Program to find area of a Trapezoid
Last Updated :
17 Feb, 2023
Definition of Trapezoid :
A Trapezoid is a convex quadrilateral with at least one pair of parallel sides. The parallel sides are called the bases of the trapezoid and the other two sides which are not parallel are referred to as the legs. There can also be two pairs of bases.
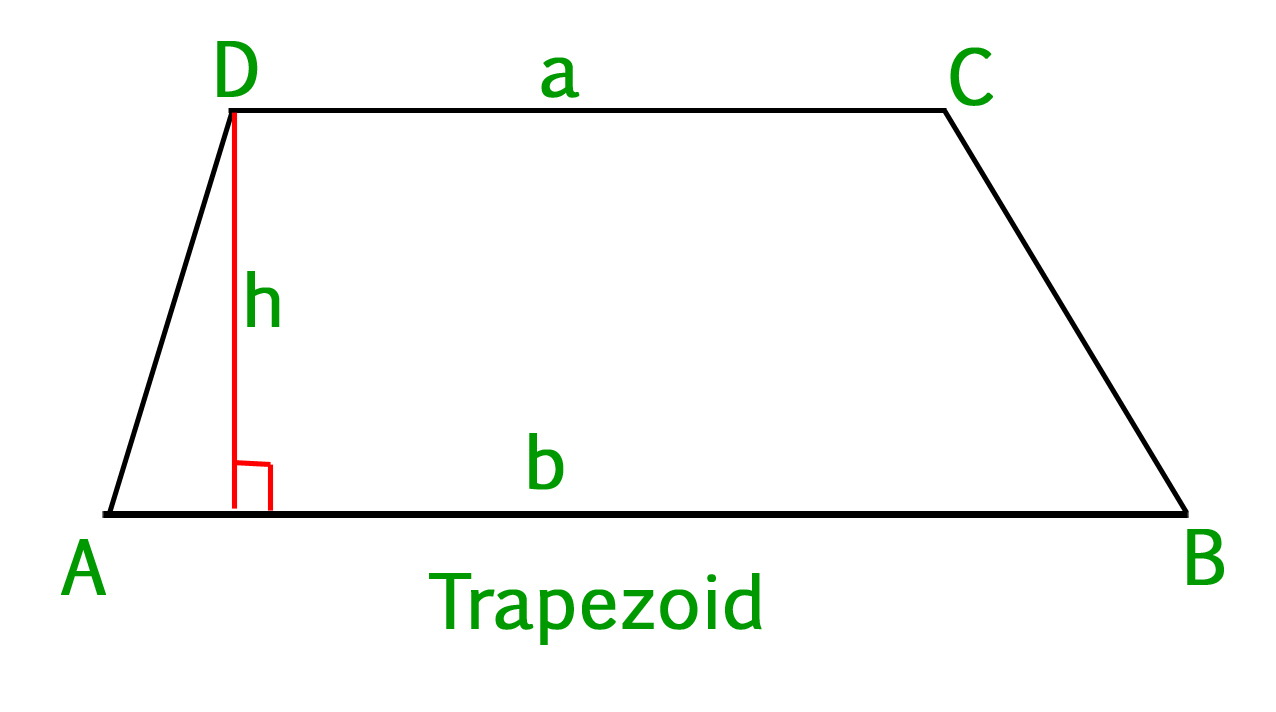
In the above figure CD || AB, so they form the bases and the other two sides i.e., AD and BC form the legs.
The area of a trapezoid can be found by using this simple formula :
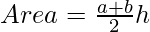
a = base
b = base
h = height
Examples :
Input : base1 = 8, base2 = 10, height = 6
Output : Area is: 54.0
Input :base1 = 4, base2 = 20, height = 7
Output :Area is: 84.0
C++
#include<bits/stdc++.h>
using namespace std;
double Area( int b1, int b2,
int h)
{
return ((b1 + b2) / 2) * h;
}
int main()
{
int base1 = 8, base2 = 10,
height = 6;
double area = Area(base1, base2,
height);
cout << "Area is: " << area;
return 0;
}
|
C
#include <stdio.h>
double Area( int b1, int b2,
int h)
{
return ((b1 + b2) / 2) * h;
}
int main()
{
int base1 = 8, base2 = 10,
height = 6;
double area = Area(base1, base2,
height);
printf ( "Area is: %.1lf" , area);
return 0;
}
|
Java
import java.io.*;
class GFG
{
static double Area( int b1,
int b2,
int h)
{
return ((b1 + b2) / 2 ) * h;
}
public static void main (String[] args)
{
int base1 = 8 , base2 = 10 ,
height = 6 ;
double area = Area(base1, base2,
height);
System.out.println( "Area is: " + area);
}
}
|
Python3
def Area(b1, b2, h):
return ((b1 + b2) / 2 ) * h
base1 = 8 ; base2 = 10 ; height = 6
area = Area(base1, base2, height)
print ( "Area is:" , area)
|
C#
using System;
class GFG
{
static double Area( int b1,
int b2,
int h)
{
return ((b1 + b2) / 2) * h;
}
public static void Main ()
{
int base1 = 8, base2 = 10,
height = 6;
double area = Area(base1, base2,
height);
Console.WriteLine( "Area is: " + area);
}
}
|
PHP
<?php
function Area( $b1 , $b2 , $h )
{
return (( $b1 + $b2 ) / 2) * $h ;
}
$base1 = 8; $base2 = 10;
$height = 6;
$area = Area( $base1 , $base2 , $height );
echo ( "Area is: " );
echo ( $area );
?>
|
Javascript
<script>
function Area(b1, b2, h)
{
return ((b1 + b2) / 2) * h;
}
let base1 = 8, base2 = 10,
height = 6;
let area = Area(base1, base2,
height);
document.write( "Area is: " + area);
</script>
|
Output :
Area is: 54.0
Time complexity: O(1)
space complexity: O(1)
Share your thoughts in the comments
Please Login to comment...