How to disable auto height for sliders by using React Bootstrap carousel?
Last Updated :
06 Nov, 2023
In ReactJS applications, we use Carousel to represent the images in a loop and in an attractive way. In many cases, the images that are added are not of the same height, so the height is been adjusted automatically. As there is no inbuilt functionality to disable the auto height for sliders in React Bootstrap Carousel, we can use different approaches like by using the utility class in Bootstrap and by using the styled components. This will allow us to set the fixed or maximum height of the image to render it properly on the Carousel Slider. So in this article, we will see both these approaches along with examples.
Prerequisite:
Steps to create React Application and Installing Bootstrap:
Step 1: Create a React application using the following command:
npx create-react-app carousel-height
Step 2: After creating your project folder(i.e. carousel-height), move to it by using the following command:
cd props-component
Step 3: Now install react-bootstrap and styled-components in your working directory i.e. carousel-height by executing the below command in the VScode terminal:
npm install react-bootstrap bootstrap styled-components
Step 4: Now we need to Add Bootstrap CSS to the index.js file:
import 'bootstrap/dist/css/bootstrap.min.css';
Project Structure:
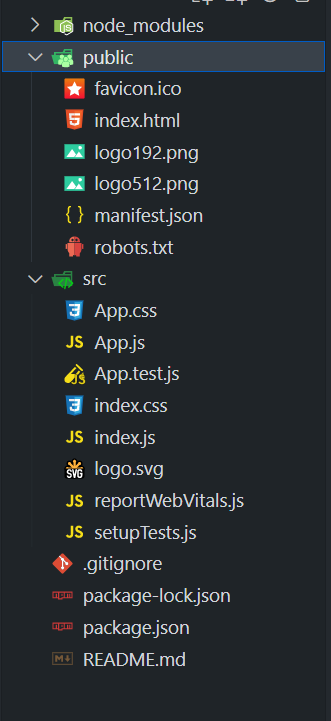
Example 1: In this example, we have sets a fixed height of 900px for all carousel items, which means each slide will have the same height regardless of the content inside. This stops the carousel from adjusting its height automatically based on the content’s size.
Javascript
import React from 'react' ;
import { Carousel, Container } from 'react-bootstrap' ;
function App() {
const carouselItemStyle = {
height: '900px' ,
};
return (
<Container className= "text-center mt-5" >
<h1 style={{ color: 'green' }}>GeeksforGeeks</h1>
<h3>Using Fixed Height Value</h3>
<Carousel>
<Carousel.Item style={carouselItemStyle}>
<img
src=
className= "d-block w-100"
alt= "Slide 1"
/>
</Carousel.Item>
<Carousel.Item style={carouselItemStyle}>
<img
src=
className= "d-block w-100"
alt= "Slide 2"
/>
</Carousel.Item>
<Carousel.Item style={carouselItemStyle}>
<img
src=
className= "d-block w-100"
alt= "Slide 3"
/>
</Carousel.Item>
</Carousel>
</Container>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
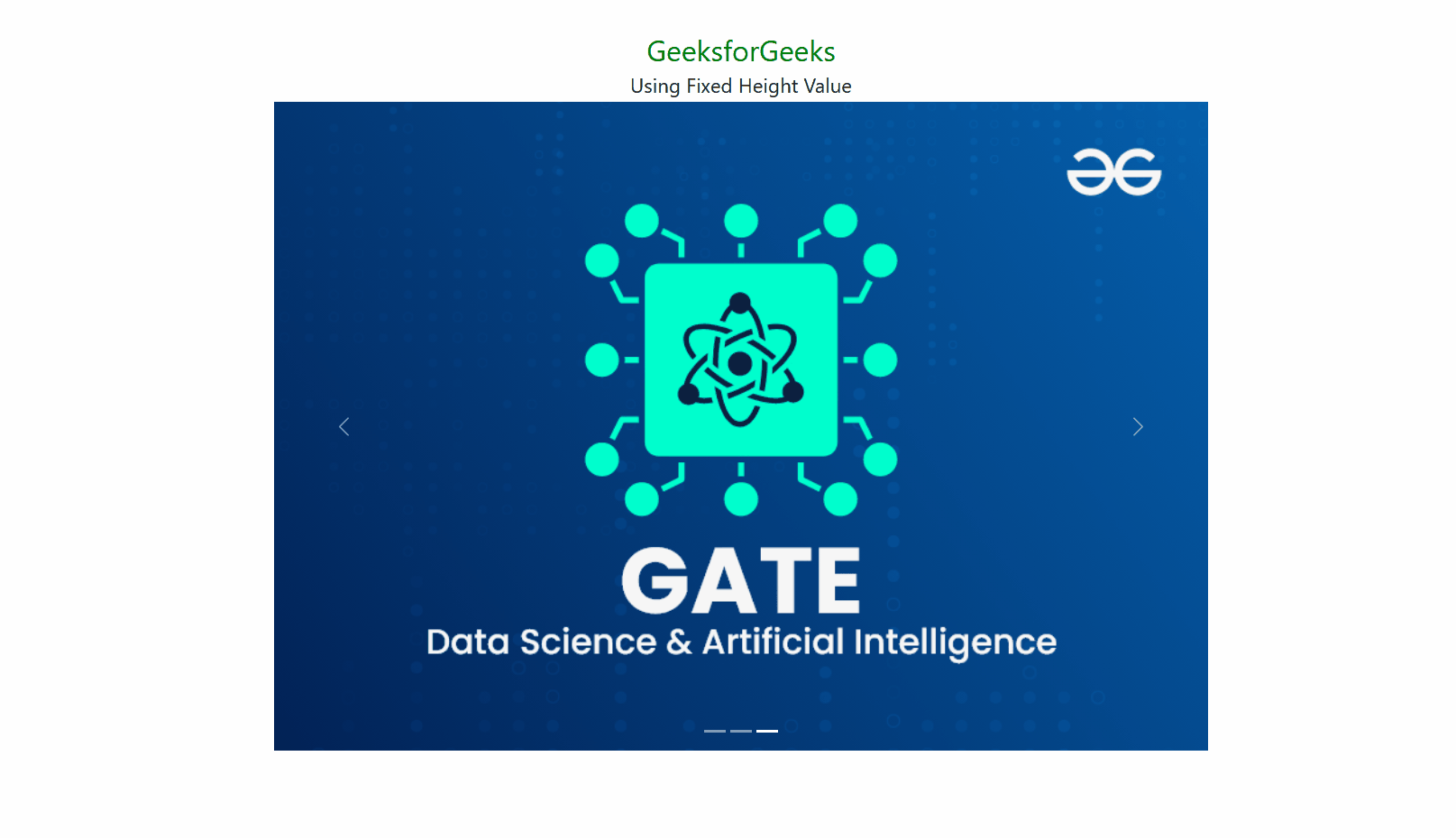
Example 2: In this example, we have used the “styled-components
” library to disable the autoheight for the sliders. Here, we creating a custom CustomCarousel
component, the maxHeight
state is set dynamically using JavaScript to determine the maximum height among the carousel items. Styled components are then used to set a fixed height for the carousel (CustomCarousel
) based on the calculated maxHeight
, effectively disabling the auto height adjustment.
Javascript
import React, { useEffect, useState } from 'react' ;
import { Carousel } from 'react-bootstrap' ;
import styled from 'styled-components' ;
const CustomCarousel = styled(Carousel)`
height: ${({ maxHeight }) => `${maxHeight}px`};
.carousel-inner {
display: block;
}
`;
const Container = styled.div`
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #f0f0f0;
`;
const TextContainer = styled.div`
text-align: center;
margin-bottom: 20px;
`;
const GreenH1 = styled.h1`
color: green;
`;
const GreenH3 = styled.h3`
color: black;
`;
const Image = styled.img`
max-width: 100%;
height: 300px;
`;
function App() {
const [maxHeight, setMaxHeight] = useState(0);
const [index, setIndex] = useState(0);
useEffect(() => {
const items = Array.from(document.querySelectorAll( '.carousel-item' ));
let max = 0;
items.forEach(item => {
const slideHeight = item.clientHeight;
max = Math.max(max, slideHeight);
});
setMaxHeight(max);
}, []);
const handleSelect = (selectedIndex) => {
setIndex(selectedIndex);
};
return (
<Container>
<TextContainer>
<GreenH1>GeeksforGeeks</GreenH1>
<GreenH3>Using Styled Components</GreenH3>
</TextContainer>
<CustomCarousel
maxHeight={maxHeight}
interval={ null }
activeIndex={index}
onSelect={handleSelect}
controls={ true }
wrap={ false }
>
<Carousel.Item className= "crossfade" >
<div>
<Image
src=
alt= "Slide 1"
/>
</div>
</Carousel.Item>
<Carousel.Item className= "crossfade" >
<div>
<Image
src=
alt= "Slide 2"
/>
</div>
</Carousel.Item>
<Carousel.Item className= "crossfade" >
<div>
<Image
src=
alt= "Slide 3"
/>
</div>
</Carousel.Item>
</CustomCarousel>
</Container>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
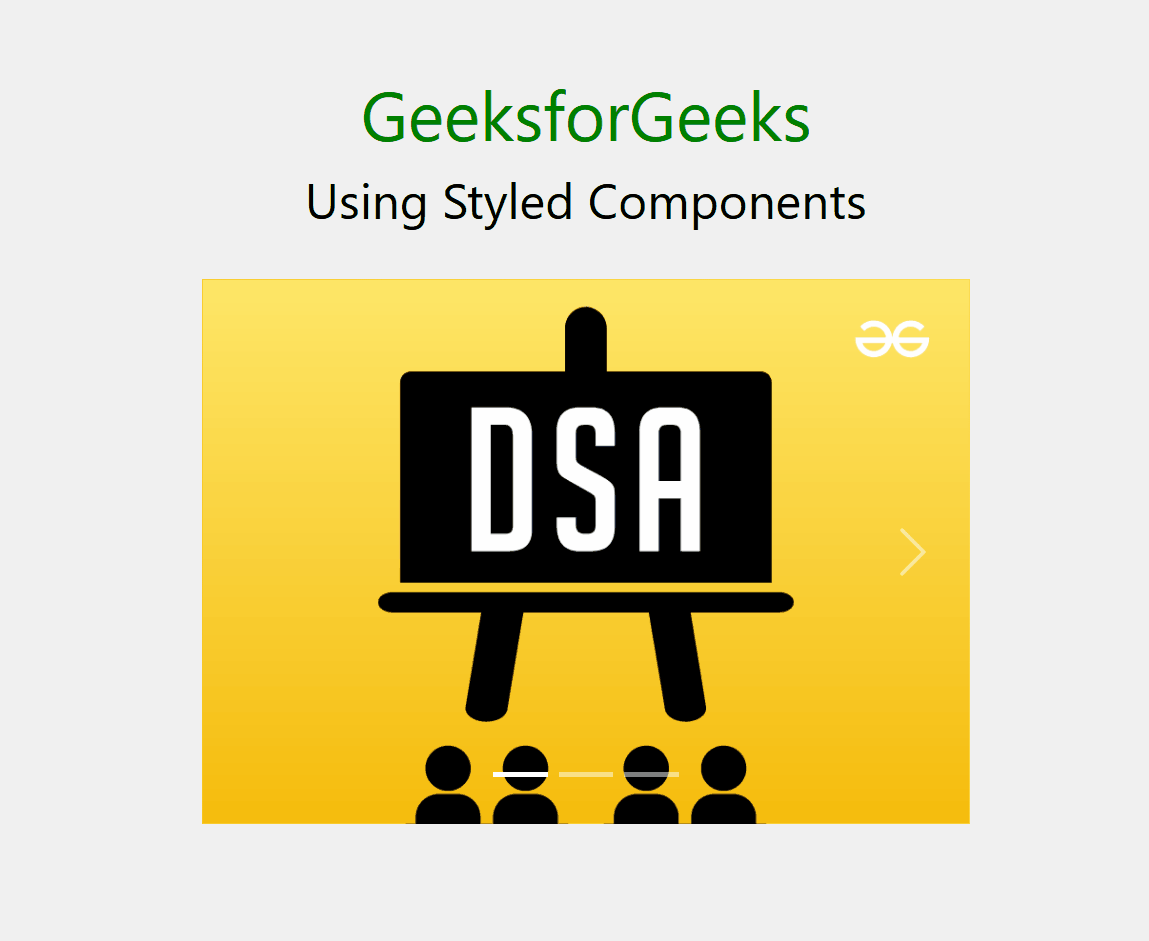
Share your thoughts in the comments
Please Login to comment...