How to add table footers with react-bootstrap-table?
Last Updated :
29 Nov, 2023
In this article we are going to learn about table footers with react-bootstrap-table.Table footers in React-Bootstrap-Table enable custom content at the bottom of a table. They’re created either by defining footer content within column definitions or by rendering a separate footer element outside the table, allowing for summary information or custom content below the table structure.
Prerequisites
Steps to create the project and install dependencies:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd foldername
Step 3: After creating the ReactJS application, Install the required module using the following command:
npm install react-bootstrap bootstrap react-bootstrap-table-next
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.1",
"react-bootstrap-table-next": "^4.0.3",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Project structure:
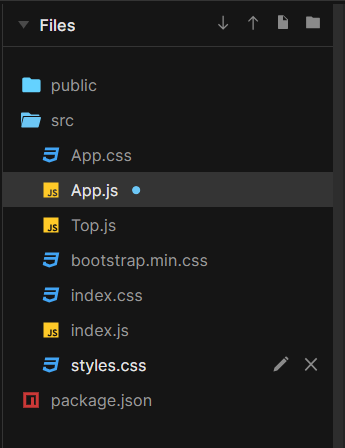
In this approach we are using the “footer” property within each column definition to set specific footers. Additionally, it employs the “footer” prop in “BootstrapTable” to establish an overall table footer, ensuring both column-specific and table-wide footer content.
In this approach we are using react-bootstrap-table-next to create the table. It employs the library’s features, like column-specific “footer” property in the column configuration and the “footer” prop within “BootstrapTable” to define table footers efficiently, showcasing column-specific and overall table footer content.
Example: Implementation of above approach.
Javascript
import React from 'react' ;
import BootstrapTable from 'react-bootstrap-table-next' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
const data = [
{
No: 1,
Course: 'HTML- Basics' ,
Price: '$29' ,
},
{
No: 2,
Course: 'CSS- Basics' ,
Price: '$25' ,
},
{
No: 3,
Course: 'JS- Basics' ,
Price: '$35' ,
},
];
const App = () => {
const columns = [
{
dataField: 'No' ,
text: 'No' ,
footer: 'Combo' ,
},
{
dataField: 'Course' ,
text: 'Course' ,
footer: 'Front-End Developer' ,
},
{
dataField: 'Price' ,
text: 'Price' ,
footer: '$89' ,
},
];
return (
<>
<h1 className= "text-success text-center" >GeeksforGeeks</h1>
<BootstrapTable keyField= 'No'
data={data}
columns={columns}
footerClasses= 'table-dark'
footer={() => (
<tfoot className= "table-dark" >
<tr>
<td>Combo</td>
<td>Front-End Developer</td>
<td>$89</td>
</tr>
</tfoot>
)}/>
</>)
};
export default App;
|
Output:
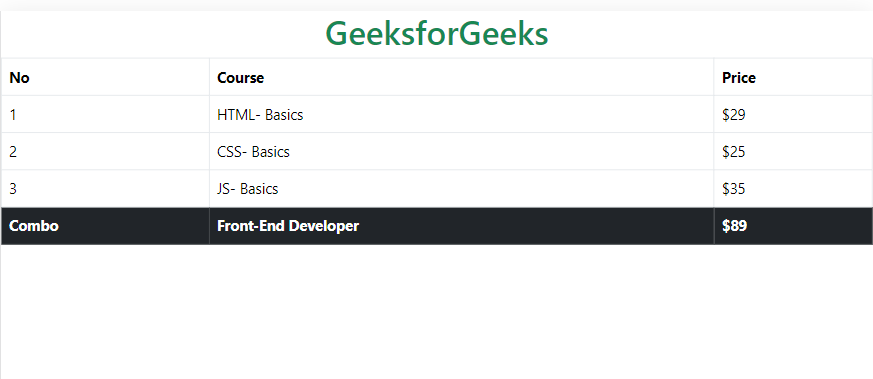
In this approach involves creating a table with React Bootstrap Table, manually crafting a footer row, and populating it with customized data (e.g., IDs, averages). It utilizes React to map data for table rows and directly inserts custom data for the footer row, providing control over its content and appearance.
Example: Implementation of above approach.
Javascript
import React from 'react' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
const data = [
{
id: 1,
name: 'Rahul' ,
age: 25,
},
{
id: 2,
name: 'Rakesh' ,
age: 28,
},
{
id: 3,
name: 'Ritesh' ,
age: 30,
},
];
const columns = [
{
dataField: 'id' ,
text: 'ID' ,
},
{
dataField: 'name' ,
text: 'Name' ,
},
{
dataField: 'age' ,
text: 'Age' ,
},
];
const App = () => {
const footerData = {
id: 'result' ,
name: 'Age average' ,
age: '27.66' ,
};
return (
<div className= "table-responsive" >
<table className= "table" >
<thead>
<tr>
{columns.map((column, index) => (
<th key={index}>{column.text}</th>
))}
</tr>
</thead>
<tbody>
{data.map((row, rowIndex) => (
<tr key={row.id}>
{Object.values(row).map((value, columnIndex) => (
<td key={columnIndex}>{value}</td>
))}
</tr>
))}
</tbody>
<tfoot>
<tr className= "table-dark" >
{Object.values(footerData).map((value, index) => (
<td key={index}>{value}</td>
))}
</tr>
</tfoot>
</table>
</div>
);
};
export default App;
|
Output:
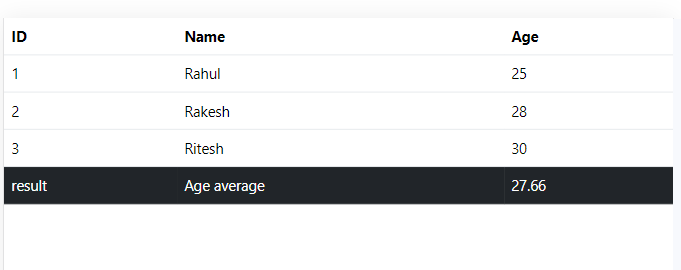
Share your thoughts in the comments
Please Login to comment...