RadioButton in Tkinter | Python
Last Updated :
16 Feb, 2021
The Radiobutton is a standard Tkinter widget used to implement one-of-many selections. Radiobuttons can contain text or images, and you can associate a Python function or method with each button. When the button is pressed, Tkinter automatically calls that function or method.
Syntax:
button = Radiobutton(master, text=”Name on Button”, variable = “shared variable”, value = “values of each button”, options = values, …)
shared variable = A Tkinter variable shared among all Radio buttons
value = each radiobutton should have different value otherwise more than 1 radiobutton will get selected.
Code #1:
Radio buttons, but not in the form of buttons, in form of button box. In order to display button box, indicatoron/indicator option should be set to 0.
Python3
from tkinter import *
master = Tk()
master.geometry( "175x175" )
v = StringVar(master, "1" )
values = { "RadioButton 1" : "1" ,
"RadioButton 2" : "2" ,
"RadioButton 3" : "3" ,
"RadioButton 4" : "4" ,
"RadioButton 5" : "5" }
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value, indicator = 0 ,
background = "light blue" ).pack(fill = X, ipady = 5 )
mainloop()
|
Output:
The background of these button boxes is light blue. Button boxes having a white backgrounds as well as sunken are selected ones.
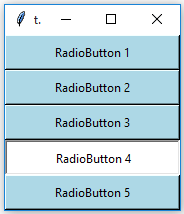
Code #2: Changing button boxes into standard radio buttons. For this remove indicatoron option.
Python3
from tkinter import *
from tkinter.ttk import *
master = Tk()
master.geometry( "175x175" )
v = StringVar(master, "1" )
values = { "RadioButton 1" : "1" ,
"RadioButton 2" : "2" ,
"RadioButton 3" : "3" ,
"RadioButton 4" : "4" ,
"RadioButton 5" : "5" }
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5 )
mainloop()
|
Output:
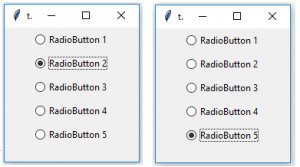
These Radiobuttons are created using tkinter.ttk that is why background option is not available but we can use style class to do styling.
Code #3: Adding Style to Radio Button using style class.
Python3
from tkinter import *
from tkinter.ttk import *
master = Tk()
master.geometry( '175x175' )
v = StringVar(master, "1" )
style = Style(master)
style.configure( "TRadiobutton" , background = "light green" ,
foreground = "red" , font = ( "arial" , 10 , "bold" ))
values = { "RadioButton 1" : "1" ,
"RadioButton 2" : "2" ,
"RadioButton 3" : "3" ,
"RadioButton 4" : "4" ,
"RadioButton 5" : "5" }
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5 )
mainloop()
|
Output:
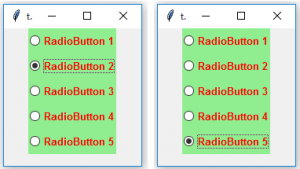
You may observe that font style is changed as well as background and foreground colors are also changed. Here, TRadiobutton is used in style class, it automatically applies styling to all the available Radiobuttons.
Share your thoughts in the comments
Please Login to comment...