React Chakra UI Typography Heading
Last Updated :
13 Feb, 2024
Chakra UI Typography Heading Component is used to display headings around the web application. Internally, the Heading Component renders HTML heading tags which can be customized using the as prop of the Heading Component.
The as prop take values h1, h2, h3, h4, h5 and h6. Additionally, we can change the size of the rendered heading using the size prop and provide the size value to it. The value of the size prop can be overridden by the fontSize prop.
Prerequisites:
Approach:
We will create Heading tags by passing different values of the as and the size props. We will also use the fontSize prop to change the size of the heading according to our needs.
Steps to Create React Application and Installing Chakra UI:
Step 1: Create a react application using the create-react-app.
npx create-react-app my-chakraui-app
Step 2: Move to the created project folder (my-chakraui-app).
cd my-chakraui-app
Step 3: After Creating the react app install the needed packages using below command
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
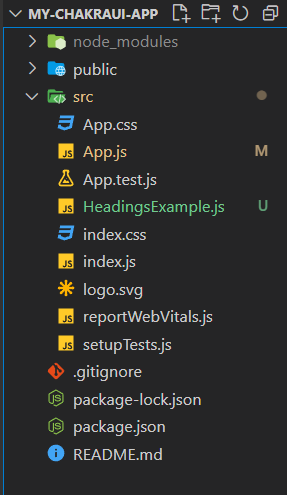
The updated dependencies in the package.json file are:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example:Â Below are the code snippets for the respective files.
Javascript
import { ChakraProvider, Text } from "@chakra-ui/react" ;
import "./App.css" ;
import HeadingsExample from "./HeadingsExample" ;
function App() {
return (
<ChakraProvider>
<Text
color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "600"
mt= "1rem"
>
GeeksforGeeks
</Text>
<Text
color= "#000"
fontSize= "1rem"
textAlign= "center"
fontWeight= "500"
mb= "2rem"
>
Chakra UI Typography Heading
</Text>
<HeadingsExample />
</ChakraProvider>
);
}
export default App;
|
Javascript
import React from 'react' ;
import { Box, Headingimport React from "react" ;
import { Box, Heading } from "@chakra-ui/react" ;
export default function HeadingExample() {
return (
<Box
display= "flex"
flexDirection= "column"
gap= "40px"
alignItems= "center"
>
<Heading as= "h1" size= "xl" >
Render H1 element with XL size
</Heading>
<Heading as= "h4" size= "sm" >
Render H4 element and have "sm" size
</Heading>
<Heading as= "h4" size= "sm" fontSize= "25px" >
fontSize value will override the size prop
</Heading>
<Heading as= "h4" size= "md" noOfLines={1} width= "380px" >
Only render 1 line. Add ellipses after that.
</Heading>
</Box>
);
}
} from '@chakra-ui/react' ;
export default function HeadingExample() {
return (
<Box
display= "flex"
flexDirection= "column"
gap= "40px"
alignItems= "center"
>
<Heading as= "h1" size= "xl" >
Render H1 element with XL size
</Heading>
<Heading as= "h4" size= "sm" >
Render H4 element and have "sm" size
</Heading>
<Heading as= "h4" size= "sm" fontSize= "25px" >
fontSize value will override the size prop
</Heading>
<Heading as= "h4" size= "md" noOfLines={1} width= "380px" >
Only render 1 line. Add ellipses after that.
</Heading>
</Box>
);
}
|
Start your application using the following command.
npm start
Output: Go to http://localhost:3000 in your browser to see the live app
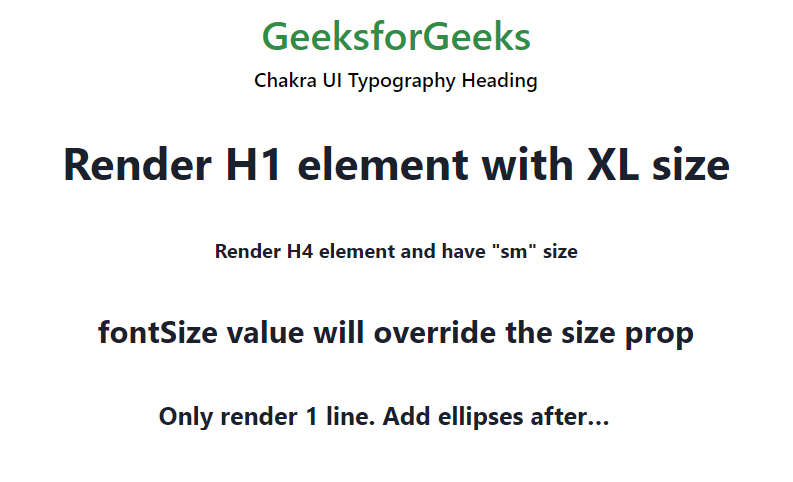
Share your thoughts in the comments
Please Login to comment...