Program to calculate area of Circumcircle of an Equilateral Triangle
Last Updated :
20 Feb, 2023
Given the length of sides of an equilateral triangle. We need to write a program to find the area of Circumcircle of the given equilateral triangle.
Examples:
Input : side = 6
Output : Area of circumscribed circle is: 37.69
Input : side = 9
Output : Area of circumscribed circle is: 84.82
All three sides of equilateral triangle are of equal length and all three interior angles are 60 degrees.
Properties of a Circumcircle are as follows:
- The center of the circumcircle is the point where the medians of the equilateral triangle intersect.
- Circumscribed circle of an equilateral triangle is made through the three vertices of an equilateral triangle.
- The radius of a circumcircle of an equilateral triangle is equal to (a / ?3), where ‘a’ is the length of the side of equilateral triangle.
Below image shows an equilateral triangle with circumcircle:
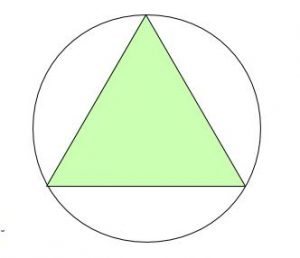
The formula used to calculate the area of circumscribed circle is:
(?*a2)/3
where a is the length of the side of the given equilateral triangle.
How this formulae works?
We know that area of circle = ?*r2, where r is the radius of given circle.
We also know that radius of Circumcircle of an equilateral triangle = (side of the equilateral triangle)/ ?3.
Therefore, area = ?*r2 = ?*a2/3.
C++
#include <iostream>
#include <math.h>
const double pi = 3.14159265358979323846;
using namespace std;
float area_circumscribed( float a)
{
return (a * a * (pi / 3));
}
int main()
{
float a, Area;
a = 6;
Area = area_circumscribed(a);
cout << "Area of CircumCircle :" << Area;
return 0;
}
|
C
#include <stdio.h>
#define PI 3.14159265
float area_circumscribed( float a)
{
return (a * a * (PI / 3));
}
int main()
{
float a = 6;
printf ( "Area of circumscribed circle is :%f" ,
area_circumscribed(a));
return 0;
}
|
Java
import java.lang.*;
class GFG {
static double PI = 3.14159265 ;
public static double area_circumscribed( double a)
{
return (a * a * (PI / 3 ));
}
public static void main(String[] args)
{
double a = 6.0 ;
System.out.println( "Area of circumscribed circle is :"
+ area_circumscribed(a));
}
}
|
Python3
PI = 3.14159265
def area_circumscribed(a):
return (a * a * (PI / 3 ))
a = 6.0
print ( "Area of circumscribed circle is :%f"
% area_circumscribed(a))
|
C#
using System;
class GFG {
static double PI = 3.14159265;
public static double area_circumscribed( double a)
{
return (a * a * (PI / 3));
}
public static void Main()
{
double a = 6.0;
Console.Write( "Area of circumscribed circle is :" +
area_circumscribed(a));
}
}
|
PHP
<?php
$PI = 3.14159265;
function area_circumscribed( $a )
{
global $PI ;
return ( $a * $a * ( $PI / 3));
}
$a = 6;
echo ( "Area of circumscribed circle is :" );
echo (area_circumscribed( $a ));
?>
|
Javascript
<script>
let pi = 3.14159265358979323846;
function area_circumscribed( a)
{
return (a * a * (pi / 3));
}
let a, Area;
a = 6;
Area = area_circumscribed(a);
document.write( "Area of CircumCircle :" + Area.toFixed(7));
</script>
|
Output:
Area of circumscribed circle is :37.6991118
Time Complexity: O(1)
Auxiliary Space: O(1), since no extra space has been taken.
Share your thoughts in the comments
Please Login to comment...