Mastering React Routing: Learn Navigation and Routing in React Apps
Last Updated :
03 Nov, 2023
In this article, we will learn how to implement Routing in React in an efficient way by implementing various aspects of routing such as dynamic routing, programmatically routing, and other concepts.
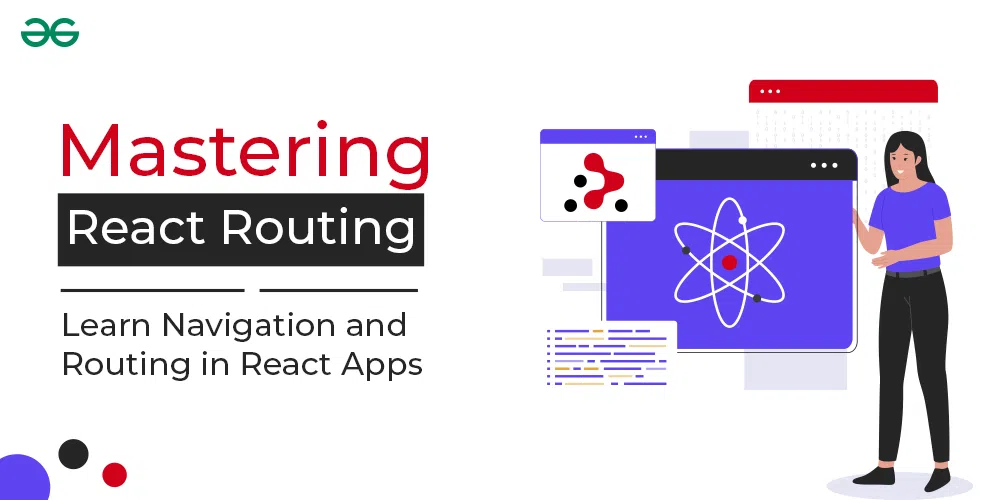
What is Navigation in React?
Navigation means browsing between various parts of the application. In React mostly SPA(Single Page Application) are developed so Navigation is complex. We do not load new pages from the server side each time a navigation link is pressed instead routing is implemented on the client side.
To implement routing in React we do not have in-built modules but instead, we use the react-router-dom module after installing and importing it.
Syntax:
// Installing
npm i react-router-dom@6
// Importing
import { BrowserRouter } from 'react-router-dom';
Routing with no page found(error 404) error handling
Sometimes user types a URL which does not exist in the website and the router fails and shows an error. To solve this problem we create a universal Route component which redirects to Link not found page whenever incorrect URL is passed
Approach:
- Create 3 basic pages where you want to add navigation
- Create a Navbar to handle navigation between the pages
- Create NoPageFound.js file to handle routing for all incorrect routing
- Add a path for non-configured routes which will be redirected to NoPageFound file
the path with ‘*‘ handles all non-configured routes
Example: Add the following code in respective files after removing default styling in App.css
- App.js: This file imports all the components and applies routing to them
- AboutUs.js: This file contains the About Us Page
- ContactUs.js: This file displays the Contact Us Page
- Home.js: This component acts as the home page
- Navbar.js: This component has the Navbar to redirect to all linking pages
- NoPageFound.js: This page is displayed when invalid links are added.
Javascript
import logo from "./logo.svg" ;
import { BrowserRouter, Routes, Route } from "react-router-dom" ;
import "./App.css" ;
import NavBar from "./components/Navbar" ;
import Home from "./components/Home" ;
import AboutUs from "./components/AboutUs" ;
import ContactUs from "./components/CotactUs" ;
import NoPageFound from "./components/NoPageFound" ;
function App() {
return (
<div className= "App" >
<BrowserRouter>
<NavBar />
<Routes>
<Route exact path= "/" element={<Home />} />
<Route exact path= "/about" element={<AboutUs />} />
<Route exact path= "/contact" element={<ContactUs />} />
<Route path= "*" element={<NoPageFound />} />
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
|
Javascript
import React from "react" ;
function AboutUs() {
return (
<div>
<h2>GeeksforGeeks is a computer science portal for geeks!</h2>
Read more about us at :
https:
</a>
</div>
);
}
export default AboutUs;
|
Javascript
import React from "react" ;
function ContactUs() {
return (
<address>
You can find us here:
<br />
GeeksforGeeks
<br />
5th & 6th Floor, Royal Kapsons, A- 118, <br />
Sector- 136, Noida, Uttar Pradesh (201305)
</address>
);
}
export default ContactUs;
|
Javascript
import React from "react" ;
function Home() {
return <h1>Welcome to the world of Geeks!</h1>;
}
export default Home;
|
Javascript
import { Link } from "react-router-dom" ;
export default function NavBar() {
return (
<div>
<ul className= "r" >
<li>
<Link to= "/" >Home</Link>
</li>
<li>
<Link to= "/about" >About Us</Link>
</li>
<li>
<Link to= "/contact" >Contact Us</Link>
</li>
</ul>
</div>
);
}
|
Javascript
export default function NoPageFound() {
return <h1>Error 404: Page Not Found</h1>;
}
|
Output:
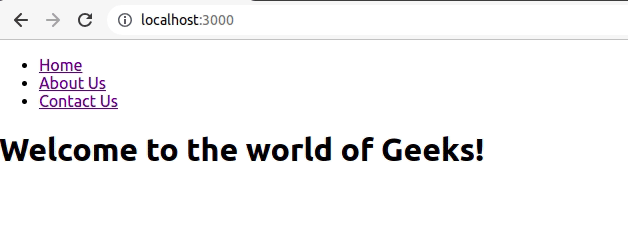
Programmatic Navigation in React
This type of routing is used when we want to redirect the user on the basis of an action such as button click
Approach:
- Create 2 basic pages between which you want to redirect
- Create buttons on each page to redirect the user
- Import the useNavigate hook provided with react-router-dom
- Use this hook on the onClick event of button to redirect
Example: Write the following code in respective files after removing default styling in App.css
- App.js: This file displays the AboutUs component
- AboutUs.js: This components creates the link to Contact Us page using Button
- ContactUs.js: This component has a button to send back to previous page
Javascript
import { BrowserRouter, Routes, Route } from "react-router-dom" ;
import "./App.css" ;
import AboutUs from "./components/AboutUs" ;
import ContactUs from "./components/CotactUs" ;
function App() {
return (
<div className= "App" >
<BrowserRouter>
<Routes>
<Route exact path= "/" element={<AboutUs />} />
<Route exact path= "/contactus" element={<ContactUs />} />
</Routes>
</BrowserRouter>
</div>
);
}
export default App;
|
Javascript
import React from "react" ;
import { useNavigate } from "react-router-dom" ;
function AboutUs() {
const nav = useNavigate();
return (
<div>
<h2>GeeksforGeeks is a computer science portal for geeks!</h2>
Read more about us at :
https:
</a>
<br></br>
<br></br>
<button
onClick={() => {
nav( "contactus" );
}}
>
Click Here to check contact details
</button>
</div>
);
}
export default AboutUs;
|
Javascript
import React from "react" ;
import { useNavigate } from "react-router-dom" ;
function ContactUs() {
const nav = useNavigate();
return (
<div>
<address>
You can find us here:
<br />
GeeksforGeeks
<br />
5th & 6th Floor, Royal Kapsons, A- 118, <br />
Sector- 136, Noida, Uttar Pradesh (201305)
</address>
<br></br>
<br></br>
<button
onClick={() => {
nav(-1);
}}
>
Click Here to Go Back
</button>
</div>
);
}
export default ContactUs;
|
Output:
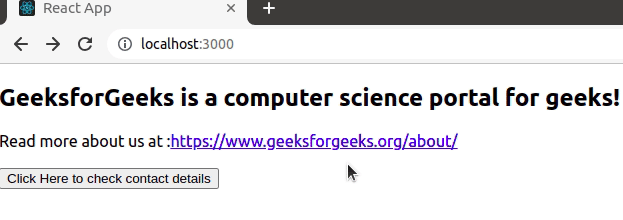
Dynamic Routing with React router
Adding Link routes gets very lengthy when there are multiple pages, so we use the concept of Dynamic Routing to reduce the lines of code and make the code shorter
Approach:
- Create a page which will create Link components using map
- Create a page which will display data dynamically using useParams
- Create a dynamic Route component which passes the id as a parameter
Example: Write the following code in respective files after removing default styling in App.css
- App.js: This file creates Link component dynamically and sends course name as a parameter
- CourseDetails.js: This file accesses the course as a parameter and passes it.
Javascript
import { BrowserRouter, Routes, Route, Link } from "react-router-dom" ;
import CourseDetails from "./components/CourseDetails" ;
function App() {
const courses = [ "JavaScript" , "React" , "HTML" , "DSA" ];
return (
<BrowserRouter>
<h1>Dynamic Routing with React</h1>
<ul>
{courses.map((course) => {
return (
<li key={course}>
<Link to={`courses/${course}`}>{course}</Link>
</li>
);
})}
</ul>
<Routes>
<Route path= "courses/:courseId" element={<CourseDetails />} />
</Routes>
</BrowserRouter>
);
}
export default App;
|
Javascript
import { useParams } from "react-router-dom" ;
function CourseDetails() {
const { courseId } = useParams();
return (
<div>
<h1>This is {courseId} course</h1>
</div>
);
}
export default CourseDetails;
|
Output:
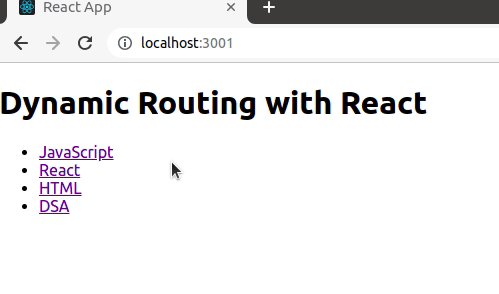
Share your thoughts in the comments
Please Login to comment...