React Chakra UI Position
Last Updated :
19 Feb, 2024
The Chakra UI library is a popular React UI library that helps build advanced and customizable user interfaces. One essential aspect of building UI is positioning the elements at the right positions on the screen.
Prerequisites:
Chakra UI Position props
- position: specify the type of positioning
- zIndex: specify the z-index of the element
- top: specify the space from the top of the relative ancestor/viewport
- right: specify the space from the right of the relative ancestor/viewport
- bottom: specify the space from the bottom of the relative ancestor/viewport
- left: specify the space from the bottom of the relative ancestor/viewport
This article will explain various approaches to position elements using Chakra UI.
Approach 1: Absolute Positioning
Absolute positioning helps you position an element relative to its closest parent or the document itself. The element does not affect the positions of other elements.
Syntax:
<Box position="absolute" top="50%" left="50%">
{ /* Your content here */ }
</Box>
Approach 2: Fixed Positioning
Fixed positioning positions the element relative to the screen viewport. The elements remains fixed in its position even if the page is scrolled.
Syntax:
<Box position="fixed" top="0" left="0">
{ /* Your content will come here */ }
</Box>
Approach 3: Relative positioning
Relative positioning is used as a reference to its absolute children elements. Relative positioning positions the elements at its normal position.
Syntax:
<Box position="relative">
{ /* Your element at absolute positioning */ }
</Box>
Approach 4: Sticky Positioning
Sticky positioning lies somewhere between relative and fixed positioning. The element behaves as relative until a certain point, after which it becomes fixed in position.
Syntax:
<Box position="sticky" top="0">
{ /* Your content will come here */ }
</Box>
Steps to Create a React App and Installing Module
Step 1: Create a new react app and enter into it by running the following commands:
npx create-react-app my-app
cd my-app
Step 2: Install the dependencies `@chakra-ui/react` by running the following command into the terminal:
npm i @chakra-ui/react
Project Structure:
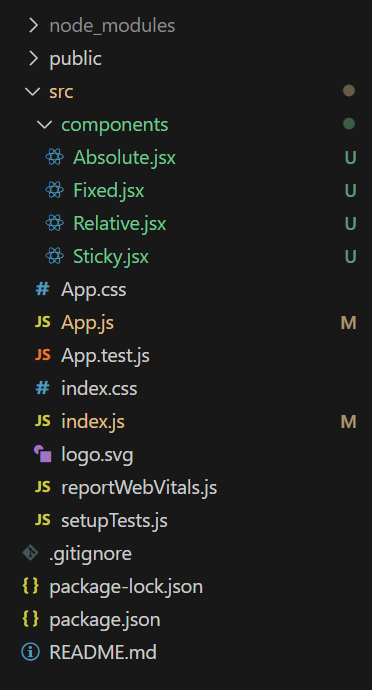
Project Structure
The updated dependencies in package.json file will look like.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Here is the implementation for the Chakra UI position.
Javascript
import { Box } from "@chakra-ui/react" ;
const AbsolutePositioningExample = () => {
return (
<Box bg= 'pink' position= "relative" w= '100%' height= "200px" >
<Box p= '24px' color= 'white' position= "absolute"
top= '30%' left= '35%' backgroundColor= "#ff5542"
borderRadius= "sm" boxShadow= "lg" >
I am absolute positioned WRT Light Pink box at Top: 30% and Left: 30%
</Box>
</Box>
);
};
export default AbsolutePositioningExample;
|
Javascript
import { Box } from "@chakra-ui/react" ;
const FixedPositioningExample = () => {
return (
<Box borderRadius= 'sm' w={ 'full' } p= '24px' color= 'white'
position= "fixed" bottom= "0" backgroundColor= "#4242ed" >
I am a Footer Fixed Positioning at Bottom: 0
</Box>
);
};
export default FixedPositioningExample;
|
Javascript
import { Box, Heading } from "@chakra-ui/react" ;
const RelativePositioningExample = () => {
return (
<Box mt={12} bg= 'gray' position= "relative" w= '100%'
height= "200px" p= '12px' >
<Heading fontSize={ 'md' } color={ 'white' }>
I am Relative reference to the green absolute box
</Heading>
<Box color= 'white' p= '24px' borderRadius= 'sm'
position= "absolute" top= '30%' left= "35%" backgroundColor= "green" >
I am absolute WRT my Grey box Reference at Top: 30% and Left: 30%
</Box>
</Box>
);
};
export default RelativePositioningExample;
|
Javascript
import React from 'react' ;
import { Box } from '@chakra-ui/react' ;
const Sticky = () => {
return (
<Box
position= "sticky"
backgroundColor= "orange"
width= 'full'
boxShadow= "md"
textAlign= "center"
lineHeight= "80px"
>
This is a Sticky Navbar
</Box>
);
};
export default Sticky;
|
Javascript
import logo from './logo.svg' ;
import './App.css' ;
import AbsolutePositioningExample from './components/Absolute' ;
import FixedPositioningExample from './components/Fixed' ;
import RelativePositioningExample from './components/Relative' ;
import Sticky from './components/Sticky' ;
function App() {
return (
<div className= "App" style={{
margin: 'auto' ,
width: '100%'
}}>
<Sticky/>
<RelativePositioningExample/>
<FixedPositioningExample/>
<AbsolutePositioningExample/>
</div>
);
}
export default App;
|
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom/client' ;
import './index.css' ;
import App from './App' ;
import { ChakraProvider } from '@chakra-ui/react' ;
const root = ReactDOM.createRoot(document.getElementById( 'root' ));
root.render(
<ChakraProvider>
<App />
</ChakraProvider>
);
|
Output:
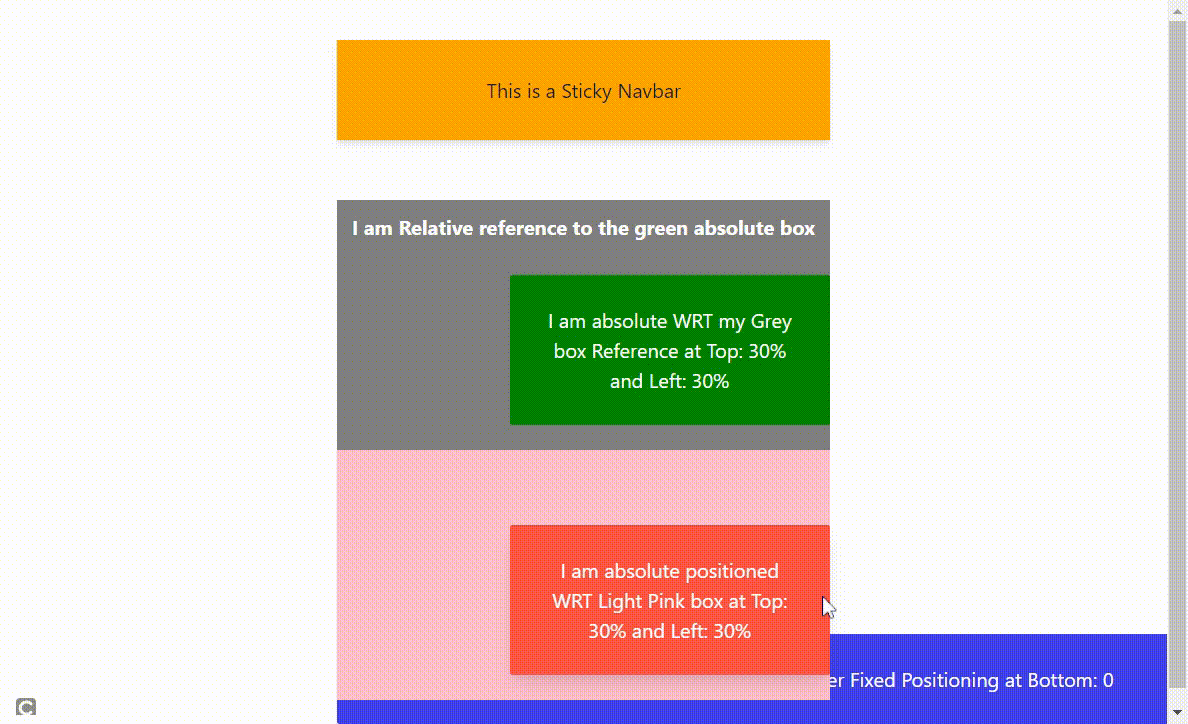
Share your thoughts in the comments
Please Login to comment...