Socket Programming in C++
Last Updated :
31 Dec, 2023
In C++, socket programming refers to the method of communication between two sockets on the network using a C++ program. We use the socket API to create a connection between the two programs running on the network, one of which receives the data by listening to the particular address port, and the other sends the data. One of the features of the socket programming is that it allows the bidirectional communication between the nodes.
In this article, we will create some simple C++ programs to demonstrate the use of socket programming.
What are Sockets?
Sockets can be viewed as the endpoint of the two-way communication between the two programming in the network. They are generally hosted on different application ports and allow bidirectional data transfer.
Types of Sockets
There are two main types of sockets:
- Stream Sockets: Stream sockets (like TCP) are like making a reliable phone call, ensuring that all information is delivered correctly.
- Datagram Sockets: Datagram sockets (like UDP) are more like sending letters. they’re faster but might get lost.
Server Stages
1. Creating the Server Socket
We create socket by using the socket() system call. It is defined inside the <sys/socket.h> header file.
Syntax
int serverSocket = socket(AF_INET, SOCK_STREAM, 0);
here,
- Â socketfd: It is the file descriptor for the socket.
- AF_INET: It specifies the IPv4 protocol family.
- SOCK_STREAM: It defines that the TCP type socket.
2. Defining Server Address
We then define the server address using the following set of statements
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(8080);
serverAddress.sin_addr.s_addr = INADDR_ANY;
here,
- sockaddr_in: It is the data type that is used to store the address of the socket.
- htons(): This function is used to convert the unsigned int from machine byte order to network byte order.
- INADDR_ANY: It is used when we don’t want to bind our socket to any particular IP and instead make it listen to all the available IPs.
3. Binding the Server Socket
Then we bind the socket using the bind() call as shown.
bind(serverSocket, (struct sockaddr*)&serverAddress, sizeof(serverAddress));
4. Listening for Connections
We then tell the application to listen to the socket refffered by the serverSocket.
listen(serverSocket, 5);
5. Accepting a Client Connection
The accept() call is used to accept the connection request that is recieved on the socket the application was listening to.
int clientSocket = accept(serverSocket, nullptr, nullptr);
6. Receiving Data from the Client
Then we start receiving the data from the client. We can specify the required buffer size so that it has enough space to receive the data sent the the client. The example of this is shown below.
char buffer[1024] = {0};
recv(clientSocket, buffer, sizeof(buffer), 0);
cout << "Message from client: " << buffer << endl;
7. Closing the Server Socket
We close the socket using the close() call and the associated socket descriptor.
close(serverSocket);
Client Stages
Similar to server, we also have to create a socket and specify the address. But instead of accepting request, we send the connection request when we can to sent the data using connect() call.
Then we sent the data using send() call. After all the operations are done, we close the connection using close() call.
1. Creating the Client Socket
int clientSocket = socket(AF_INET, SOCK_STREAM, 0);
2. Defining Server Address
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(8080);
serverAddress.sin_addr.s_addr = INADDR_ANY;
3. Connecting to the Server
connect(clientSocket, (struct sockaddr*)&serverAddress, sizeof(serverAddress));
4. Sending Data to the Server
const char* message = "Hello, server!";
send(clientSocket, message, strlen(message), 0);
5. Closing the Client Socket
close(clientSocket);
Example of Socket Programming in C++
server.cpp
C++
#include <cstring>
#include <iostream>
#include <netinet/in.h>
#include <sys/socket.h>
#include <unistd.h>
using namespace std;
int main()
{
int serverSocket = socket(AF_INET, SOCK_STREAM, 0);
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(8080);
serverAddress.sin_addr.s_addr = INADDR_ANY;
bind(serverSocket, ( struct sockaddr*)&serverAddress,
sizeof (serverAddress));
listen(serverSocket, 5);
int clientSocket
= accept(serverSocket, nullptr, nullptr);
char buffer[1024] = { 0 };
recv(clientSocket, buffer, sizeof (buffer), 0);
cout << "Message from client: " << buffer
<< endl;
close(serverSocket);
return 0;
}
|
client.cpp
C++
#include <cstring>
#include <iostream>
#include <netinet/in.h>
#include <sys/socket.h>
#include <unistd.h>
int main()
{
int clientSocket = socket(AF_INET, SOCK_STREAM, 0);
sockaddr_in serverAddress;
serverAddress.sin_family = AF_INET;
serverAddress.sin_port = htons(8080);
serverAddress.sin_addr.s_addr = INADDR_ANY;
connect(clientSocket, ( struct sockaddr*)&serverAddress,
sizeof (serverAddress));
const char * message = "Hello, server!" ;
send(clientSocket, message, strlen (message), 0);
close(clientSocket);
return 0;
}
|
By compiling and running server and client source files, we get the following output.
Output
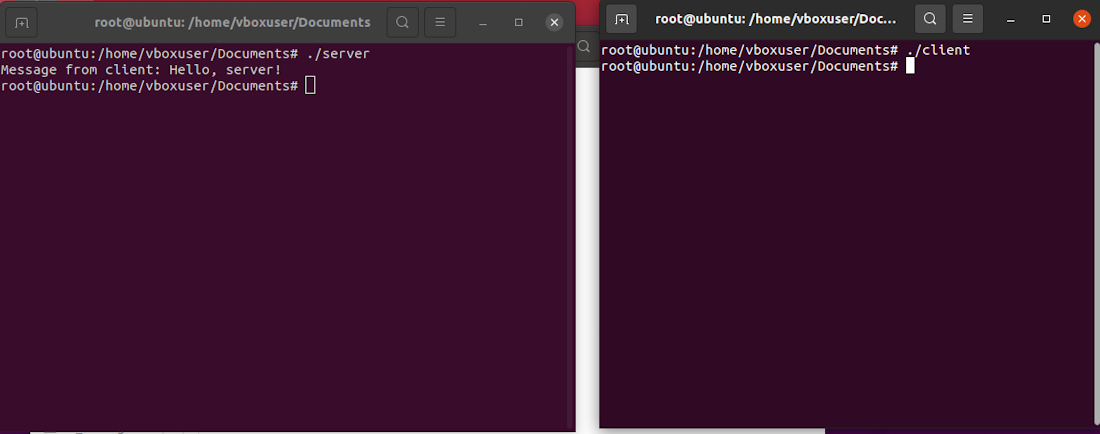
As we can see, the message we sent to the server at port 8080 is revived and printed by the server. We can also create a loop where we can keep sending the messages to the server.
Application and Benefits
Real-world Applications
Socket programming finds applications in a myriad of scenarios, from fundamental client-server interactions to intricate distributed systems. Its versatility powers web servers, chat applications, file transfer protocols and online gaming platforms.
Advantages of Socket Programming
Talking in Different Languages: Sockets help programs written in different languages understand each other.
Fast and Efficient Communication: They enable direct communication, making data exchange quicker.
Handling Many Users: Sockets let applications handle many users at the same time, like a busy chat room.
Share your thoughts in the comments
Please Login to comment...