Delete a Node from linked list without head pointer
Last Updated :
16 Feb, 2023
You are given a singly linked list and pointer which is pointing to the node which is required to be deleted. Any information about the head pointer or any other node is not given. You need to write a function to delete that node from the linked list. Your function will take only one argument, i.e., a pointer to the node which is to be delete
Note: No head reference is given to you. It is guaranteed that the node to be deleted is not the last node:
A linked list is built as:
Definition of each node is as follows:
struct Node {
int data;
struct Node* next;
};
Examples:
Consider below LL for given examples:
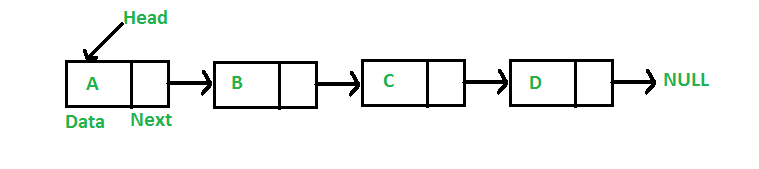
Input: C (a pointer to C)
Output: A–>B–>D–>E–>F
Input: A (a pointer to A)
Output: B–>D–>E–>F
Approach: To solve the problem follow the below idea:
- Why conventional deletion method would fail here?
It would be a simple deletion problem from the singly linked list if the head pointer was given because for deletion you must know the previous node and you can easily reach there by traversing from the head pointer. Conventional deletion is impossible without knowledge of the previous node of a node that needs to be deleted.
- How to delete the node when you dont have the head pointer?
The trick here is we can copy the data of the next node to the data field of the current node to be deleted. Then we can move one step forward. Now our next has become the current node and the current has become the previous node. Now we can easily delete the current node by conventional deletion methods.
Illustration of the above approach:
For example, suppose we need to delete B and a pointer to B is given
If we had a pointer to A, we could have deleted B easily.
But here we will copy the data field of C to the data field of B. Then we will move forward.
Now we are at C and we have a pointer to B i.e. the previous pointer. So we will delete C.
That’s how node B will be deleted.
Below image is a dry run of the above approach:
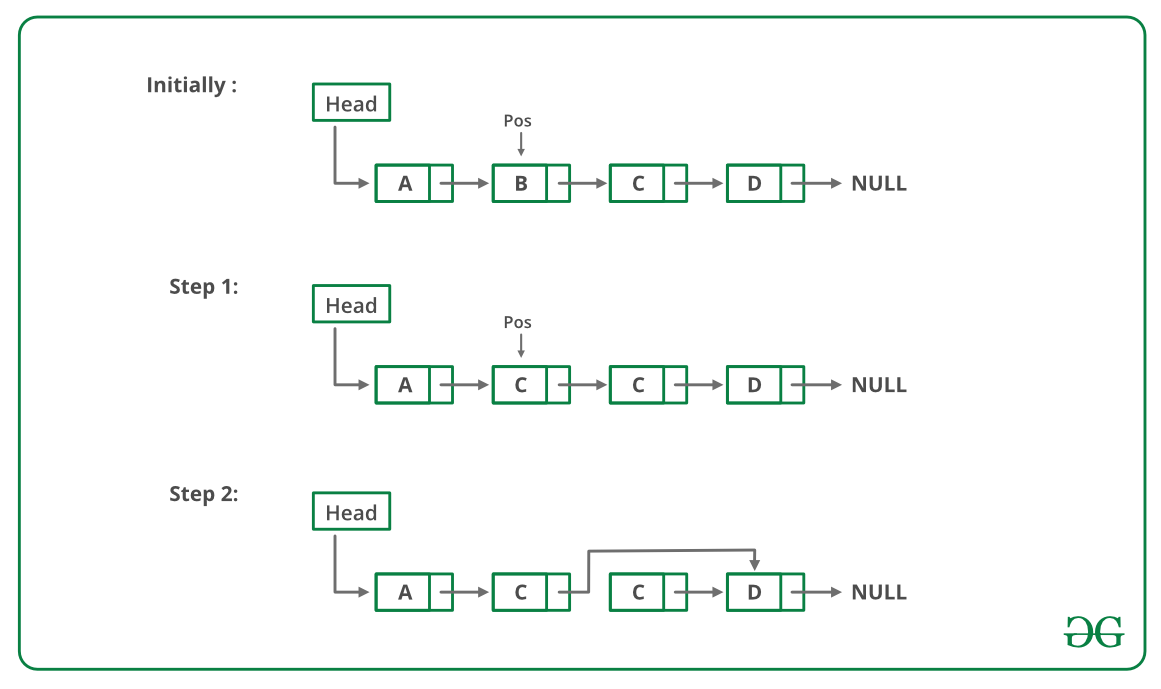
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
struct Node* next;
};
void deleteNodeWithoutHead( struct Node* pos)
{
if (pos == NULL)
return ;
else {
if (pos->next == NULL) {
printf ( "This is last node, require head, can't "
"be freed\n" );
return ;
}
struct Node* temp = pos->next;
pos->data = pos->next->data;
pos->next = pos->next->next;
free (temp);
}
}
void print(Node* head)
{
Node* temp = head;
while (temp) {
cout << temp->data << " -> " ;
temp = temp->next;
}
cout << "NULL" ;
}
void push( struct Node** head_ref, int new_data)
{
struct Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
int main()
{
struct Node* head = NULL;
push(&head, 20);
push(&head, 4);
push(&head, 15);
push(&head, 35);
cout << "Initial Linked List: \n" ;
print(head);
cout << endl << endl;
Node* del = head->next;
deleteNodeWithoutHead(del);
cout << "Final Linked List after deletion of 15:\n" ;
print(head);
return 0;
}
|
Java
class Node{
int data;
Node next;
Node( int val){
data = val;
next = null ;
}
}
class LinkedList{
Node head;
void deleteNodeWithoutHead(Node pos){
if (pos == null )
return ;
else {
if (pos.next == null ){
System.out.println( "This is last node, require head, can't be freed\n" );
return ;
}
}
pos.data = pos.next.data;
pos.next = pos.next.next;
}
public void push( int new_data){
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
public void printList(){
Node temp = head;
while (temp != null ){
System.out.print(temp.data + "->" );
temp = temp.next;
}
System.out.println( "NULL\n" );
}
public static void main(String args[]){
LinkedList llist = new LinkedList();
llist.push( 20 );
llist.push( 4 );
llist.push( 15 );
llist.push( 35 );
System.out.println( "Initial Linked List : " );
llist.printList();
Node del = llist.head.next;
llist.deleteNodeWithoutHead(del);
System.out.println( "Final Linked List after deletion of 15 : " );
llist.printList();
}
}
|
Python3
class Node:
def __init__( self , val):
self .data = val
self . next = None
class LinkedList:
def __init__( self ):
self .head = None
def delete_node_without_head( self , pos):
if pos is None :
return
elif pos. next is None :
print ( "This is last node, require head, can't be freed\n" )
return
pos.data = pos. next .data
pos. next = pos. next . next
def push( self , new_data):
new_node = Node(new_data)
new_node. next = self .head
self .head = new_node
def print_list( self ):
temp = self .head
while temp is not None :
print (temp.data, end = '->' )
temp = temp. next
print ( "NULL\n" )
if __name__ = = '__main__' :
llist = LinkedList()
llist.push( 20 )
llist.push( 4 )
llist.push( 15 )
llist.push( 35 )
print ( "Initial Linked List : " )
llist.print_list()
del_node = llist.head. next
llist.delete_node_without_head(del_node)
print ( "Final Linked List after deletion of 15 : " )
llist.print_list()
|
C#
using System;
class Node
{
public int data;
public Node next;
public Node( int val)
{
data = val;
next = null ;
}
}
class LinkedList
{
public Node head;
public LinkedList()
{
head = null ;
}
public void delete_node_without_head(Node pos)
{
if (pos == null )
{
return ;
}
else if (pos.next == null )
{
Console.WriteLine( "This is last node, require head, can't be freed\n" );
return ;
}
pos.data = pos.next.data;
pos.next = pos.next.next;
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
public void print_list()
{
Node temp = head;
while (temp != null )
{
Console.Write(temp.data + "->" );
temp = temp.next;
}
Console.WriteLine( "NULL\n" );
}
}
class Program
{
static void Main( string [] args)
{
LinkedList llist = new LinkedList();
llist.push(20);
llist.push(4);
llist.push(15);
llist.push(35);
Console.WriteLine( "Initial Linked List : " );
llist.print_list();
Node del_node = llist.head.next;
llist.delete_node_without_head(del_node);
Console.WriteLine( "Final Linked List after deletion of 15 : " );
llist.print_list();
}
}
|
Javascript
class Node{
constructor(val){
this .data = val;
this .next = null ;
}
}
class LinkedList{
constructor(){
this .head = null ;
}
delete_node_without_head(pos){
if (pos == null )
return ;
else if (pos.next == null ){
console.log( "This is last node, require head, can't be freed\n" );
return ;
}
pos.data = pos.next.data;
pos.next = pos.next.next;
}
push(new_data){
let new_node = new Node(new_data);
new_node.next = this .head;
this .head = new_node;
}
print_list(){
let temp = this .head;
while (temp != null ){
console.log(temp.data + "->" );
temp = temp.next;
}
console.log( "NULL \n\n" );
}
}
let llist = new LinkedList();
llist.push(20);
llist.push(4);
llist.push(15);
llist.push(35);
console.log( "Initial Linked List : \n" );
llist.print_list();
let del_node = llist.head.next;
llist.delete_node_without_head(del_node);
console.log( "Final Linked List after deletion of 15 : \n" );
llist.print_list();
|
Output
Initial Linked List:
35 -> 15 -> 4 -> 20 -> NULL
Final Linked List after deletion of 15:
35 -> 4 -> 20 -> NULL
Time complexity: O(1) since performing constant operations and modifying only a single pointer to delete node
Auxiliary Space: O(1)
Please see Given only a pointer to a node to be deleted in a singly linked list, how do you delete it for more details and complete implementation.
Share your thoughts in the comments
Please Login to comment...