React Bootstrap Stacks
Last Updated :
14 Mar, 2024
React Bootstrap Stacks are a type of layout in React Bootstrap that follows the style of a stack arranging elements one over another or one after another. It is a helper utility built on top of the existing flexbox layout to make it easier to create component-based layouts.
Syntax:
import { Stack } from "react-bootstrap";
<Stack ...>
...
</Stack>
Stacks API properties
Name
| Type
| Default
| Description
|
---|
bsPrefix
| string
| “hstack | vstack”
| Changes the underlying component base class name, defaults to hstack if the direction is horizontal, vstack incase of vertical.
|
direction
| string
| “vertical”
| Modifies the direction of stack, defaults to vertical also supports horizontal layout.
|
gap
| custom
|
| Configures the gap between the elements of stack.
|
as
|
| <div>
| Changes the underlying HTML tag, defaults to use div tag.
|
Steps to use React Bootstrap Stack
Step 1: Initialize a React Project. Check this post for setting up react project
Step 2: Install the react-bootstrap and bootstrap dependencies using the below npm command.
npm install react-bootstrap bootstrap
Step 3: Import the bootstrap css file in the index.js to use the css in every components.
import "bootstrap/dist/css/bootstrap.css";
Step 4: Add the following codes to public/index.html to use Bootstrap in the application.
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css"
integrity="sha384-9ndCyUaIbzAi2FUVXJi0CjmCapSmO7SnpJef0486qhLnuZ2cdeRhO02iuK6FUUVM"
crossorigin="anonymous"
/>
<script src="https://cdn.jsdelivr.net/npm/react/umd/react.production.min.js" crossorigin></script>
<script
src="https://cdn.jsdelivr.net/npm/react-dom/umd/react-dom.production.min.js"
crossorigin></script>
<script
src="https://cdn.jsdelivr.net/npm/react-bootstrap@next/dist/react-bootstrap.min.js"
crossorigin>
</script>
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.2",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
React Bootstrap Stacks Examples
Example 1: This example implements the following approach,
- In this approach, we will use Vertical Stack layout, every element will be aligned vertically and will utilize full width by default. It is the default alignment of React Bootstrap stacks.
- Open the App.js file and write the below code to render a vertical stack using react bootstrap.
- Inside the Stack component, insert your HTML elements, the stack component will display the elements vertically.
- Specify the gap value to separate the elements ranging from 1 to 5.
Javascript
// App.js
import "./App.css";
import { Stack } from "react-bootstrap";
function App() {
return (
<div>
<h1>React Bootstrap Stacks</h1>
<h3>Vertical Stack</h3>
<hr />
<Stack gap={3}>
<div className="p-2 h4 bg-info">Stack Item 1</div>
<div className="p-2 h4 bg-info">Stack Item 2</div>
<div className="p-2 h4 bg-info">Stack Item 3</div>
<div className="p-2 h4 bg-info">Stack Item 4</div>
</Stack>
</div>
);
}
export default App;
Output:
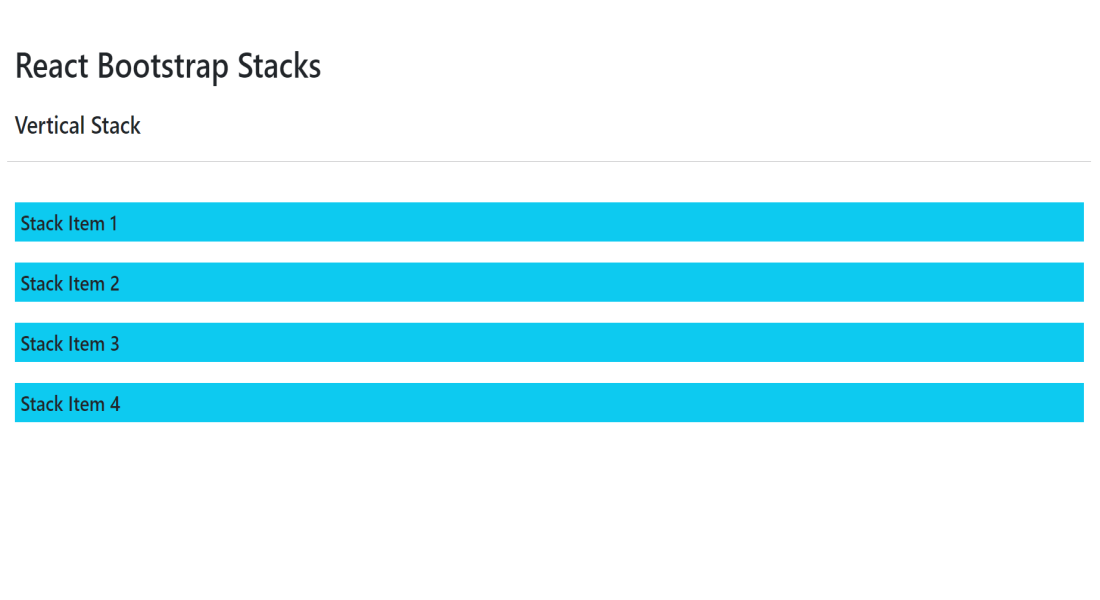
Example 1 output
Example 2: This example will implement the following approach:
- In this, we will use the horizontal layout of Stack to display the elements in the horizontal fashion.
- For this we will utilize the direction property of Stack Component and pass the horizontal argument for the Component.
- Open the App.js file and write the below code to render a vertical stack using react bootstrap.
- Inside the Stack component, insert your HTML elements, the stack component will display the elements, pass the horizontal value to the string to display the elements horizontally.
- Specify the gap value to separate the elements ranging from 1 to 5.
- Add some vertical lines to visualize the separation of the HTML elements.
Javascript
// App.js
import "./App.css";
import { Stack } from "react-bootstrap";
function App() {
return (
<div>
<h1>React Bootstrap Stacks</h1>
<h3>Horizontal Stack</h3>
<hr />
<Stack direction="horizontal" gap={3}>
<div className="p-2 h4 bg-primary">Stack Item 1</div>
<div className="p-2 h4 bg-primary">Stack Item 2</div>
<div className="p-2 h4 bg-primary">Stack Item 3</div>
<div className="p-2 h4 bg-primary">Stack Item 4</div>
</Stack>
</div>
);
}
export default App;
Output:
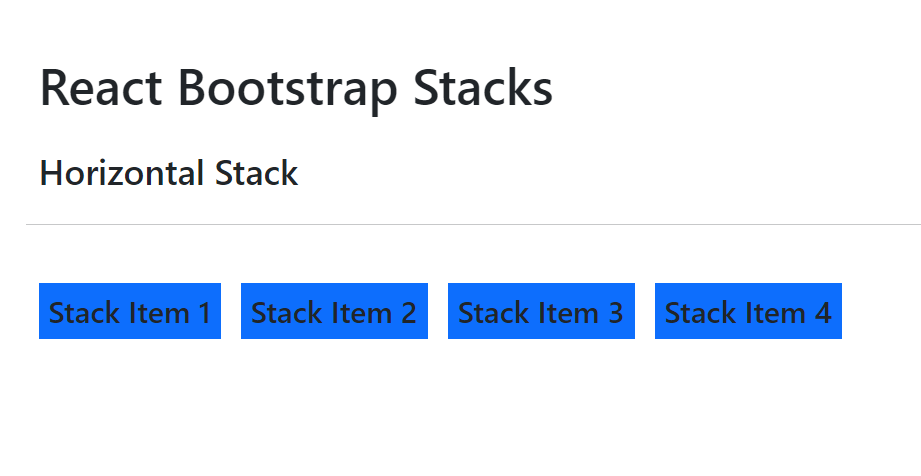
Example 2 Output
Share your thoughts in the comments
Please Login to comment...