Every great programmer, like you, works to develop code that is as efficient as possible and produces the best results. So the main goal of every programmer is not to merely write a code that works but to write a well-structured code that works efficiently. This skill can only be developed if one has a solid understanding of Data Structures and Algorithms. Keeping this in mind, we have created a complete beginner’s guide for you to learn and master DSA. By following this ultimate beginner’s guide for DSA, you can grow your DSA skills from beginner to master level, for sure. Worries about where to start? Don’t worry, we got you covered.
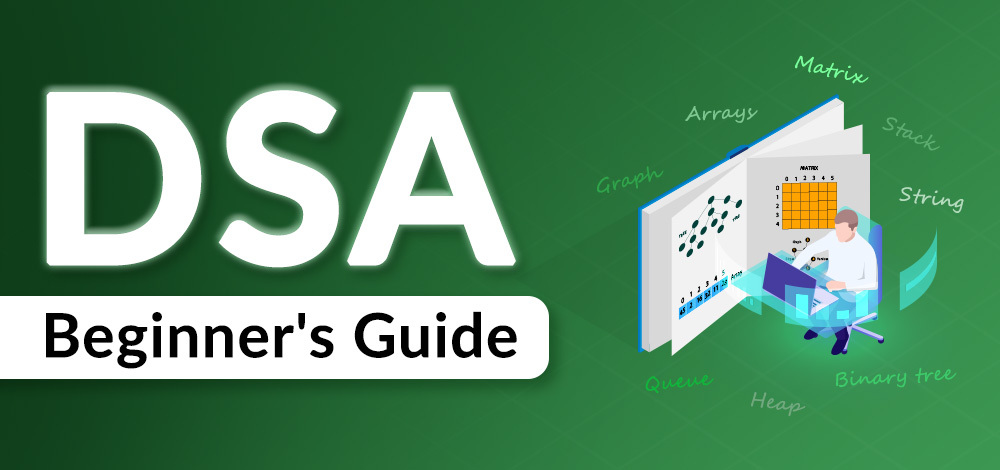
The Ultimate Beginner’s Guide for DSA
In this post, we will be discussing Data Structures and Algorithms in detail, from a beginner’s perspective. So in this beginner’s guide for DSA, you will learn about the basics of DSA, why and how to get started with DSA, learning, strategy, resources, and much more. So, let’s get started.
Table of Contents/Roadmap
DSA – Self Paced Course
In order to crack any technical interview rounds, having a proper understanding of data structures and algorithms is a must. Learning DSA will help you write an optimised code that runs faster and stands out from the crowd. The only way you can achieve it is through learning DSA. Start your journey today and get prepared for interviews in top-notch product or service-based companies like Microsoft, Amazon, Adobe, etc. with our DSA self-paced course. Learn more!
What is Data Structure And Algorithm (DSA)?
In today’s world, Data Structure and Algorithms are an integral part of computer science. It’s much easier to understand data structures and algorithms if we break them down into two parts:
- Data structures: A Data Structure is a way to organize data in a form that is accessible to computers. It allows the processing of a large amount of data in a relatively short period of time.
- Algorithms: Algorithms are well-defined sets of instructions designed that are used to solve problems or perform a task. To explain in simpler terms, it is a set of operations performed in a step-by-step manner to execute a task.
How are Data Structures and Algorithms related?
Data Structure and Algorithms are different but they are very much interrelated, let’s see how:
- A Data Structure is an entity that contains information used by algorithms.
- A Data Structure allows you to store elements in memory and provides functions for manipulating stored elements.
- Some Data Structures are more suitable for solving specific problems.
- We implement an algorithm on our computer using Data Structures, which allows us to store the data that will be used to solve the problem.
Why Data Structures and Algorithms are important to learn?
You must have heard about Data Structures and Algorithms in the programming world every now and then. So it is very common to find yourself in a dilemma as to why you should learn Data Structure and Algorithms? Data Structure and Algorithms help in understanding the nature of the problem at a deeper level and thereby providing a solution that solves the problem in the best way possible.
Let us explain through some scenarios in this beginner’s guide for DSA as to why DSA is important to learn:
Problem statement 1: You went to store and find the records of some particular patients in the hospital. The challenges that you will face without having any knowledge about data structure:
- Challenge 1: How will you keep records of tens of thousands of patients?
- Challenge 2: How to search records of a particular patient quickly?
Problem statement 2: There are various seasons of League Cricket matches to be held every year. So there has to be a way to efficiently find loads of metrics from across seasons.
- Challenge 1: How will you keep the details of each match played, one after the other?
- Challenge 2: How to find metrics of particular scenarios in various instances of the match?
Each of the above problems and much more needs proper knowledge and implementation of Data Structures and Algorithms for efficient storage, searching, and other operations with the best results.
How to learn Data Structures and Algorithms?
Now that we have covered the basics of Data Structures and Algorithms in this beginner’s guide for DSA, it is now time to learn DSA. You can follow the following step-by-step method to master DSA from scratch:
- Learn about fundamental concepts of Programming
- Choose a programming language to implement those concepts
- Start learning with Data structures
- Get to know about Algorithms
- Learn, and practice about Complexity analysis
- FInding the best resources to practice DSA
- Practice and practice problems based on DSA
1. Learn About Fundamental Concepts of Programming:
No matter what DSA concept you are using, you need a programming language to implement those concepts. Hence it is must to have a fundamental understanding of programming languages. There are some basic concepts of programming that you must know regardless of the language, such as:
- Variables and Data Types
- Control Structures (Conditional Statements and Loops)
- Functions and how to use them
- Object-Oriented Programming concepts
- Basic Syntax
- Debugging
- IDEs and Coding Environments
1.a) Variables and Data Types
A variable is a memory location that holds values of a given type. It is the basic unit of storage in a program. Variables are created using a declaration or keyword that varies across languages. Variable names are usually alphanumeric, which contains a-z and 0-9, and can also include special characters like underscore ( _ ) or the dollar sign ( $ ).
All variables use data-type during declaration to restrict the type of data to be stored. Therefore, we can say that data types are used to tell the variables the type of data they can store. Whenever a variable is defined, the compiler allocates some memory for that variable based on the data type with which it is declared. Every data type requires a different amount of memory.
The following are the most common data types:
- Integer: It is the most common numeric data type that stores whole numbers without a fractional component. Example: 110, 123, 0 etc.
- Floating Point: It’s also a numeric data type for storing fractional values. Example: 110.12, 123.001, 0.5 etc.
- Character: It is used to store a single digit, letter, symbol, punctuation mark, or blank space.
- Boolean: It is used to represent the values true and false.
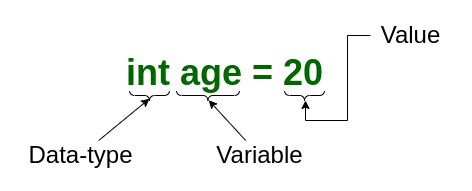
Variables and Data Types
1.b) Control Structures (Conditional Statements and Loops)
In programming, the codes are executed in sequential format. So there might be a need where you need to skip a few lines of codes based on some conditions or repeat a few lines of codes or something just like that. Programming languages provide concepts of control structures to handle such situations.
Control structures are programming concepts that break the sequential flow of execution and mandate the compiler/interpreter to execute code in a specific format for some specific lines of code. There are mainly two types of control structures:
- Conditional Statements: This control structure is used to execute some line of code if a particular condition is fulfilled. If not, then it executes some other line of code. Such concepts include- if-else statements, switch statements, try-catch statements, etc.
- Loop Statements: This control structure is used to execute some lines of code repetitively till an underlying condition is fulfilled. Such concepts include- for loop, while loop, and do-while loop.
1.c) Functions
A function is a set of statements that take inputs, do some specific computation, and produce output.
The idea is to put some commonly or repeatedly done tasks together and make a function so that instead of writing the same code again and again for different inputs, we can call the function.
The general form of a function is:
return_type function_name([ arg1_type arg1_name, … ]) { code }
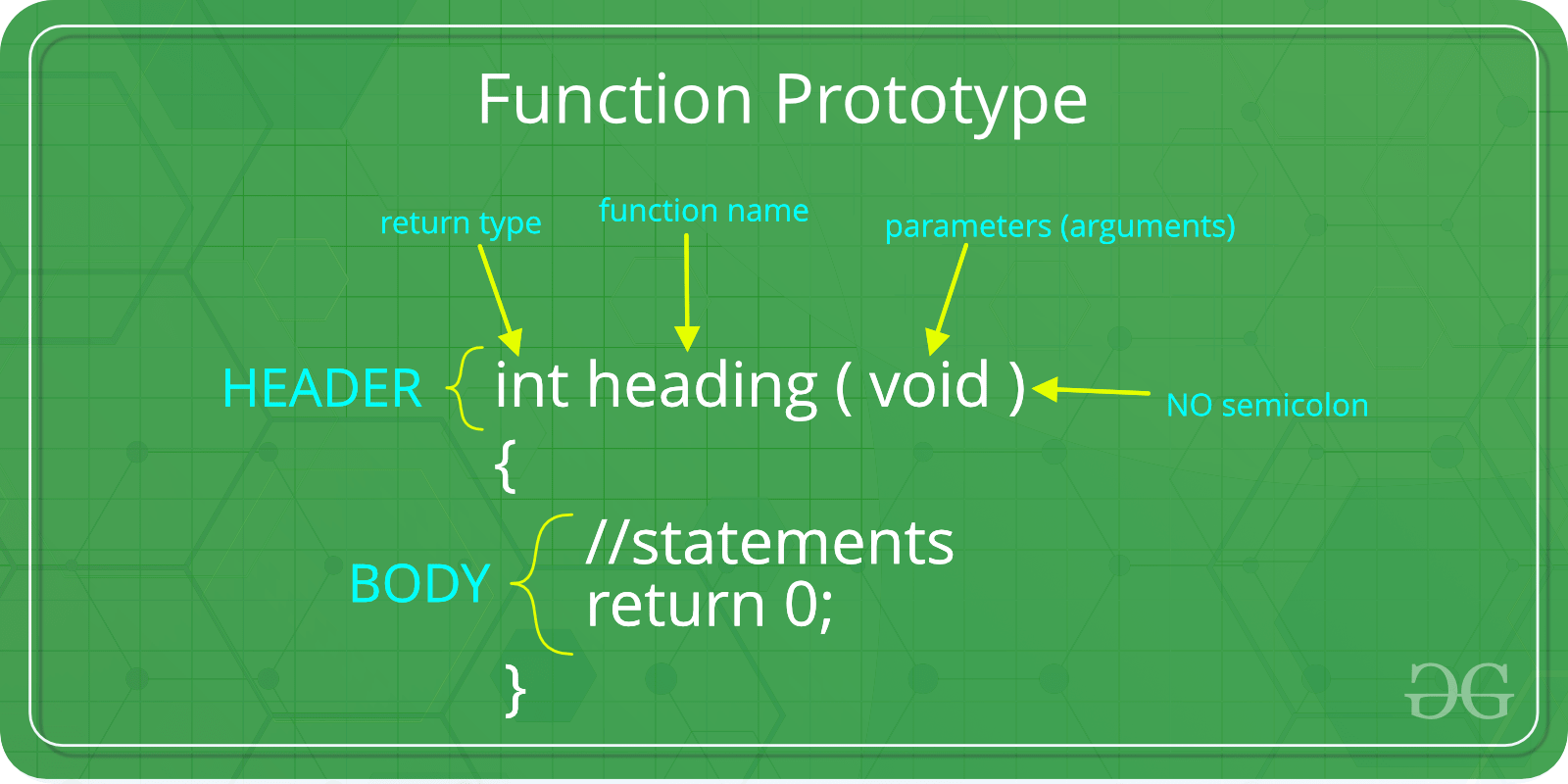
Functions in C/C++
1.d) Object-Oriented Programming concepts
As the name suggests, Object-Oriented Programming or OOPs refers to languages that uses objects in programming. Object-oriented programming aims to implement real-world entities like inheritance, hiding, polymorphism etc in programming. The main aim of OOP is to bind together the data and the functions that operate on them so that no other part of the code can access this data except that function.
OOPs, Concepts are as follows:
- Class
- Object
- Method and method passing
- Pillars of OOPS
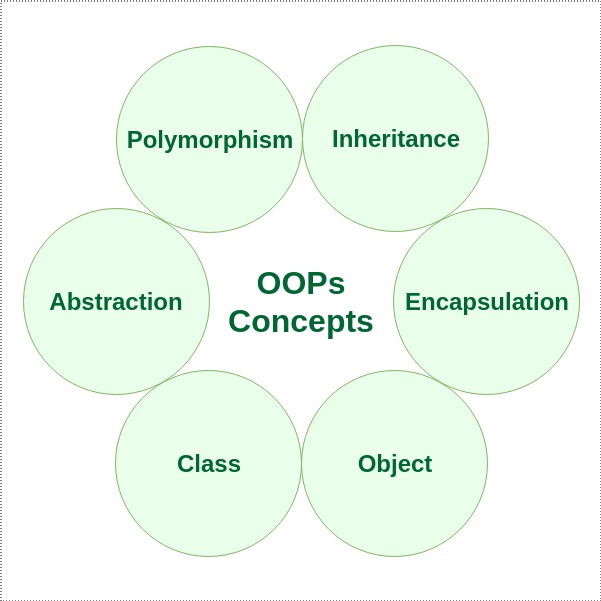
Object-Oriented Programming concepts
1.e) Basic syntax
Every programming language has its own syntax, and you’ll need to understand the basics of the one you’re learning. The set of rules that define a language’s structure is referred to as syntax. Without the syntax of a programming language, it is nearly impossible to read or understand it.
The basic syntax of some of the most used programming languages can be learned using the below links:
For example: Below is the example to declare variable named geeks and assign the value “Hello World” to it.
C++
string geeks = "Hello World";
Java
String geeks = "Hello World";
Python3
C#
string geeks = "Hello World";
Javascript
let geeks = "Hello World";
1.f) Debugging
One of the most terrible and painful things for programmers is errors, and no matter what, every programmer has to go through this phase while working on a project. You start working on a project with full enthusiasm. You wrote thousands of lines of clean code, and everything seems to work fine there, but when you try to run the project, it doesn’t work or it doesn’t behave in the way you want it to behave. A lot of programmers might have faced this issue in their careers, and believe us, it becomes even more frustrating you come across them. The only solution you have in such situations is debugging your code.
Debugging is all about figuring out the source of a problem than identifying its causes, testing your hypothesis, and trying every possible solution to eliminate the cause behind its unexpected behavior.
1.g) IDEs and Coding Environments
Integrated Development Environments (IDEs) are software tools that programmers use to write code and arrange text groups. It increases a programmer’s speed and productivity with features like code compilation, completion, syntax highlighting, debugging, and others.
Below are some common examples of IDEs are:
Here are some of the major IDEs based on the programming language you choose:
2. Choose a programming language
A programming language is a computer language that is used to interact with computers. It is a set of instructions for completing any task. So it becomes important to choose a particular programming language and it is based on your choices, like Java, C, C++, Python, or any other language. This will help you to implement your ideas that a computer can understand and take action on it.
You can easily learn the programming language of your choice with the help of curated tutorials on some of the most popular programming languages, such as:
3. Start learning with Data structures
A Data Structure is a particular way of organizing data in a computer so that it can be used effectively. The choice of your data structure will always depend on your requirements and usage scenario.
Now you must be wondering why to use Data structures when there are concepts of data types, variables, and objects on the programming language level!
Let us explain this to you with the help of a simple example.
Case 1: Consider that you want to store the records of 5 persons in your system. You might say that lets just create 5 variables, one for each person, and store the data effectively.
Alright, we agree. But now lets consider next scenario.
Case 2: Consider you want to store similar data for lets say 1000 persons now. How will you achieve that?
If you try to create 1000 variables, the solution might cost you more than the problem (as the number of persons can be indefinite).
So here comes the concepts of Data structures. With the help of data structures, like Array in above scenario, you can store any amount of data, in the most efficient way possible.
Which Data Structure to use and in which situation?
Choosing the type of data structure is completely dependent on the following parameters:
- Type of data to be stored
- Amount of data to be stored
- Possible operations to be done upon the stored data
- Cost of storage vs cost of using a data structure
In general terms of programming, the type of data structure to be used is chosen on the type of data to be stored- linear or non-linear. Based on this, the data structures can be classified into two categories:
- Linear data structure: Linear data structures are data structures with elements arranged sequentially or linearly, where each element is connected to the previous and next adjacent elements.
Below are some linear data structures: - Non-linear data structure: Non-linear data structures are those in which data elements are not arranged sequentially or linearly. It utilizes computer memory efficiently in comparison to a linear data structure.
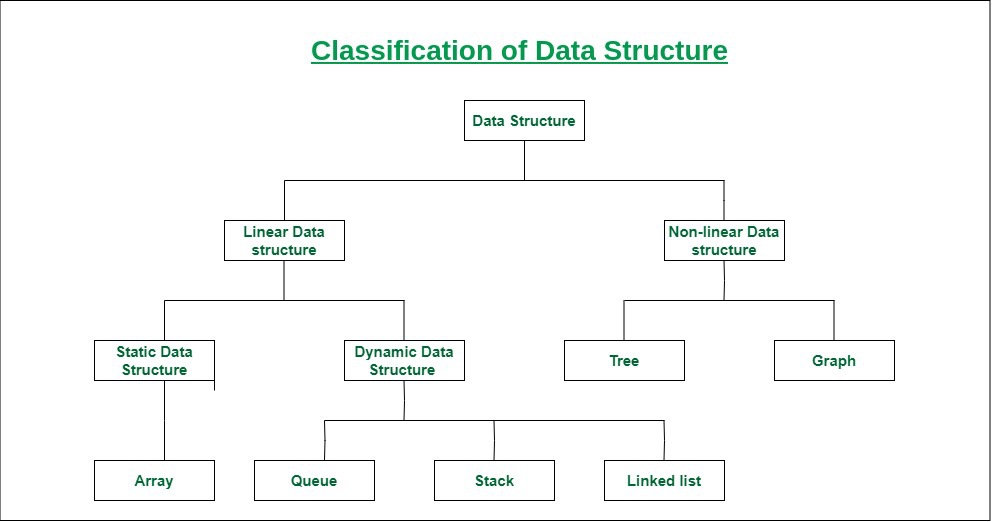
Classification of Data Structure
4. Get to know about Algorithms
The word Algorithm means ”A set of rules to be followed in calculations or other problem-solving operations” Or ”A procedure for solving a mathematical problem in a finite number of steps that frequently by recursive operations“.
Therefore Algorithm refers to a sequence of finite steps to solve a particular problem. Algorithms can be simple and complex depending on what you want to achieve.
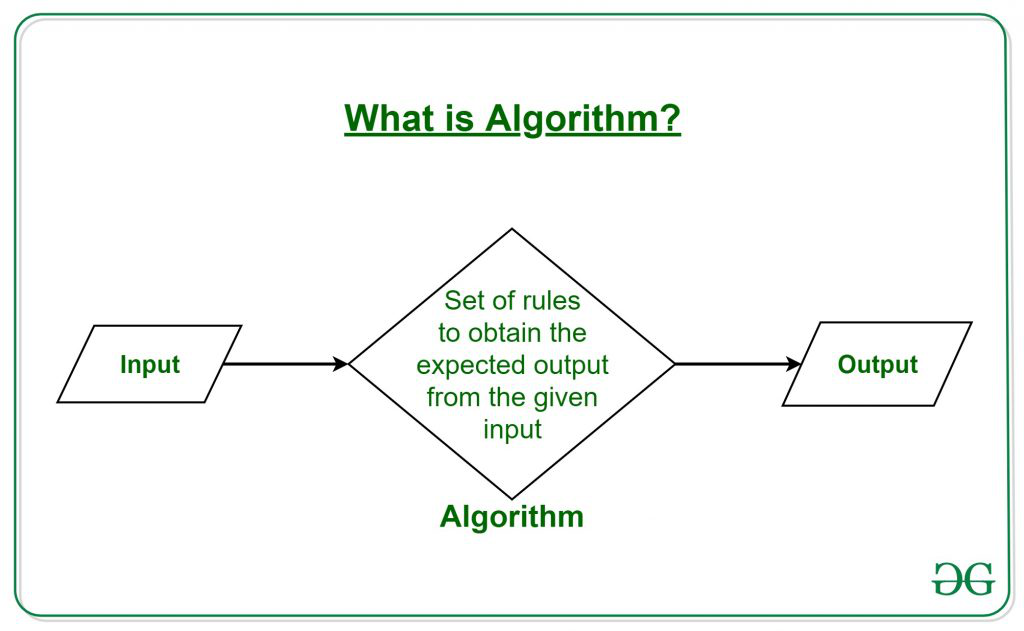
What is an Algorithm
Why do we need Algorithms?
Now you must be thinking, what is the need for an Algorithm and where is it used! So let’s take the previous scenario where you are storing the records of numerous persons in the system. Now consider the following scenarios:
- What if you need to find a person with a particular name?
- What if you need to align records with respect to some parameters?
- What if you need to update some record, or even delete it?
- What if you need to perform some other processing on stored information?
- What if…?
- What if…?
The answer to all your “What if…s” is Algorithm. The algorithm enables us to perform some tasks in the best possible way such that the cost of performing the task with respect to time and memory is optimized. This in turn helps us to reduce the overall cost of the program and thus leads us to profits.
Some of the major algorithms include:
- Brute Force Algorithm: It is the simplest approach for a problem. A brute force algorithm is the first approach that comes to finding when we see a problem.
- Recursive Algorithm: A recursive algorithm is based on recursion. In this case, a problem is broken into several sub-parts and called the same function again and again.
- Backtracking Algorithm: The backtracking algorithm basically builds the solution by searching among all possible solutions. Using this algorithm, we keep on building the solution following criteria. Whenever a solution fails we trace back to the failure point and build on the next solution and continue this process till we find the solution or all possible solutions are looked after.
- Searching Algorithm: Searching algorithms are the ones that are used for searching elements or groups of elements from a particular data structure. They can be of different types based on their approach or the data structure in which the element should be found.
- Sorting Algorithm: Sorting is arranging a group of data in a particular manner according to the requirement. The algorithms which help in performing this function are called sorting algorithms. Generally sorting algorithms are used to sort groups of data in an increasing or decreasing manner.
- Hashing Algorithm: Hashing algorithms work similarly to the searching algorithm. But they contain an index with a key ID. In hashing, a key is assigned to specific data.
- Divide and Conquer Algorithm: This algorithm breaks a problem into sub-problems, solves a single sub-problem, and merges the solutions together to get the final solution. It consists of the following three steps:
- Greedy Algorithm: In this type of algorithm the solution is built part by part. The solution of the next part is built based on the immediate benefit of the next part. The one solution giving the most benefit will be chosen as the solution for the next part.
- Dynamic Programming Algorithm: This algorithm uses the concept of using the already found solution to avoid repetitive calculation of the same part of the problem. It divides the problem into smaller overlapping subproblems and solves them.
- Randomized Algorithm: In the randomized algorithm we use a random number so it gives immediate benefit. The random number helps in deciding the expected outcome.
5. Learn, and Practice Complexity Analysis
Why performance analysis?
There are many important things that should be taken care of, like user-friendliness, modularity, security, maintainability, etc for a code. Then Why worry about performance?
The answer to this is simple. We can have all the above things only if we have performance. So performance is like currency through which we can buy all the above things. To summarize, performance == scale.
Imagine a text editor that can load 1000 pages, but can spell check 1 page per minute OR an image editor that takes 1 hour to rotate your image 90 degrees left OR … you get it. If a software feature can not cope with the scale of tasks users need to perform – it is as good as dead.
How to measure the performance of a code?
The performance of a code is measured by the term “Complexity“, which means by how much time and/or space an algorithm requires for an input of a given size (n).
The complexity of a code/algorithm can be measured in terms of the following concepts:
- Time Complexity: Time complexity is used to measure the amount of time required to execute the code.
The time complexity of an algorithm is commonly expressed using asymptotic notations:- Big-O Notation (Ο) – This notation specifically describes the worst-case scenario. This is mostly used notation in the analysis of a code, which gives an upper bound of the running time of the code (or the amount of memory used in terms of input size).
- Omega Notation (Ω) – This notation specifically describes the best-case scenario.
- Theta Notation (θ) – This notation represents the average complexity of an algorithm.
- Space Complexity: Space complexity means the amount of space required to execute successfully the functionalities of the code.
- Auxiliary Space: You will also come across the term Auxiliary Space very commonly in DSA, which refers to the extra space used in the program other than the input data structure.
To learn about complexity analysis in detail, you can refer to our complete set of articles on the Analysis of Algorithms.
6. Finding the best resources for DSA
A guide is never complete without proper resources and references. Similarly, in this ultimate beginner’s guide for DSA, we have compiled comprehensive references of resources that you can opt for to learn DSA.
There are a lot of resources available on the market and the internet, such as paid or unpaid video lectures, tutorials, articles, books, etc, and rather than making students proficient in Data Structure and Algorithms, a lack of guidance results in ineffective learning resources that kill their interest and curiosity in the subject.
Finding relevant material can be a challenge but using a strategic plan will make your learning more convenient and efficient.
You can learn Data Structure and Algorithms from various text, video, or hybrid types of resources such as:
- Textbooks:
- “Introduction to Algorithms” by T.H.Cormen,
- “Algorithms”, by Robert Sedgewick
- “Data Structures and Algorithms Made Easy in Java”, by Narasimha Karumanchi
- “Data structures and algorithms in C++”, by Adam Drozdek
- Self-Paced Courses:
- Live Courses:
7. Practice and Practice
After learning the fundamental of programming, choosing a programming language, and learning about Data Structure and Algorithms and their space-time complexity, it becomes necessary to practice the problem based on different data structures and algorithms.
We have curated the selective list of problems for you to solve as a beginner for DSA, and named it the Beginner’s DSA Sheet. The problem on the sheet includes:
For practicing problems on individual data structures and algorithms, you can use the following links:
Apart from these, there are many other practice problems that you can refer based on their respective difficulties:
You can also try to solve the most asked interview questions based on the list curated by us at:
You can also try our curated lists of problems below articles:
Conclusion
Learning Data Structures and Algorithms is a lengthy and difficult process, but with the help of this beginner’s guide for DSA, we can assure you that it is achievable, if you follow the above path of learning, revisions, and practicing questions consistently.
During the learning phase, the most important thing to keep in mind is that learning is a continuous process. so, you should be consistent while learning and devote at least a small amount of time each day. If you will inconsistent then you might forget the previously learned topics, and you will have to start from scratch, which can ruin your all hard work.
As a final word of advice, take advantage of the fact that practice makes a man perfect, and that there will be ups and downs on your journey – don’t be afraid to keep learning and growing.
Related Articles:
Share your thoughts in the comments
Please Login to comment...