Program to find Circumference of a Circle
Last Updated :
16 Feb, 2023
Given radius of a circle, write a program to find its circumference.
Examples :
Input : 2
Output : Circumference = 12.566
Input : 8
Output : Circumference = 50.264
In a circle, points lie in the boundary of a circle are at same distance from its center. This distance is called radius. Circumference of a circle can simply be evaluated using following formula.
Circumference = 2*pi*r
where r is the radius of circle
and value of pi = 3.1415.
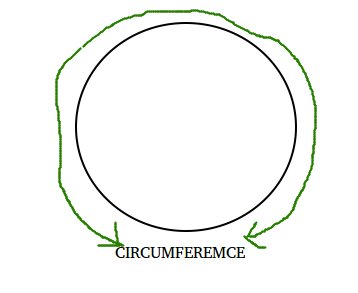
C++
#include<bits/stdc++.h>
using namespace std;
#define PI 3.1415
double circumference( double r)
{
double cir = 2*PI*r;
return cir;
}
int main()
{
double r = 5;
cout << "Circumference = "
<< circumference(r);
return 0;
}
|
Java
import java.io.*;
class Geometry {
static double circumference( double r){
double PI = 3.1415 ;
double cir = 2 *PI*r;
return cir;
}
public static void main (String[] args) {
double r = 5 ;
double result = Math.round(circumference(r) * 1000 ) / 1000.0 ;
System.out.println( "Circumference = " + result);
}
}
|
Python3
PI = 3.1415
def circumference(r):
return ( 2 * PI * r)
print ( '%.3f' % circumference( 5 ))
|
C#
using System;
class GFG {
static double circumference( double r){
double PI = 3.1415;
double cir = 2*PI*r;
return cir;
}
public static void Main () {
double r = 5;
double result =
Math.Round(circumference(r)
* 1000) / 1000.0;
Console.WriteLine( "Circumference = "
+ result);
}
}
|
PHP
<?php
$PI = 3.1415;
function circumference( $r )
{
global $PI ;
$cir = 2 * $PI * $r ;
return $cir ;
}
$r = 5;
echo "Circumference = " ,
circumference( $r );
?>
|
Javascript
<script>
function circumference(r)
{
let cir = 2*3.1415*r;
return cir;
}
let r = 5;
document.write( "Circumference = "
+ circumference(r));
</script>
|
Output :
Circumference = 31.415
Time Complexity: O(1), since there is no loop or recursion.
Auxiliary Space: O(1), since no extra space has been taken.
This article is contributed by Saloni Gupta .
Share your thoughts in the comments
Please Login to comment...