How to Add Icon in NavBar using React-Bootstrap ?
Last Updated :
17 Oct, 2023
This article will show you how to add an icon in the navigation bar using React-Bootstrap. We will use React-Bootstrap because it makes it easy to use reusable components without implementing them.
Prerequisites
Creating React App and Installing Module
Step 1: Create a React application using the following command
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command.
cd foldername
Step 3: Now install React-bootstrap and bootstrap
npm install react-bootstrap bootstrap
Step 4: Add Bootstrap CSS to index.js
import 'bootstrap/dist/css/bootstrap.min.css';
Project Structure
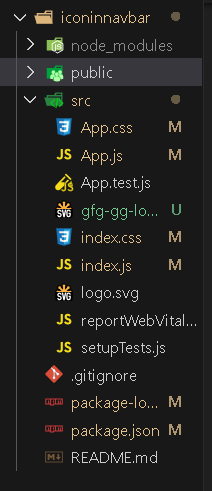
Project Structure
The updated dependencies in package.json will look like this:
{
"name": "iconinnavbar",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
}
Example: Now write down the following code in App.js file. Here App is our default component where we have written our code.
Javascript
import Container from "react-bootstrap/Container" ;
import Nav from "react-bootstrap/Nav" ;
import Navbar from "react-bootstrap/Navbar" ;
import gfglogo from "./GeeksforGeeks.svg" ;
import "bootstrap/dist/css/bootstrap.min.css" ;
function App() {
return (
<div>
<Navbar collapseOnSelect expand= "lg"
className= "bg-info" >
<Container>
<Navbar.Brand href= "#home" >
<img
src={gfglogo}
width= "50"
height= "50"
className= "d-inline-block align-top"
alt= "React Bootstrap logo"
/>
</Navbar.Brand>
<Navbar.Toggle
aria-controls= "responsive-navbar-nav" />
<Navbar.Collapse id= "responsive-navbar-nav" >
<Nav className= "me-auto" >
<Nav.Link href= "#DataStructures" >
Data Structures
</Nav.Link>
<Nav.Link
href= "#CompetitiveProgramming" >
Competitive Programming
</Nav.Link>
</Nav>
<Nav>
<Nav.Link href= "#contactus" >
Contact Us
</Nav.Link>
<Nav.Link eventKey={2}
href= "#community" >
Community
</Nav.Link>
</Nav>
</Navbar.Collapse>
</Container>
</Navbar>
</div>
);
}
export default App;
|
Steps To Run Application
Step: Run the application using following command from root directory of Application
npm start
Output: Now open the browser and type the ‘http://localhost:3000/’
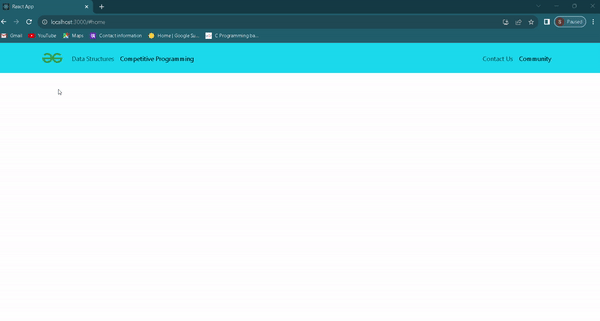
Navbar with Icon
Share your thoughts in the comments
Please Login to comment...