Chakra UI Overlay Alert Dialog
Last Updated :
04 Feb, 2024
Chakra UI Overlay Alert Dialog component helps you bring user attention to critical actions or information. It creates a modal overlay, preventing interaction with the main interface until a decision is made. In the following situations we generally uses the Chakra UI Overlay Alert Dialog:
- Confirmation prompts: Ensure users understand the consequences of actions before they commit.
- Error messages: Clearly communicate issues requiring user attention.
- Success notifications: Celebrate achievements and provide feedback in a dedicated space.
With its customizable design and accessibility features, Chakra UI Overlay Alert Dialog empowers you to deliver clear, impactful messages and guide users towards informed decisions within your application.
Prerequisites:
Approach:
We created a basic “Delete” function to implement the delete functionality in websites and use Chakra UI Alert Dialog Component from the Chakra UI Library and implements the Chakra UI Overlay Alert Dialog using React.
Steps to Create the Project:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. my-app), move to it by using the following command:
cd gfg
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
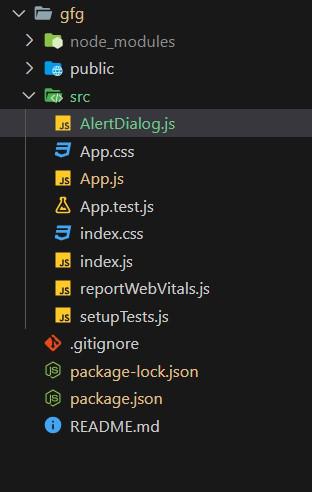
Project Structure
The updated dependencies in package.json will look like this:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^11.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Write down the code in respective files:
Javascript
import React from 'react' ;
import {
ChakraProvider,
CSSReset, Box, Container
}
from '@chakra-ui/react' ;
import AlertDialog from './AlertDialog' ;
function App() {
return (
<ChakraProvider>
<CSSReset />
<Container maxW= "container.sm" mt={10}>
<Box>
<AlertDialog />
</Box>
</Container>
</ChakraProvider>
);
}
export default App;
|
Javascript
import React, { useState, useRef } from "react" ;
import {
Button,
AlertDialog,
AlertDialogBody,
AlertDialogFooter,
AlertDialogHeader,
AlertDialogContent,
AlertDialogOverlay,
} from "@chakra-ui/react" ;
const DeleteButtonWithConfirmation = () => {
const [isOpen, setIsOpen] = useState( false );
const cancelRef = useRef();
const handleDelete = () => {
};
const onOpen = () => setIsOpen( true );
const onClose = () => setIsOpen( false );
return (
<>
<Button colorScheme= "red" onClick={onOpen}>
Delete
</Button>
<AlertDialog
isOpen={isOpen}
leastDestructiveRef={cancelRef}
onClose={onClose}
>
<AlertDialogOverlay>
<AlertDialogContent>
<AlertDialogHeader fontSize= "lg" fontWeight= "bold" >
Delete Item
</AlertDialogHeader>
<AlertDialogBody>
Are you sure you want to delete this item? This
action cannot be undone.
</AlertDialogBody>
<AlertDialogFooter>
<Button ref={cancelRef} onClick={onClose}>
Cancel
</Button>
<Button
colorScheme= "red"
onClick={handleDelete}
ml={3}
>
Delete
</Button>
</AlertDialogFooter>
</AlertDialogContent>
</AlertDialogOverlay>
</AlertDialog>
</>
);
};
export default DeleteButtonWithConfirmation;
|
Steps to run the application:
Step 1: Type the following command in terminal
npm start
Step 2: Open web-browser and type the following URL
http://localhost:3000/
Output:
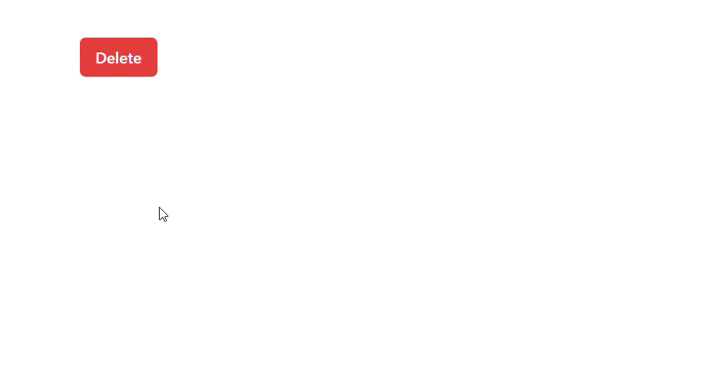
Output
Share your thoughts in the comments
Please Login to comment...