Python – Tkinter askquestion Dialog
Last Updated :
27 Mar, 2023
In Python, There Are Several Libraries for Graphical User Interface. Tkinter is one of them that is most useful. It is a standard interface. Tkinter is easy to use and provides several functions for building efficient applications. In Every Application, we need some Message to Display like “Do You Want To Close ” or showing any warning or Something information. For this Tkinter provide a library like messagebox. By using the message box library we can show several Information, Error, Warning, Cancellation ETC in the form of Message-Box. It has a Different message box for a different purpose.
- showinfo() – To display some important information .
- showwarning() – To display some type of Warning.
- showerror() –To display some Error Message.
- askquestion() – To display a dialog box that asks with two options YES or NO.
- askokcancel() – To display a dialog box that asks with two options OK or CANCEL.
- askretrycancel() – To display a dialog box that asks with two options RETRY or CANCEL.
- askyesnocancel() – To display a dialog box that asks with three options YES or NO or CANCEL.
Syntax of the Message-Box Functions:
messagebox.name_of_function(Title, Message, [, options])
- name_of_function – Function name that which we want to use .
- Title – Message Box’s Title.
- Message – Message that you want to show in the dialog.
- Options –To configure the options.
Askquestion()
This function is used to ask questions to the user. That has only two options YES or NO.
Application of this function:
- We can use this to ask the user if the User want’s to continue.
- We can use this to ask the user if the User wanted to Submit or not.
Syntax:
messagebox.askfunction((Title, Message, [, options])
Example:
Python3
from tkinter import *
from tkinter import messagebox
main = Tk()
def Submit():
messagebox.askquestion( "Form" ,
"Do you want to Submit" )
main.geometry( "100x100" )
B1 = Button(main, text = "Submit" , command = Submit)
B1.pack()
main.mainloop()
|
Output:
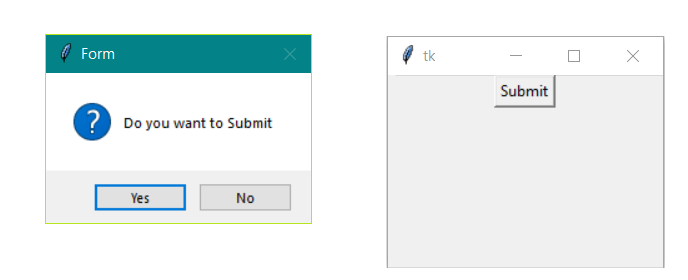
1. Importing the Libraries
To use the GUI functionality in python we have to import the libraries. In the first line, we are importing Tkinter, and second-line we’re importing messagebox library
from tkinter import *
from tkinter import messagebox
2. Main window instance
We have to create an instance or object for the window to TK(); Tk() is a function of Tkinter that create a window that can be referred from the main variable
main = Tk()
3. Set dimension
we set the dimension of the window we can set it in various ways.in this we are setting is by geometry() function of size “100X100”.
top.geometry("100x100")
4. Applying other widget and function
In our example, we create a method named Submit and call the askquestion() and Creating Button and setting it by Pack() function
def Submit():
messagebox.askquestion("Form", "Do you want to Submit")
main.geometry("100x100")
B1 = Button(main, text = "Submit", command = Submit)
B1.pack()
5. mainloop()
This method can be used when all the code is ready to execute.It runs the INFINITE Loop used to run the application. A window will open until the close button is pressed.
ICONS that We can use in Options
- Error
- Info
- Warning
- Question
We can change the icon of the dialog box. The type of icon that we want to use just depends on the application’s need. we have four icons.
Error
messagebox.function_name(Title, Message, icon='error')
Example-
Python3
from tkinter import *
from tkinter import messagebox
main = Tk()
def check():
messagebox.askquestion( "Form" ,
"Is your name correct?" ,
icon = 'error' )
main.geometry( "100x100" )
B1 = Button(main, text = "check" , command = check)
B1.pack()
main.mainloop()
|
Output:
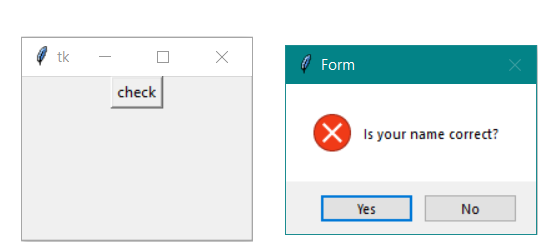
info
messagebox.function_name(Title, Message, icon='info')
Example-
Python3
from tkinter import *
from tkinter import messagebox
main = Tk()
def check():
messagebox.askquestion( "Form" ,
"do you want to continue" ,
icon = 'info' )
main.geometry( "100x100" )
B1 = Button(main, text = "check" , command = check)
B1.pack()
main.mainloop()
|
Output:
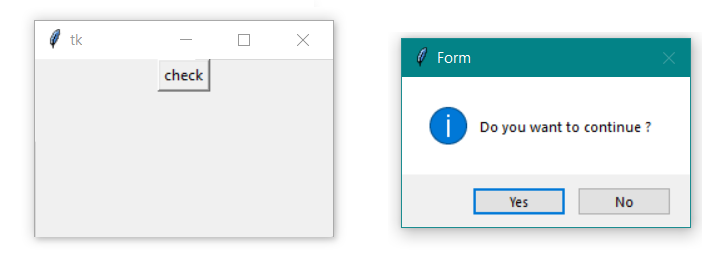
Question
messagebox.function_name(Title, Message, icon='question')
Example-
Python3
from tkinter import *
from tkinter import messagebox
main = Tk()
def check():
messagebox.askquestion( "Form" ,
"are you 18+" ,
icon = 'question' )
main.geometry( "100x100" )
B1 = Button(main, text = "check" , command = check)
B1.pack()
main.mainloop()
|
Output:
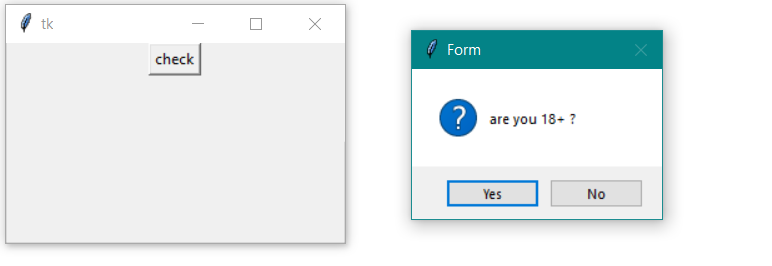
Warning
messagebox.function_name(Title, Message, icon='warning')
Example-
Python3
from tkinter import *
from tkinter import messagebox
main = Tk()
def check():
messagebox.askquestion( "Form" ,
"Gender is empty?" ,
icon = 'warning' )
main.geometry( "100x100" )
B1 = Button(main, text = "check" , command = check)
B1.pack()
main.mainloop()
|
Output:
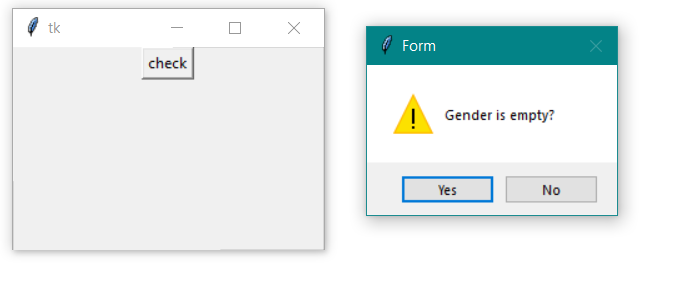
Share your thoughts in the comments
Please Login to comment...