Amazon SDE Sheet: Interview Questions and Answers
Last Updated :
09 Feb, 2024
Amazon SDE sheet is the collection of the most important topics or the most frequently asked question in Amazon Software Development Engineer Interviews. Here we have collected all the interview questions and answers to land a job on Amazon
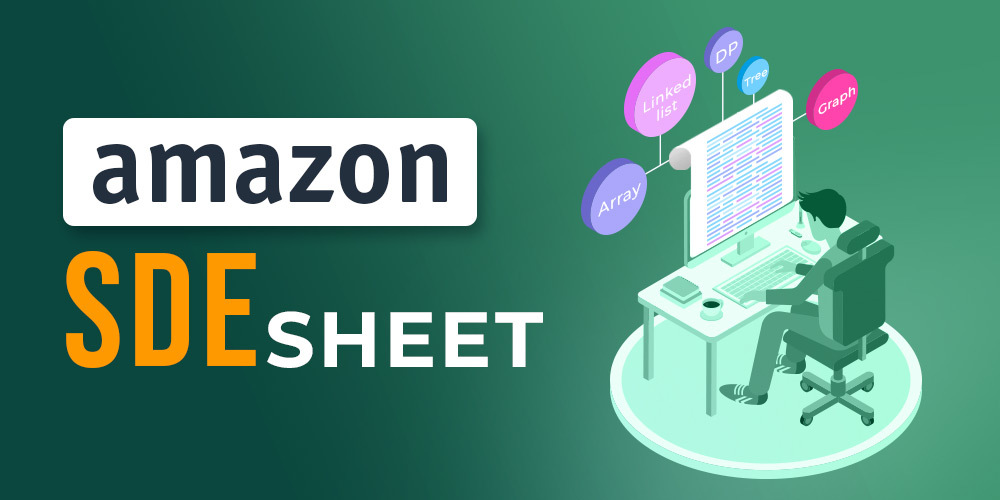
Amazon SDE Sheet
Amazon is an American multinational technology company that focuses on e-commerce, cloud computing, digital streaming, and artificial intelligence. To get employed by amazon is a dream for many. One must have clear Data Structure concepts, good communication skills, and analytical thinking and should be able to solve real-world problems to crack top companies like Amazon. Steps to follow to get a chance at amazon are:
- Online Application
- Online test: (60 mins) 2 coding questions of medium difficulty level. The Amazon online test (20 questions) is based on OOPs, DBMS, operating systems, data structures, and algorithms.
- Technical Interview 1: Project discussions, coding questions based on DS algorithms, and quizzes. It basically tests real-world problem-solving skills.
- Technical Interview 2: Questions of relatively higher difficulty level (mostly DS and algorithms based)
- Bar raiser round: Behavioral questions and project discussions. Some technical questions are followed by an assessment of leadership quality and cultural fit.
Roadmap to Amazon SDE
For one to land a job at Amazon, he/she must have clear concepts of DSA and good practice of questions on sorting, array, string, Linked List, searching, sorting, stack, queue, tree, graph recursion backtracking dynamic programming, etc. Here through this Amazon SDE sheet, we are providing most of the coding questions which frequently pop up in amazon interviews topic-wise. This collection of interview questions will help you prepare better for your interview.
Computer Fundamentals: There are three focused computer subjects, on the basis of those subjects MCQs will be there, we have designed top MCQs for that, so you can easily test your knowledge on those subjects.
Language Based Questions: They can be asked language-based questions, to check your grasp of the language you used for the coding round.
Data Structure & Algorithms
Array: An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together.
String: Strings are defined as an array of characters. The difference between a character array and a string is the string is terminated with a special character ‘\0’.
Linked List: Linked List is the data structure that can overcome all the limitations of an array. A Linked list is a linear data structure, in which the elements are not stored at contiguous memory locations, it allocates memory dynamically.
Searching: Searching Algorithms are designed to check for an element or retrieve an element from any data structure where it is stored.
Sorting: A Sorting Algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. The comparison operator is used to decide the new order of elements in the respective data structure.
Stack: A stack is a linear data structure in which elements can be inserted and deleted only from one side of the list, called the top. A stack follows the LIFO (Last In First Out) principle.
Queue: A queue is a linear data structure in which elements can be inserted only from one side of the list called rear, and the elements can be deleted only from the other side called the front. The queue data structure follows the FIFO (First In First Out) principle.
Tree: A tree is non-linear and a hierarchical data structure consisting of a collection of nodes such that each node of the tree stores a value, a list of references to nodes (the “children”).
Graph: A Graph is a non-linear data structure consisting of nodes and edges. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph.
Trie: Trie is an efficient information retrieval data structure. Using Trie, search complexities can be brought to optimal limit (key length).
Heap and Hash: A Heap is a special Tree-based data structure in which the tree is a complete binary tree. Heap and hash is an efficient implementation of a priority queue. The linear hash function monotonically maps keys to buckets, and each bucket is a heap.
BitMagic: Bit stands for binary digit. A bit is the basic unit of information and can only have one of two possible values that is 0 or 1.
Recursion and Backtracking:
- Recursion: The process in which a function calls itself directly or indirectly is called recursion and the corresponding function is called a recursive function.
- Backtracking: Backtracking is an algorithmic technique for solving problems recursively by trying to build a solution incrementally, one piece at a time, removing those solutions that fail to satisfy the constraints of the problem at any point in time (by time, here, is referred to the time elapsed till reaching any level of the search tree).
Dynamic Programming: Dynamic Programming is mainly an optimization over plain recursion. Wherever we see a recursive solution that has repeated calls for same inputs, we can optimize it using Dynamic Programming.
After qualifying the Online test you have to face 2 technical interviews, where they asked about Data Structure, algorithms, and different kinds of puzzles. Technical rounds are face-to-face algorithmic rounds in which candidates are presented with 2-4 questions, all from data structures. The most commonly asked DSs are the matrix, binary tree, BST, and Linked list. The second technical round is more difficult and more questions from Trees, BST, and Graph are asked. One should have a clear knowledge of tree-based recursion, and the standard questions based on it are a must.
P.S: After solving all the problems mentioned above you can answer the questions which will be asked in these rounds.
Puzzles
Puzzles are one of the ways to check your problem-solving skills. These are tricky questions that let you think logically. Amazon will test your problem-solving skills through the puzzles as well. Try to solve these 20 Puzzles Commonly Asked During SDE Interviews
Project Discussion
Thoroughly revise all the work you have done till now in your projects. The grilling about projects can sometimes be very deep. Mention only those topics where you think you are fine to be grilled upon. If you don’t have any project they will not ask about it, but better to have some projects, it involves questions like what’s new in your project if you have created a basic clone, or what’s your input followed by questions based on your technology stack.
If you haven’t made a project then take an idea from GFG Projects and start working on it.
Note: If you have a project on AWS then you have to be confident enough to provide sufficient answers of each question,
To let you know in details about Amazon Recruitment Process, we have a article on that too so you can go through this post:
https://www.geeksforgeeks.org/amazon-recruitment-process/
Share your thoughts in the comments
Please Login to comment...