How to redirect to another page in ReactJS ?
Last Updated :
31 Oct, 2023
Redirect to another page in React JS refers to navigating to different components in the single page react app using the react-router-dom package. To switch between multiple pages react-router-dom t enables you to implement dynamic routing in a web page.
Approach
Redirect will be implemented by using the react-router-dom package used to define routing in the react applications. It will redirect the user to the app component according to the defined paths and if the input path is not defined it will redirect the user to the home page.
Steps to create the React application
Step 1: Create a basic react app using the following command in your terminal.
npm create-react-app myreactapp
Step 2: Change your directory and enter your main folder
cd myreactapp
Step 3: Install react-router-dom for routing using this command
npm i react-router-dom
Project Structure
After creating components, the project structure will look like this
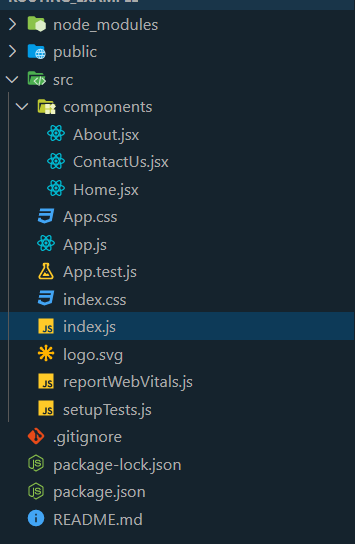
Folder structure after making components
After installing dependencies/package package.json file looks like this,
{
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-icons": "^4.11.0",
"react-router-dom": "^6.16.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
}
Example: Here, is the code of the App.js file where We are going to implement the react-router-dom package.
Javascript
import "./App.css" ;
import {
BrowserRouter as Router,
Routes,
Route,
Navigate,
} from "react-router-dom" ;
import Home from "./components/Home" ;
import About from "./components/About" ;
import ContactUs from "./components/ContactUs" ;
function App() {
return (
<>
{ }
<Router>
<Routes>
{
}
<Route
exact
path= "/"
element={<Home />}
/>
{
}
<Route
path= "/about"
element={<About />}
/>
{
}
<Route
path= "/contactus"
element={<ContactUs />}
/>
{
}
{ }
<Route
path= "*"
element={<Navigate to= "/" />}
/>
</Routes>
</Router>
</>
);
}
export default App;
|
Javascript
import React from "react" ;
import { Link } from "react-router-dom" ;
const Home = () => {
return (
<div>
<h1>Home Page</h1>
<br />
<ul>
<li>
{ }
<Link to= "/" >Home</Link>
</li>
<li>
{ }
<Link to= "/about" >About</Link>
</li>
<li>
{ }
<Link to= "/contactus" >Contact Us</Link>
</li>
</ul>
</div>
);
};
export default Home;
|
Javascript
import React from "react" ;
const About = () => {
return (
<div>
<h1>About Page</h1>
</div>
);
};
export default About;
|
Javascript
import React from "react" ;
const ContactUs = () => {
return (
<div>
<h1>Contact Us Page</h1>
</div>
);
};
export default ContactUs;
|
Step to run the application: Open the terminal and run the following command.
npm start
Output: This output will be visible on https://localhost:3000/ on browser
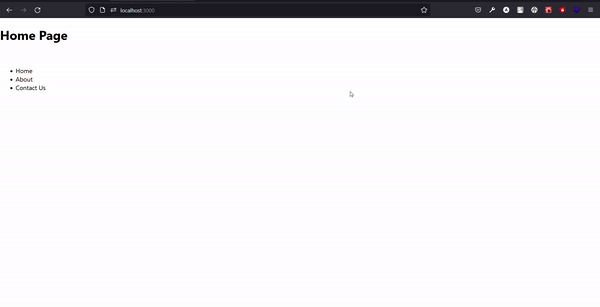
React-app output
Share your thoughts in the comments
Please Login to comment...