How does React Router handle navigation in a React application?
Last Updated :
11 Mar, 2024
React Router is a standard library for routing in React. It enables the navigation among views of various components in a React Application, allows changing the browser URL, and keeps the UI in sync with the URL. Let us create a simple application to React to understand how the React Router works
React Router simplifies navigation in React applications by providing a declarative approach to define routes and their corresponding components using JSX syntax. It supports nested routing, route parameters, and programmatic navigation, that allows for dynamic and hierarchical navigation structures.
Approach to Handle navigation Using Link
Link is a component provided by React Router DOM that creates a hyperlink to navigate to a different route in your application. It’s typically used as a replacement for the <a> tag to navigate between different views in a React application without a page reload. When you click on a Link component, React Router DOM handles the navigation without a full page refresh.
Steps to create React App with React Router
Step 1: Create the react Application using the following command.
npx create-react-app navigation
Step 2: Now move into the project’s directory
cd navigation
Step 3: Installing React Router using the following command.
npm install react-router-dom
Folder Structure:
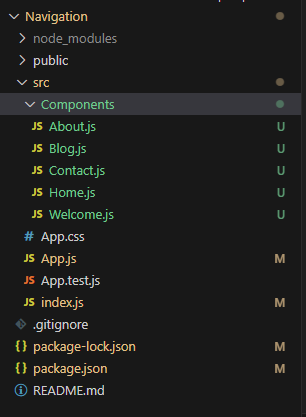
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Create the following folders s seen in folder structure and add the following codes.
Javascript
import {
BrowserRouter as Router,
Routes,
Route,
} from "react-router-dom" ;
import Home from './Components/Home' ;
import Contact from './Components/Contact' ;
import About from './Components/About' ;
import Welcome from './Components/Welcome' ;
import Blog from './Components/Blog' ;
function App() {
return (
<>
<Router>
<Welcome />
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "about" element={<About />} />
<Route path= "contact" element={<Contact />} />
<Route path= "blog" element={<Blog />} />
</Routes>
</Router>
</>
);
}
export default App;
|
Javascript
import React from 'react'
const Home = () => {
return (
<div style={{ color: "#00FFBF" }}>In <b>Home</b> page...</div>
)
}
export default Home
|
Javascript
import React from 'react'
const Contact = () => {
return (
<div style={{ color: "#62b6cb" }}> In <b>Contact</b> page...</div>
)
}
export default Contact
|
Javascript
import React from 'react'
const Blog = () => {
return (
<div style={{ color: "#a594f9" }}>In <b>Blog</b> page...</div>
)
}
export default Blog
|
Javascript
import React from 'react'
const About = () => {
return (
<div style={{ color: "#f72585" }}>In <b>About</b> page...</div>
)
}
export default About
|
Javascript
import React from 'react'
import { Link } from 'react-router-dom'
const Welcome = () => {
return (
<>
<h3>Welcome to GFG</h3>
<h5> React app: Navigation using Link</h5>
<ul>
<li><Link to= '/' >Home</Link></li>
<li><Link to= 'about' >About</Link></li>
<li><Link to= 'contact' >Contact</Link></li>
<li><Link to= 'blog' >Blog</Link></li>
</ul>
</>
)
}
export default Welcome
|
Output:
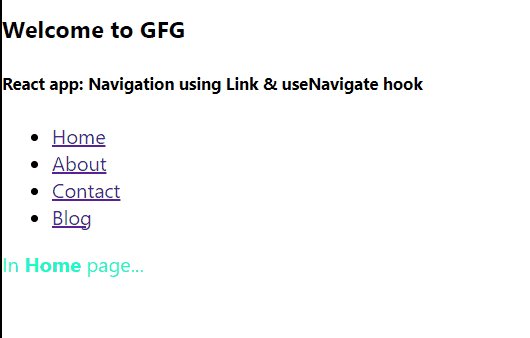
Share your thoughts in the comments
Please Login to comment...