Geometry Method in Python Tkinter
Last Updated :
20 Mar, 2023
Tkinter is a Python module that is used to develop GUI (Graphical User Interface) applications. It comes along with Python, so you do not have to install it using the pip command.
Tkinter provides many methods, one of them is the geometry() method. This method is used to set the dimensions of the Tkinter window and is used to set the position of the main window on the user’s desktop.
Tkinter window without using geometry method.
Python3
from tkinter import Tk, mainloop, TOP
from tkinter.ttk import Button
root = Tk()
button = Button(root, text = 'Geeks' )
button.pack(side = TOP, pady = 5 )
root.mainloop()
|
Output:
As soon as you run the application, you’ll see the position of the Tkinter window is at the northwest position of the screen and the size of the window is also small as shown in the output below.
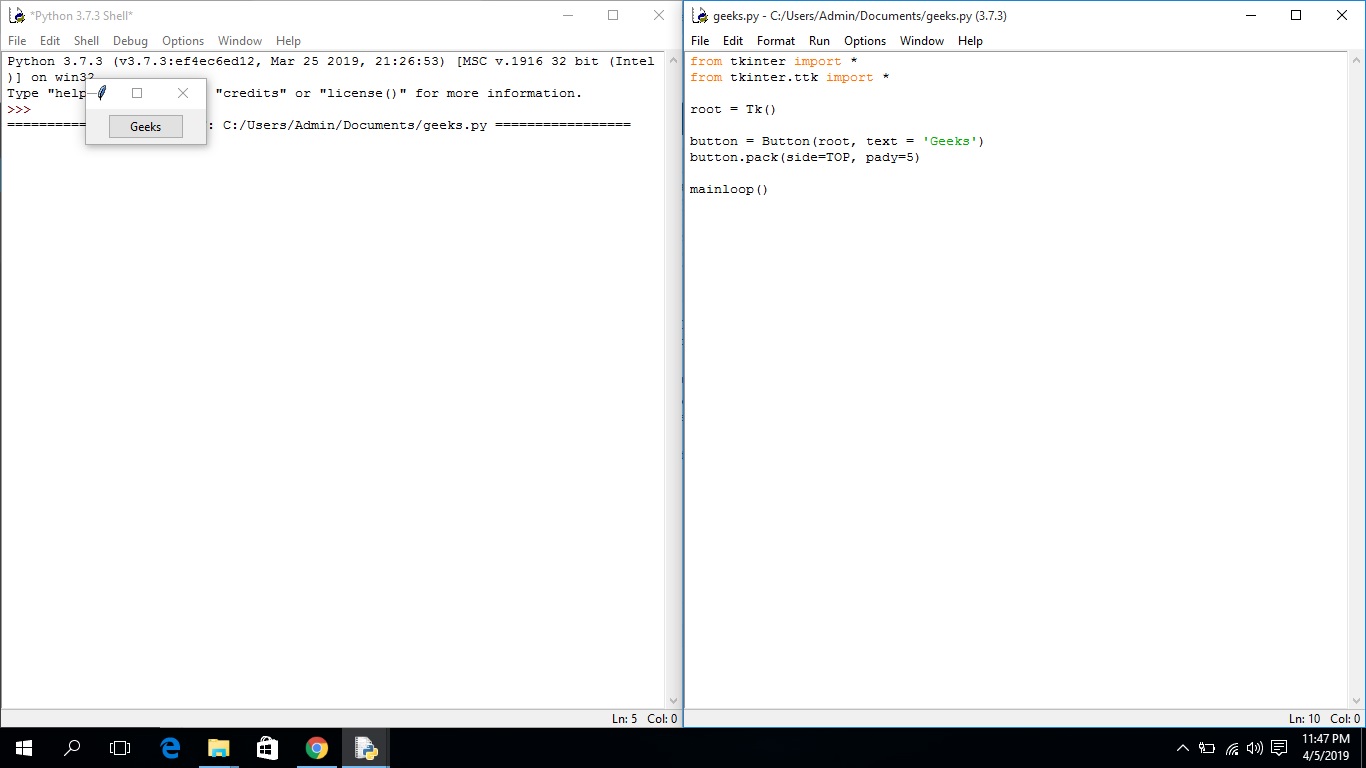
Tkinter window without geometry method
Examples of Tkinter Geometry Method in Python
Now, let us see a few examples of using the geometry method in Python Tkinter.
Using the Tkinter geometry method to set the dimensions of the window
Python3
from tkinter import Tk, mainloop, TOP
from tkinter.ttk import Button
root = Tk()
root.geometry( '200x150' )
button = Button(root, text = 'Geeks' )
button.pack(side = TOP, pady = 5 )
root.mainloop()
|
Output:
After running the application, you’ll see that the size of the Tkinter window has changed, but the position on the screen is the same.
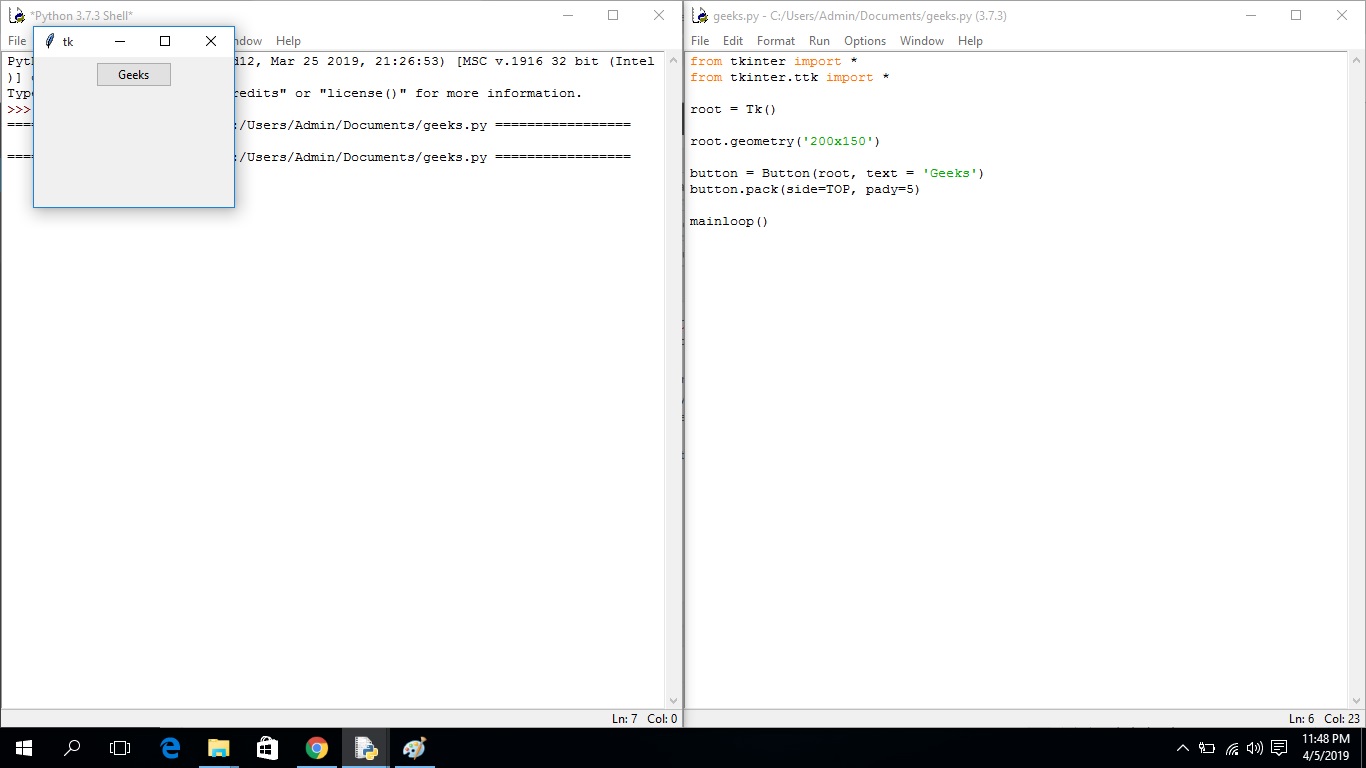
geometry method to set window dimensions
Using the geometry method to set the dimensions and positions of the Tkinter window.
Python3
from tkinter import Tk, mainloop, TOP
from tkinter.ttk import Button
root = Tk()
root.geometry( '200x150+400+300' )
button = Button(root, text = 'Geeks' )
button.pack(side = TOP, pady = 5 )
root.mainloop()
|
Output:
When you run the application, you’ll observe that the position and size both are changed. Now the Tkinter window is appearing at a different position (400 shifted on X-axis and 300 shifted on Y-axis).
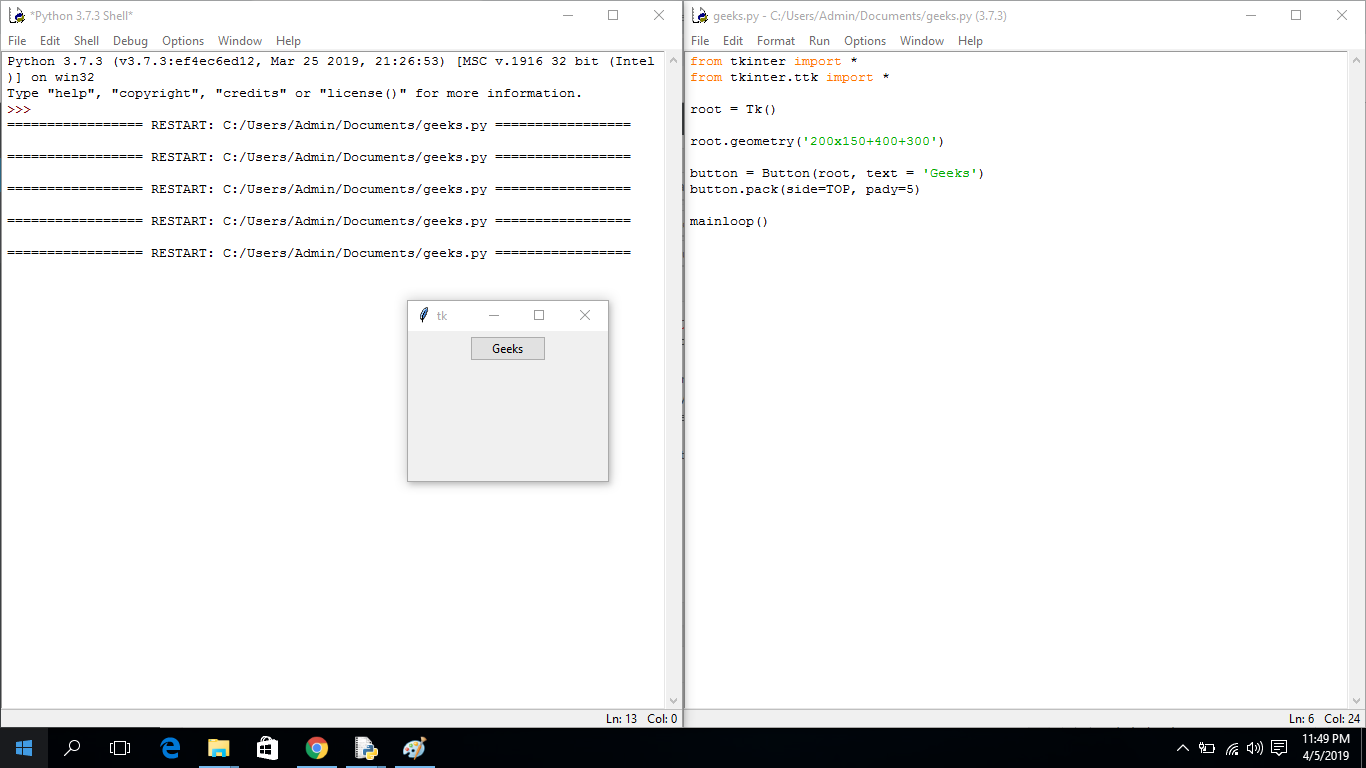
geometry method to set the dimensions and position of the Tkinter window
Note: We can also pass a variable argument in the geometry method, but it should be in the form (variable1) x (variable2); otherwise, it will raise an error.
Share your thoughts in the comments
Please Login to comment...