Chakra UI Navigation Stepper
Last Updated :
06 Feb, 2024
Chakra UI Navigation Stepper is a versatile and user-friendly component designed to facilitate smooth navigation within web applications and also used to indicate progress through a multi-step process. With a focus on simplicity and customization, Chakra UI Stepper seamlessly integrates into Chakra UI, a popular React component library. It empowers developers to create intuitive step-by-step navigation, enhancing user experience by providing clear progress indicators within complex processes or workflows.
Prerequisites:
Approach:
We will build different types of Navigation Steppers using Chakra UI with ReactJS. The Chakra UI Stepper component provides various functionalities such as Step, StepDescription, StepIcon, StepIndicator, StepNumber, StepSeparator, StepStatus, StepTitle allowing developers to customize the appearance and behavior of the stepper according to their requirements.
Steps to Create the Project:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. my-app), move to it by using the following command:
cd gfg
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
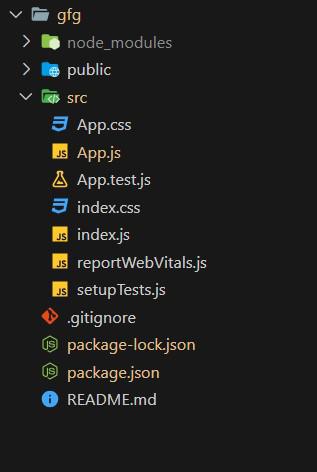
Project Structure
The updated dependencies in package.json will look like this:
"dependencies": {
"@chakra-ui/icons": "^2.1.1",
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^11.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the basic example of the Chakra UI Navigation Stepper:
Javascript
import { ChakraProvider, Box, Text }
from "@chakra-ui/react" ;
import {
Step,
StepDescription,
StepIcon,
StepIndicator,
StepNumber,
StepSeparator,
StepStatus,
StepTitle,
Stepper,
useSteps,
} from '@chakra-ui/react' ;
const steps = [
{ title: 'First' , description: 'Contact Info' },
{ title: 'Second' , description: 'Date & Time' },
{ title: 'Third' , description: 'Select Rooms' },
]
function App() {
const { activeStep } = useSteps({
index: 1,
count: steps.length,
})
return (
<ChakraProvider>
<Box
w= "55%"
mx= "auto"
>
<Text
color= "#2F8D46"
fontSize= "1.4rem"
textAlign= "center"
fontWeight= "400"
my= "1rem"
mt= "2rem"
>
Horizontal Navigation Stepper
</Text>
<Stepper index={activeStep}>
{steps.map((step, index) => (
<Step key={index}>
<StepIndicator>
<StepStatus
complete={<StepIcon />}
incomplete={<StepNumber />}
active={<StepNumber />}
/>
</StepIndicator>
<Box flexShrink= '0' >
<StepTitle>{step.title}</StepTitle>
<StepDescription>
{step.description}
</StepDescription>
</Box>
<StepSeparator />
</Step>
))}
</Stepper>
<Text
color= "#2F8D46"
fontSize= "1.4rem"
textAlign= "center"
fontWeight= "400"
my= "1rem"
mt= "2rem"
>
Vertical Navigation Stepper
</Text>
<Stepper index={activeStep}
orientation= 'vertical'
height= '400px' gap= '0' >
{steps.map((step, index) => (
<Step key={index}>
<StepIndicator>
<StepStatus
complete={<StepIcon />}
incomplete={<StepNumber />}
active={<StepNumber />}
/>
</StepIndicator>
<Box flexShrink= '0' >
<StepTitle>{step.title}</StepTitle>
<StepDescription>
{step.description}
</StepDescription>
</Box>
<StepSeparator />
</Step>
))}
</Stepper>
</Box>
</ChakraProvider>
)
}
export default App;
|
Steps to run the application:
npm start
Output:
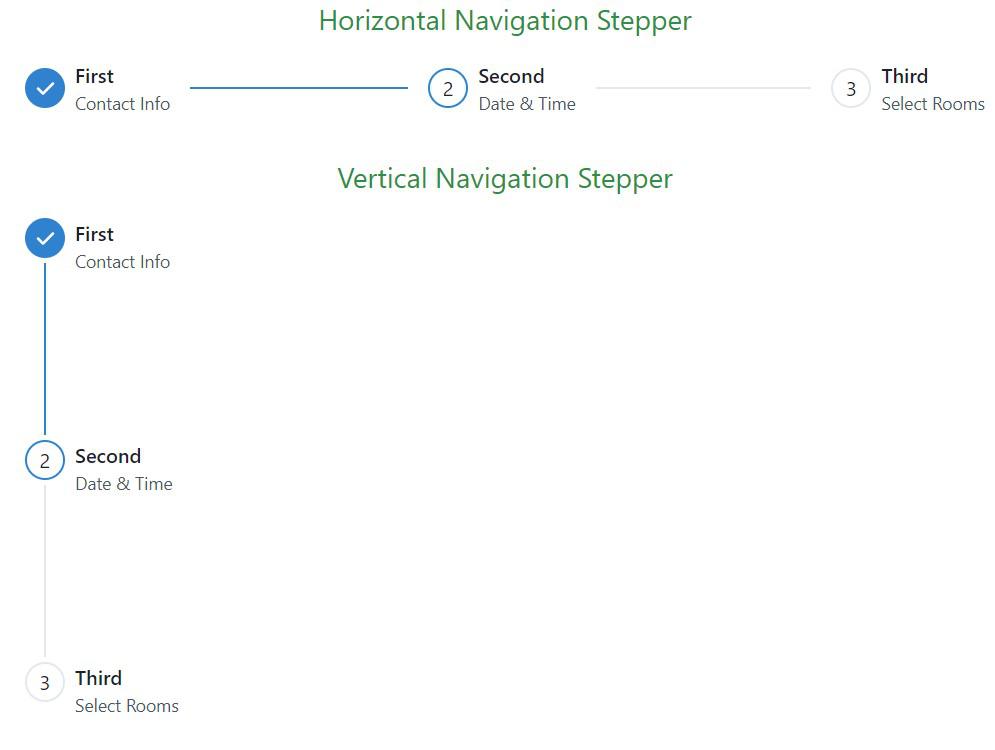
Chakra UI Navigation Stepper
Share your thoughts in the comments
Please Login to comment...