism helps in controlling concurrent authority to the database records, preventing conflicts between transactions and ensuring data consistency. The Session.lock() is used for explicitly taking the pessimistic lock over the persistent object.
- During multiple transactions, there is a possibility for conflict to raise as they are working with the same data side-by-side.
- For example, if two transactions in the database try to update the same records side-by-side which can cause inconsistency.
- So, we use Locking which allows you to undergo the database records by preventing modification in other transactions until lock is released.
Hibernate provided us two types of locking: optimistic locking and pessimistic locking. Though session.lock() is used for acquiring the pessimistic lock, which means until the lock will not be released the transaction explicitly acquires a lock on the record by preventing other transactions from being modified.
29. What is Hibernate caching?
It is a technique which reduces repeatedness of fetching data from the database which clean application response timing, reduces load on database server and improves performance of the database communication by saving frequent data in memory.
Hibernate provides many levels of caching where each serves different benefits and specific purposes. It could efficiently improve application performance, whenever complex queries are involved or the same data access again and again.
Types of Hibernate Caching:
I. First-Level(Session) Cache:
- It is enabled by default and cache mapped with Hibernate Session.
- It stores objects of the current session which are recovered.
- It also provides data consistency at transaction level.
II. Second-Level(Session Factory) Cache:
- In the same Sessionfactory, the cache is distributed among all the sessions created.
- It caches data used in different sessions.
- It also provides data consistency at application level.
Hibernate Interview Questions For Experienced
30. When is merge() method of the hibernate session useful?
It is mainly used when you want to save the changes back from your previously detached(outside scope of the Hibernate session) in the database. It generally used to update a detached object back to the persistence context(managed state).
In Hibernate, an object could be in one of these three states:
- transient
- persistent
- detached
So, if you want to merge the detached object you can use the merge() method to reattach it in a new session and compile it with changes to reflect it into the database.
Example:
To update existing Employee object which has been detached:-
// Detached object
Employee detachedEmployee = new Employee();
detachedEmployee.setId(1L);
detachedEmployee.setName("John Doe");
// You want to update the employee's name
detachedEmployee.setName("Jane Smith");
Session session = sessionFactory.openSession();
session.beginTransaction();
// Using merge to update the detachedEmployee
Employee updatedEmployee
= (Employee)session.merge(detachedEmployee);
session.getTransaction().commit();
session.close();
31. Does Hibernate support Native SQL Queries?
Yes, Hibernate supports Native SQL Queries which allows us to run SQL statements right against the database and we can use the createSQLQuery() method to use it in hibernate.
createSQLQuery(): It runs and recovers the results which returns a query object that is customised with parameters.
List of employee names and their salaries using custom SQL queries from database-
Session session = sessionFactory.openSession();
session.beginTransaction();
String sql = "SELECT name, salary FROM employees";
SQLQuery query = session.createSQLQuery(sql);
List<Object[]> results = query.list();
for (Object[] result : results) {
String name = (String)result[0];
BigDecimal salary = (BigDecimal)result[1];
System.out.println("Name: " + name
+ ", Salary: " + salary);
}
session.getTransaction().commit();
session.close();
32. What happens when the no-args constructor is absent in the Entity bean?
If no-args constructor is absent, instantiation of objects by hibernate can’t happen properly which leads to errors. No-args constructor in hibernate is an entity bean which is very essential as in instantiation of objects in hibernate done by its reflection of constructing java objects and reading data from the database.
Example without a no-args constructor:
Java
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
// No no-args constructor
public Employee(String name) {
this.name = name;
}
}
In this example, when hibernate tries to instantiate Employee objects using reflection during data retrieval it will get an error as we can’t create objects without a no-args constructor.
Solution: Always add no-args constructor in your entity classes to get proper functioning with hibernate:
Java
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name") private String name;
// No-args constructor
public Employee() {}
public Employee(String name) { this.name = name; }
}
By including no-args constructor, ‘Employee’ objects successfully get instantiated in hibernate when it requires reading data through the database.
33. Can we declare the Entity class final?
Yes, you can declare an entity or class as ‘final’ in hibernate but it has some complications as it can’t be subclassed which affects hibernate’s ability to perform tasks like runtime enhancements and proxy generation for lazy loading for certain features.
Entity class with ‘final’ modifier:
Java
@Entity
@Table(name = "employees")
public final class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name") private String name;
}
34. Explain Query Cache?
The Query Cache in Hibernate is a mechanism designed to cache the results of database queries. It helps to optimise query performance by storing the query results in memory, reducing the need to repeatedly execute the same query against the database. When a cached query is executed again with the same parameters, hibernate can quickly retrieve the results from the cache, enhancing application responsiveness and reducing database load.
To executes query caching you should to do:
- By enabling second-level cache for session factory configurations.
- By enabling caching for a particular query using setCacheable(true)
35. How to solve the N+1 SELECT problem in Hibernate?
N+1 SELECT problem occurs in hibernate when calling the collections of entities takes a very large number of single SQL SELECT statements being executed and this majorly impacts performance as it runs the high number of database round-trips.
To solve this problem, we can use batch fetching and fetch joins techniques as: –
I. Batch Fetching
It can be used globally in hibernate configuration or at mapping level by using @BatchSize. It also fetches entities in batches by reducing the number of SELECT statements.
Java
@Entity
@Table(name = "departments")
@BatchSize(size = 10) // Batch fetching for employees
public class Department {
}
II. Fetch Join
It allows to get both main and its related entities in a single result set. In HQL query by using JOIN FETCH syntax you can recover related entities in a single query.
String hql = "SELECT d FROM Department d JOIN FETCH d.employees WHERE d.id = :deptId";
Query query = session.createQuery(hql);
query.setParameter("deptId", 1L);
Department department = (Department)query.uniqueResult();
36. What is a Single Table Strategy?
In hibernate it’s one of the inheritance mapping strategies and mapped onto a single database table. Here all the attributes of the whole hierarchy stored in a single table and sidley discriminator column is used to separate between different subclasses.
Example:
Let’s say we have an inheritance hierarchy including base class Vehicle’ with two subclasses ‘Car’ and ‘Motorcycle’. By using Single Table Strategy all attributes of ‘Vehicle’, ‘Car’ and ‘Motorcycle’ feeded in a single database table also with a discriminator column to show the subclass type. To show the Single Table Strategy by using marked inheritance hierarchy:
// Entity 1
@Entity
@DiscriminatorValue(“car”)
public class Car extends Vehicle {
private int numberOfDoors;
}
// Entity 2
@Entity
@DiscriminatorValue(“motorcycle”)
public class Motorcycle extends Vehicle {
private boolean hasSideCar;
}
37. What are the benefits of NamedQuery?
Named queries provide many benefits that support more maintainability, efficiency, and cleanliness of code. Some main advantages of using Named queries as:
- Separation of Concerns: It helps in increasing the code maintainability and readability by using SQL and HQL queries in the Java code and encourages clearer differences between application logic and data access.
- Code Reusability: It helps in reducing redundancy by reusing it across distinct parts of the application and makes it simple to apply changes to queries continuously by enhancing consistency.
- Performance Improvements: It helps in the repeated execution of queries by compiling at startup and by supplying needed performance improvements over ad-hoc queries each time.
- Maintainability: It helps when optimization efforts or schema changes need query adjustments. It can be managed centrally by making it simple to modify or update queries over your application.
Hibernate Bonus Question 2024
Q. What is the purpose of the @DynamicUpdate annotation in Hibernate, and how does it affect entity updates?
The @DynamicUpdate annotation is used in Hibernate to optimize for entities to update the statements. By default, when an entity is updated, Hibernate generates an update statement that includes all columns, even if only a few columns have actually changed. This can result in unnecessary database traffic and decreased performance.
The @DynamicUpdate annotation addresses this issue by instructing Hibernate to generate an update statement that includes only the columns that have been modified in the current transaction. This optimization can significantly reduce the amount of data being sent to the database during updates, leading to improved performance and reduced network overhead.
The @DynamicUpdate annotation resolves this concern by directing Hibernate to create an update statement containing solely the columns that have undergone modification within the current transaction. This enhancement substantially minimizes the volume of data transmitted to the database during updates, thereby enhancing performance and diminishing network overhead. Below are the key points about the @DynamicUpdate annotation in Hibernate:
- Optimized Updates
- Selective Common Update
- Reduction in Data Transfer
- Performance Boost
- Network Overhead Reduction
Conclusion
In Summary, Hibernate is like a magic wand that traverses between your Java code and the database making your coding smoother and enjoyable. It bridges the gap between object-oriented programming languages, such as Java, and relational databases, like
At last, There is a high demand for Java developers who are skilled in Hibernate. Preparing for these questions can boost your chances of success in your next Hibernate interview.
Most Asked Hibernate Interview Questions
1. What is Hibernate?
Hibernate is an open source Object-Relational Mapping (ORM) framework for Java. It provides a way to map Java objects to database tables and vice versa. This allows developers to write code that is more object-oriented and less database-specific.
2. What are the advantages of using Hibernate?
Hibernate offers a number of advantages over JDBC, the traditional way to interact with databases from Java. These advantages include:
- Simplified code
- Object-oriented programming
- Immutability
- Transactions
- Caching
3. What are the disadvantages of using Hibernate?
Hibernate also has some disadvantages, such as:
- Learning curve: Hibernate is a complex framework, so it takes some time to learn how to use it effectively.
- Performance: Hibernate can add some overhead to database access, so it is important to use it carefully to avoid performance problems.
- Vendor lock-in: Hibernate is an open source project, but it is developed and maintained by a single vendor. This can make it difficult to switch to a different ORM framework if necessary.
4. What are some of the important interfaces of Hibernate framework?
Some of the important interfaces of Hibernate framework are:
- SessionFactory: This is the factory class that is used to create Session objects.
- Session: This is the main object used to interact with the database.
- Transaction: This is used to group a series of database operations together.
- Criteria: This is used to create dynamic queries.
- Query: This is used to execute a predefined query.
- HQL: This is a high-level query language that is used to query the database.
5. What is lazy loading in Hibernate?
Lazy loading is a technique that is used to defer the loading of an object’s child objects until they are actually needed. This can improve performance by reducing the number of database queries that need to be executed.
Lazy loading is enabled by default in Hibernate. However, it can be disabled for specific objects or relationships.
6. What is the difference between first level cache and second level cache?
First level cache is a cache that is associated with a Session object. It is used to store objects that have been loaded by the Session.
Second level cache is a shared cache that is used to store objects that have been loaded by multiple Session objects. It can be used to improve performance by reducing the number of database queries that need to be executed.
7. What are some of the best practices for using Hibernate?
Some of the best practices for using Hibernate include:
- Use immutable objects: Immutable objects are objects that cannot be modified after they are created. This can help to improve performance and prevent data corruption.
- Use lazy loading: Lazy loading can improve performance by deferring the loading of an object’s child objects until they are actually needed.
- Use caching: Caching can improve performance by storing frequently accessed objects in memory.
- Use transactions: Transactions can help to ensure the ACID properties of database operations.
- Use logging: Logging can help to troubleshoot problems with Hibernate applications.
8. How is SQL query created in Hibernate?
In Hibernate, SQL queries are generated automatically based on HQL (Hibernate Query Language), these are generated from high-level queries and are autonomously produced or through the criteria API. The Hibernate translator takes these high-level queries and converts them into the actual SQL queries so that the database could understand. This operation is referred to as the query translation. This process gives developers the ability to engage in the database interaction through queries resembling Java that constructs while the underlying translation to SQL is executed seamlessly.
Share your thoughts in the comments
Please Login to comment...