React Chakra UI Navigation
Last Updated :
19 Feb, 2024
React Chakra UI Navigation Bar is used in every website to make it more user-friendly so that the navigation through the website becomes easy and the user can directly search for the topic of their interest.
Prerequisites:
We will use the following approaches to implement Navigation Bar using React Chakra UI
Steps to Create React Application And Installing Module:
Step 1:Â Create a React application using the following command:
npx create-react-app gfg-navigation-app
Step 2:Â After creating your project folder(i.e. gfg-navigation-app), move to it by using the following command:
cd gfg-navigation-app
Step 3:Â After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
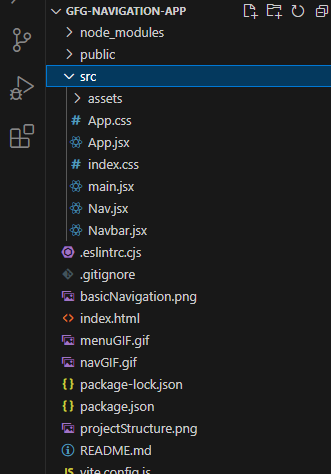
The updated dependencies in the package.json file:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0"
}
Approach 1: Navigation Bar Using Breadcrumb and LinkBox:
We created the basic box having navigation components like Breadcrumb and LinkBox from the Chakra UI library and placed it for handling a header menu bar with the usual menu items as “Home”, “About”, “Contact” and “Career”. A logo is added with an image icon and a href link is used for adding links to various navigated landing web pages. Access to other links is implemented using HTML href and Chakra UI BreadcrumbLink.
Example: Below example demonstrates the usage of first approach to create the navigation.
Javascript
import {
ChakraProvider,
Text
} from "@chakra-ui/react" ;
import Nav from "./Nav" ;
import "./App.css" ;
function App() {
return (
<ChakraProvider>
<Text
color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "600"
my= "1rem" >
GeeksforGeeks -
ReactJS Chakra UI concepts
</Text>
<Nav />
</ChakraProvider>
);
}
export default App;
|
Javascript
import { useState } from 'react'
import gfgLogo from './assets/gfg-new-logo.svg'
import {
Flex, Box,
Text,Image,Link,
Breadcrumb,
BreadcrumbItem,
BreadcrumbLink,
BreadcrumbSeparator,
} from "@chakra-ui/react" ;
const Nav = () => {
return (
<Box bg= "lightgrey" w= "100%" h= "90%" p={4}>
<Text as= "h2" fontWeight= "bold" >
Top Navigation Bar by Chakra UI
</Text>
<Flex mt= '3' justifyContent= "left" >
<Flex
direction= "column"
alignItems= "left"
w={{ base: "90%" , md: "90%" , lg: "100%" }}>
<Breadcrumb bg= "#BFD8AF" fontWeight= 'bold'
spacing= '4px' pt= '1' separator= ' ' >
<img bg= 'red' src={gfgLogo} className= "logo react"
alt= "gfg logo" />
</a>
<BreadcrumbItem>
<BreadcrumbLink color= 'blue'
Home
</BreadcrumbLink>
</BreadcrumbItem>
<BreadcrumbItem>
<Link color= 'blue'
href=
isExternal>
About
</Link>
</BreadcrumbItem>
<BreadcrumbItem>
<BreadcrumbLink color= 'blue' href=
Career
</BreadcrumbLink>
</BreadcrumbItem>
<BreadcrumbItem>
<BreadcrumbLink color= 'blue' href= '#' >
Contact
</BreadcrumbLink>
</BreadcrumbItem>
</Breadcrumb>
</Flex>
</Flex>
</Box>
);
};
export default Nav;
|
Step to Run the application:Â Run the application using the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
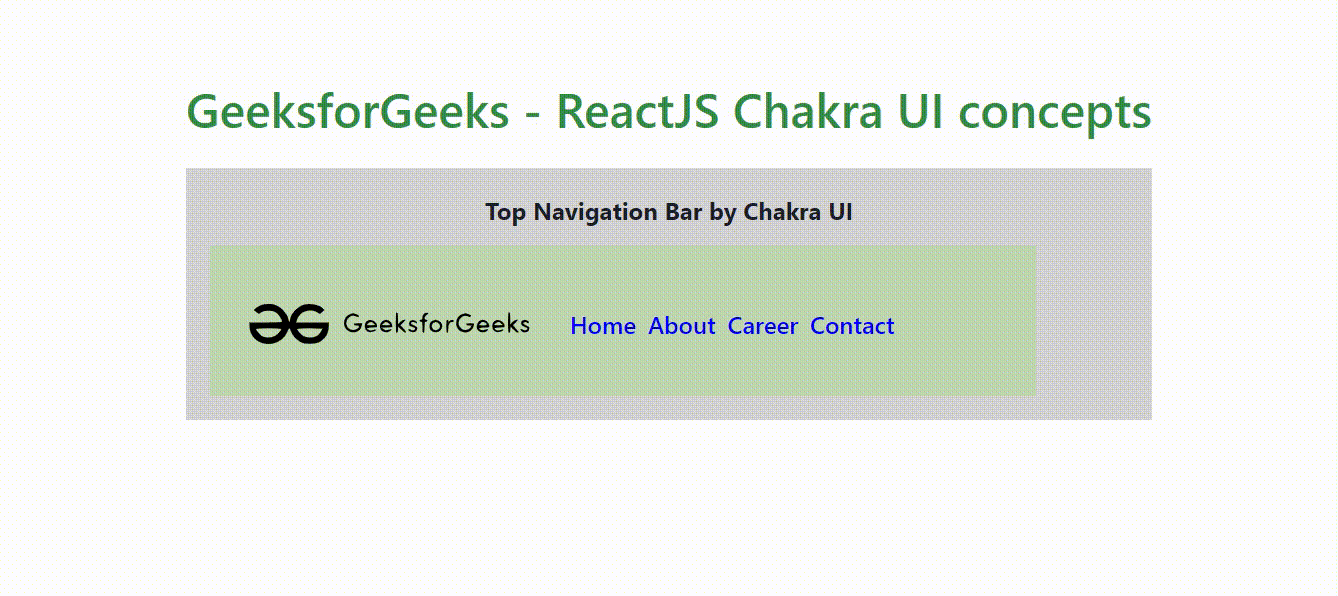
In this approach, we have used the menu components including MenuButton, MenuList, MenuItem, and MenuDivider of ChakraUI.
Example: Below example demonstrates the usage of menu component approach to create the navigation.
Javascript
import { useState } from 'react'
import gfgLogo
from './assets/gfg-new-logo.svg'
import {
Flex, Box,
Link, Button,
Menu,
MenuButton,
MenuList,
MenuItem,
MenuDivider,
} from "@chakra-ui/react" ;
const Nav = () => {
return (
<Box bg= "lightgrey"
w= "100%" h= "100%" p={4}>
<Flex mt= '3' justifyContent= "center" >
<Flex
direction= "row"
alignItems= "left"
w={{ base: "90%" , md: "70%" , lg: "50%" }}>
<Menu>
<MenuButton>
target= "_blank" >
<img src={gfgLogo}
className= "logo react"
alt= "gfg logo" />
</a>
</MenuButton>
<MenuList>
<MenuItem as= 'a'
Home
</MenuItem>
<MenuItem as= 'a' href= '#' >
About Us
</MenuItem>
<MenuItem as= 'a' href= '#' >
Contact Us
</MenuItem>
<MenuDivider />
<MenuItem as= 'a' href=
Careers
</MenuItem>
<MenuItem as= 'a' href=
Our Courses
</MenuItem>
</MenuList>
</Menu>
</Flex>
</Flex>
</Box>
);
};
export default Nav;
|
Steps to Run the App:
npm start
Output: Now go to http://localhost:3000 in your browser:
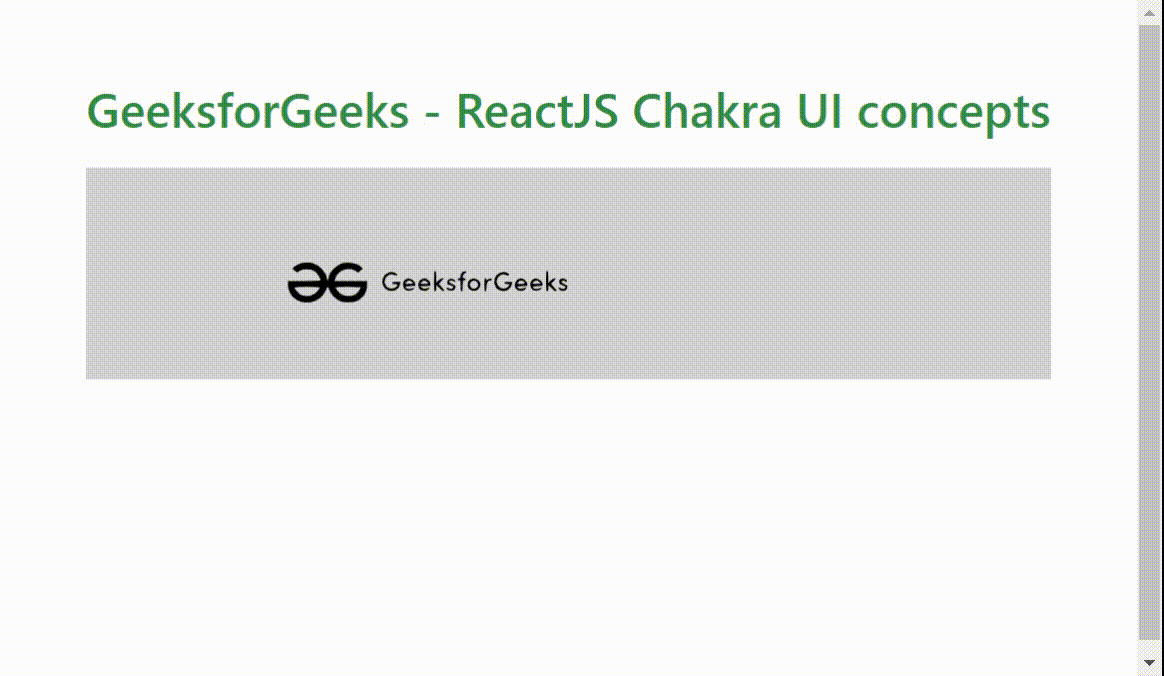
Share your thoughts in the comments
Please Login to comment...