Google SDE Sheet: Interview Questions and Answers
Last Updated :
15 Feb, 2024
Google is an American multinational technology company specializing in search engine technology, online advertising, cloud computing, computer software, quantum computing, e-commerce, and artificial intelligence. It is a dream of many people to work for Google. This sheet will assist you to land a job at Google, we have compiled all the interview questions and answers..
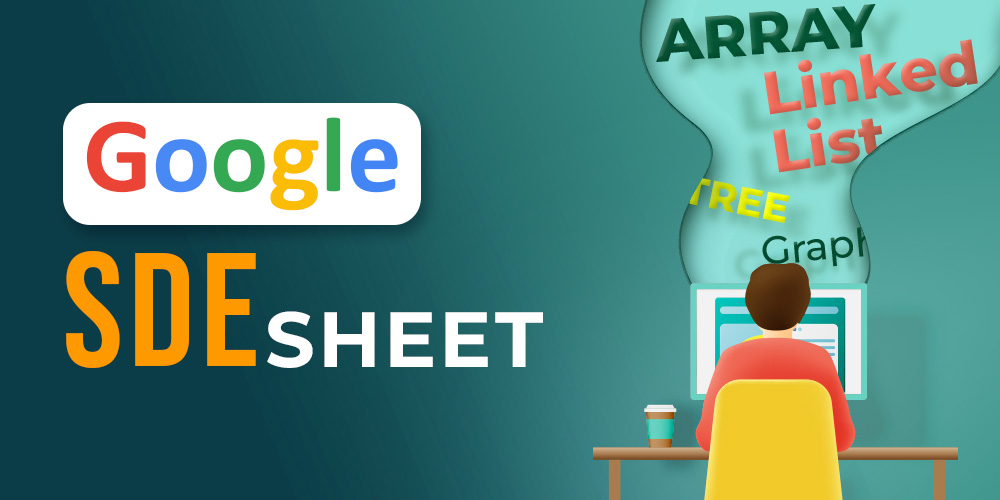
Google SDE Sheet
For top companies like Google, one must have solid Data Structure concepts, good communication skills, and an analytical mindset. Steps to follow to get a chance at Google are:
- Online Application
- Online assessments: After Google approves your application (resume/cover letter), you will be required to take an online assessment. Here’s an overview of the online assessment:
Number of questions: 2 questions
Time allowed: 90 minutes
Topics: typical algorithm/data structure questions
- Technical Phone Screen: (30-60 minutes) Google’s interview process begins with a technical phone interview for experienced software engineers. During this stage, tech leads conduct interviews via Google Meet or Hangouts.
- Onsite: Onsite is the last stop. On average, 4 to 6 rounds should be finished in a day. Each round should take around 45 minutes and include questions on both system design and code. Interviewees note that more coding is done than system design, and expectations change depending on your skill level.
- Behavioral Skills(Googleyness): As part of its hiring process, Google wants to determine whether a potential employee has “Googleyness”, a value that the company uses to describe those who are culturally fit.
Google SDE Roadmap
Why this sheet?
Google coding interviews can be a tough nut to crack. The questions in google interviews cover a wide range of topics and are difficult and specific to Google. This sheet is like a helping hand for those preparing to get a job at Google. It covers all the important and popular technical questions asked in Google Interviews so far. Questions in this sheet are grouped topic-wise and cover all major DSA topics that have weightage in Google interviews.
Computer Science Concepts: Google focuses on the following Computer Science Subjects in their interviews:
Data Structure & Algorithms
Array: An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together.
String: Strings are defined as an array of characters. The difference between a character array and a string is the string is terminated with a special character ‘\0’.
Linked List: A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations.
Stack and Queue:
- Stack: A stack is a linear data structure in which elements can be inserted and deleted only from one side of the list, called the top. A stack follows the LIFO (Last In First Out) principle.
- Queue: A queue is a linear data structure in which elements can be inserted only from one side of the list called rear, and the elements can be deleted only from the other side called the front. The queue data structure follows the FIFO (First In First Out) principle.
Searching: Searching Algorithms are designed to check for an element or retrieve an element from any data structure where it is stored.
Sorting: A Sorting Algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. The comparison operator is used to decide the new order of elements in the respective data structure.
Hash and Heap:
- Hash: Hashing is a technique or process of mapping keys, and values into the hash table by using a hash function. It is done for faster access to elements. The efficiency of mapping depends on the efficiency of the hash function used
- Heap: A Heap is a special Tree-based data structure in which the tree is a complete binary tree. Heap and hash is an efficient implementation of a priority queue. The linear hash function monotonically maps keys to buckets, and each bucket is a heap.
Trees: A tree is non-linear and a hierarchical data structure consisting of a collection of nodes such that each node of the tree stores a value, a list of references to nodes (the “children”). :
Graph: A Graph is a non-linear data structure consisting of nodes and edges. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph.
Dynamic Programming: Dynamic Programming is mainly an optimization over plain recursion. Wherever we see a recursive solution that has repeated calls for same inputs, we can optimize it using Dynamic Programming.
Projects
Thoroughly revise all the work you have done till now in your projects. The grilling about projects can sometimes be very deep. Also, choose your words before you speak. Mention only those topics where you think you are fine to be grilled upon. If you haven’t made a project then take an idea from GFG Projects and start working on it.
System Design
System Design is the process of designing the architecture, components, and interfaces for a system so that it meets the end-user requirements. System Design for tech interviews is something that can’t be ignored!
Almost every IT giant whether it be Facebook, Amazon, Google, or any other asks various questions based on System Design concepts such as scalability, load-balancing, caching, etc. in the interview.
This specifically designed System Design tutorial & System Design Course will help you to learn and master System Design concepts in the most efficient way from basics to advanced level.
Behavioral Skills
Many people are very afraid of behavioural interview questions in technical interviews as they seem to be just something most programmers are not good at. Do you know that less than 10% of candidates passed their on-site interviews as reported in Silicon Valley? Don’t forget that those who failed have already passed the phone screen.
Share your thoughts in the comments
Please Login to comment...