How to pass data from child component to its parent in ReactJS ?
Last Updated :
23 Nov, 2023
In ReactJS, data flow between components is typically unidirectional, meaning data is passed from parent components to child components. However, there are scenarios where you may need to pass data from a child component back to its parent component.
Approach:
Following are the steps to pass data from the child component to the parent component:
- In the parent component, create a callback function. This callback function will retrieve the data from the child component.
- Pass the callback function to the child as a prop from the parent component.
- The child component calls the parent callback function using props and passes the data to the parent component.
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Project Structure: 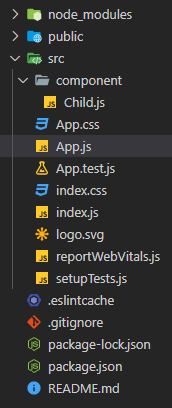
Example : This example uses the Child component prop to pass data from child component to parent component.
Javascript
import React from "react" ;
import Child from "./Child" ;
class App extends React.Component {
state = {
name: "" ,
};
handleCallback = (childData) => {
this .setState({ name: childData });
};
render() {
const { name } = this .state;
return (
<div>
<Child
parentCallback={ this .handleCallback}
/>
{name}
</div>
);
}
}
export default App;
|
Javascript
import React from "react" ;
class Child extends React.Component {
onTrigger = (event) => {
this .props.parentCallback(
event.target.myname.value
);
event.preventDefault();
};
render() {
return (
<div>
<form onSubmit={ this .onTrigger}>
<input
type= "text"
name= "myname"
placeholder= "Enter Name"
/>
<br></br>
<br></br>
<input type= "submit" value= "Submit" />
<br></br>
<br></br>
</form>
</div>
);
}
}
export default Child;
|
Step to run the application: Open the terminal and type the following command.
npm start
Output: Open the browser and our project is shown in the URL http://localhost:3000/
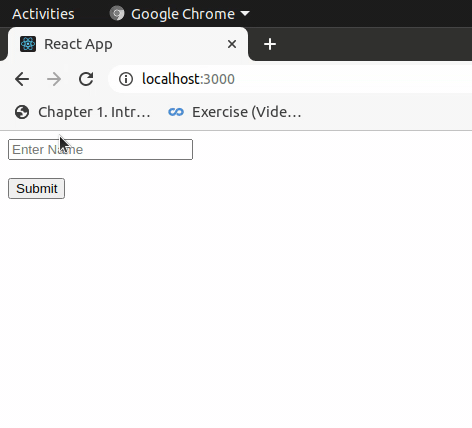
Share your thoughts in the comments
Please Login to comment...