React Chakra UI Data Display
Last Updated :
19 Feb, 2024
React Chakra UIÂ Data Display is the component that can be embedded in the React application to display various data components. All these components are for data visualization purposes for an interactive and user-friendly interface. The various data components include Card, Badges, List, Table, Tag, Code, and many more data components.
Pre-requisites:
Approach:
We have created a basic box with data components like a Card having all the sub-components like Card header, Content, Card footer, Badges showing notifications, and Code components using Chakra UI Data Display components. We have taken some of the components as examples. Users can include as per their needs or requirements.
Steps To Create React Application And Installing Module:
Step 1:Â Create a React application using the following command:
npx create-react-app data-display-app
Step 2:Â After creating your project folder(i.e. data-display-app), move to it by using the following command:
cd data-display-app
Step 3:Â After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
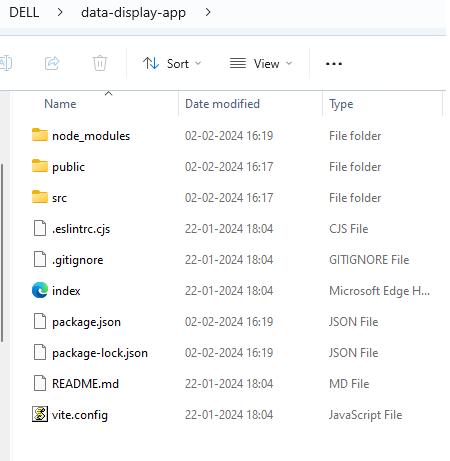
The updated dependencies are in the package.json file.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Write down the code in respective files.
Javascript
import { ChakraProvider, Text} from "@chakra-ui/react" ;
import Data from "./Data" ;
import "./App.css" ;
function App() {
return (
<ChakraProvider>
<Text color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "600"
my= "1rem" >
GeeksforGeeks - ReactJS Chakra UI concepts
</Text>
<Data />
</ChakraProvider>
);
}
export default App;
|
Javascript
import {
Flex, Box, Badge,Heading,
Stack,Text,Avatar,Button,
Card, CardHeader, CardBody, CardFooter,
Code,HStack,VStack, List,
ListItem,UnorderedList,
} from "@chakra-ui/react" ;
const Data = () => {
return (
<Box bg= "lightgrey" w= "100%" p={4}>
<Text as= "h2" fontWeight= "bold" mb= '4' >
Data Display by Chakra UI
</Text>
<Avatar src= '' />
<Text as= "i" fontSize= 'md' >
This is avatar
<Badge ml= '2' colorScheme= 'purple' >New</Badge>
</Text>
<Flex justifyContent= "center" >
<Flex direction= "column"
alignItems= "left"
w={{ base: "90%" , md: "70%" , lg: "50%" }}>
<Card mb= '4' >
<CardHeader>
<Heading size= 'sm' >This is card header</Heading>
</CardHeader>
<CardBody>
<Stack spacing= '2' >
<Box>
<Text pt= '2' fontSize= 'sm' >
This is the main content part to
be filled by the user.
<UnorderedList>
<ListItem>Solve DSA papers</ListItem>
<ListItem>
what is operations management</ListItem>
<ListItem>Use AI tool</ListItem>
</UnorderedList>
</Text>
</Box>
</Stack>
</CardBody>
<CardFooter>
<Text fontWeight= 'bold' >
This is card footer
</Text>
</CardFooter>
</Card>
<Stack direction= 'row' mb= '4' >
<Button>
<Text> Notifications
<Badge variant= 'solid' colorScheme= 'green' >10</Badge>
</Text>
</Button>
<Button>
<Text> Messages
<Badge variant= 'outline' colorScheme= 'red' >
14
</Badge>
</Text>
</Button>
</Stack>
<Stack direction= 'row' >
<Code children= 'console.log(welcome to ReactJS)' />
<Code colorScheme= 'green' children= 'npm run dev' />
</Stack>
</Flex>
</Flex>
</Box>
);
};
export default Data;
|
Data.js
Javascript
import {
Flex, Box, Badge,Heading,
Stack,Text,Avatar,Button,
Card, CardHeader, CardBody, CardFooter,
Code,HStack,VStack, List,
ListItem,UnorderedList,
} from "@chakra-ui/react" ;
const Data = () => {
return (
<Box bg= "lightgrey" w= "100%" p={4}>
<Text as= "h2" fontWeight= "bold" mb= '4' >
Data Display by Chakra UI
</Text>
<Avatar src= '' />
<Text as= "i" fontSize= 'md' >
This is avatar
<Badge ml= '2' colorScheme= 'purple' >New</Badge>
</Text>
<Flex justifyContent= "center" >
<Flex
direction= "column"
alignItems= "left"
w={{ base: "90%" , md: "70%" , lg: "50%" }}>
<Card mb= '4' >
<CardHeader>
<Heading size= 'sm' >This is card header</Heading>
</CardHeader>
<CardBody>
<Stack spacing= '2' >
<Box>
<Text pt= '2' fontSize= 'sm' >
This is the main content part to
be filled by the user.
<UnorderedList>
<ListItem>Solve DSA papers</ListItem>
<ListItem>what is operations management</ListItem>
<ListItem>Use AI tool</ListItem>
</UnorderedList>
</Text>
</Box>
</Stack>
</CardBody>
<CardFooter>
<Text fontWeight= 'bold' >
This is card footer
</Text>
</CardFooter>
</Card>
<Stack direction= 'row' mb= '4' >
<Button>
<Text> Notifications <Badge variant= 'solid'
colorScheme= 'green' >10</Badge>
</Text>
</Button>
<Button>
<Text> Messages
<Badge variant= 'outline' colorScheme= 'red' >
14
</Badge>
</Text>
</Button>
</Stack>
<Stack direction= 'row' >
<Code children= 'console.log(welcome to ReactJS)' />
<Code colorScheme= 'green' children= 'npm run dev' />
</Stack>
</Flex>
</Flex>
</Box>
);
};
export default Data;
|
Step to run the application:Â Run the application using the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
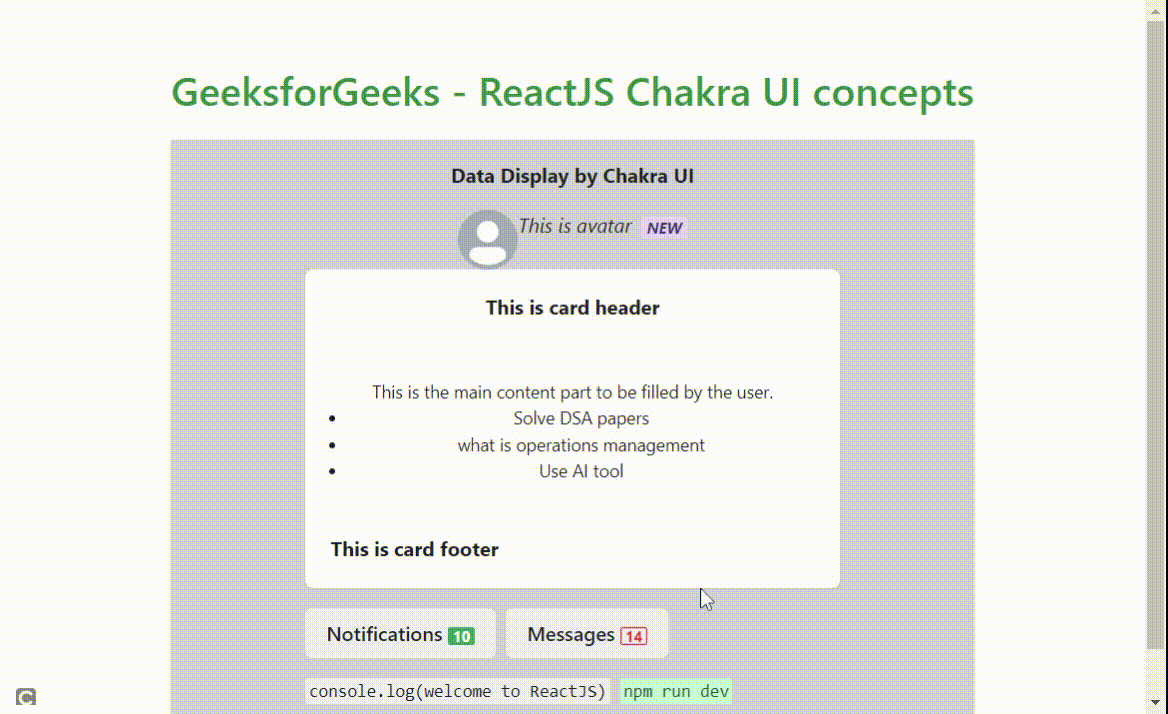
Output:
Share your thoughts in the comments
Please Login to comment...