React Bootstrap Form Range
Last Updated :
23 Jan, 2024
React Bootstrap Form Range is a react component from React Bootstrap library, it allows us to easily create a input range element inside react, which has a better default styling and looks the same across different browsers for consistency. The Input range element is highly used in forms in the front end to get the data from the user in the form of numeric value using a slider track and thumb.
Syntax:
import FormRange from "react-bootstrap/FormRange";
<FormRange ... />
FormRange API Properties
bsPrefix
|
string
|
{‘form-range’}
|
Changes the underlying component base class name, used as an escape hatch to heavily modify bootstrap css.
|
disabled
|
bool
|
|
Disables the given FormRange
|
value
|
string | number | arrayOf
|
|
The value attribute of the underlying input tag, controlled by onChange to update the state in react.
|
id
|
string
|
|
Uses controlId of FormGroup if not specifically provided.
|
onChange
|
function
|
|
A callback function get triggered for any change in the value of FormRange element
|
Creating React Application
Step 1: Create a react application using the below command.
npx create-react-app project-name
Step 2: Enter into the project directory using the cd command and open the project in your code editor.
cd project-name
Step 3: Install react-bootstrap and bootstrap dependencies using the below npm install command.
npm install react-bootstrap bootstrap
Step 4: Import the bootstrap css in the index.js file to use the css in every react components.
import "bootstrap/dist/css/bootstrap.css";
Project Structure
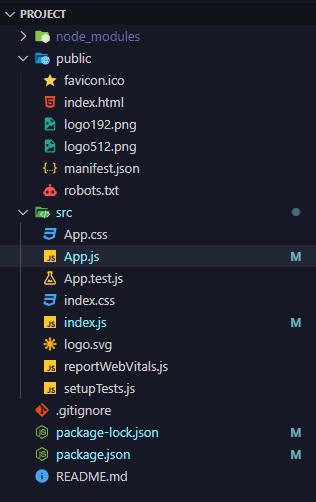
project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.2",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example 1:In this example, we will use the FormRange from React bootstrap to create a input range element, that looks good by default and also easy to implement in react. Open the App.js file in the project and add the below code.
Javascript
import { Container, Row } from "react-bootstrap" ;
import { useState } from "react" ;
import FormRange from "react-bootstrap/FormRange" ;
function App() {
const [value, setValue] = useState(50);
return (
<Container
bsPrefix= "container d-flex flex-column
justify-content-center align-items-center"
style={{ minHeight: 100 + "vh" }}
>
<h1 className= "mb-5" style={{ color: "#106C50" }}>
GeeksforGeeks
</h1>
<h3 className= "mb-5" >React Bootstrap Form Range</h3>
<Row className= "w-50 mb-5" >
<FormRange
value={value}
onChange={(event) => {
setValue(event.target.value);
}}
/>
</Row>
<Row>
<h3>Range Value: {value}</h3>
</Row>
</Container>
);
}
export default App;
|
Output
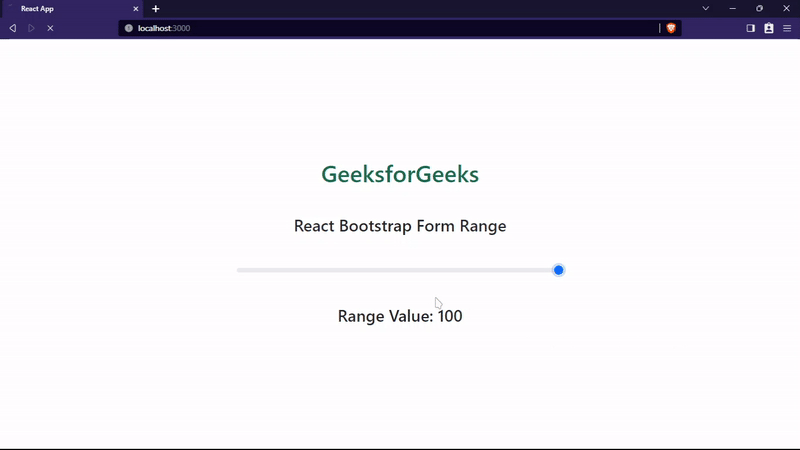
example output 1
Example 2:In the below example, disabled property is used to disable the FormRange of React Bootstrap.
Javascript
import { Container, Row } from "react-bootstrap" ;
import { useState } from "react" ;
import FormRange from "react-bootstrap/FormRange" ;
function App() {
const [value, setValue] = useState(50);
return (
<Container
bsPrefix= "container d-flex flex-column
justify-content-center align-items-center"
style={{ minHeight: 100 + "vh" }}
>
<h1 className= "mb-5" style={{ color: "#106C50" }}>
GeeksforGeeks
</h1>
<h3 className= "mb-5" >React Bootstrap Form Range</h3>
<Row className= "w-50 mb-5" >
<FormRange
value={value}
onChange={(event) => {
setValue(event.target.value);
}}
/>
</Row>
<Row>
<h3>Range Value: {value}</h3>
</Row>
</Container>
);
}
export default App;
|
Output
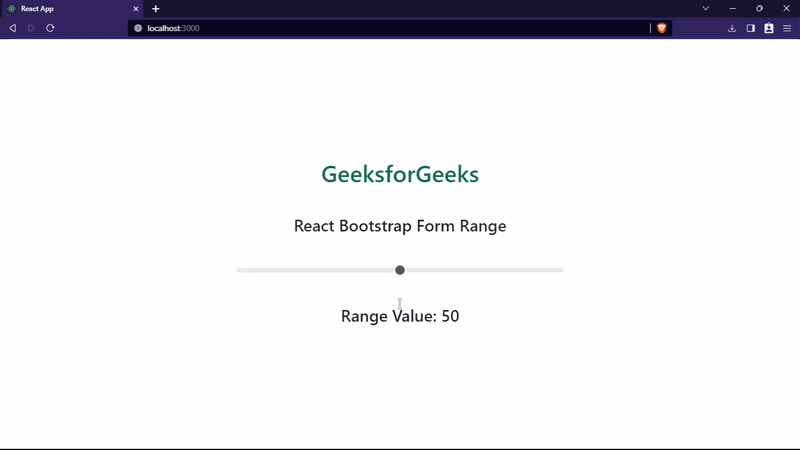
example output 2
Share your thoughts in the comments
Please Login to comment...