How to Add a Logo to the React Bootstrap Navbar ?
Last Updated :
31 Oct, 2023
In this article, we will see how to add the Logo to the Navbar using React Bootstrap. The Navbar or Navigation bar is one of the most important UI components for dynamic and single-page applications. The navbar provides a better user experience and navigation over different components. In this navigation bar, we can perform different customizations like changing its color, layout, styling, etc.
Prerequisites:
Steps to Create React Application and Installing Bootstrap:
- Create a React application using the following command:
npx create-react-app logo-nav
- After creating your project folder(i.e. logo-nav), move to it by using the following command:
cd logo-nav
- Now install react-bootstrap in your working directory i.e. logo-nav by executing the below command in the VScode terminal:
npm install react-bootstrap bootstrap
- Now we need to Add Bootstrap CSS to the index.js file:
import 'bootstrap/dist/css/bootstrap.min.css';
Project Structure:
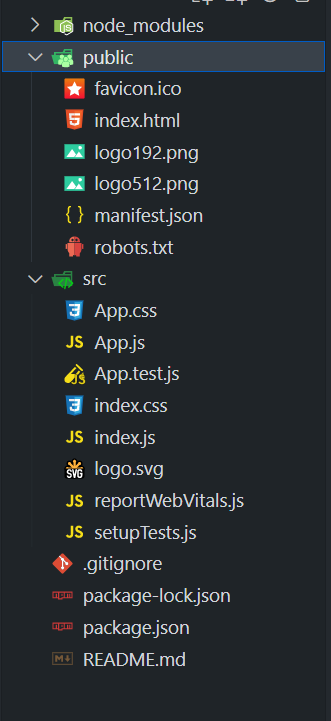
Example 1: In this example, we are displaying the logo on the left side of the NavBar, we are adding the GeeksforGeeks logo in the React Bootstrap Nabbar. The App component mainly creates the responsive React NavBar which has the dark theme.
Javascript
import React from 'react' ;
import { Navbar, Nav } from 'react-bootstrap' ;
function App() {
const logoUrl =
return (
<Navbar bg= "dark" expand= "lg"
variant= "dark"
className= "container-fluid" >
<Navbar.Brand href= "#home" >
<img src={logoUrl}
width= "50" height= "50"
alt= "Logo" />
<span className= "brand-text" >
GeeksforGeeks
</span>
</Navbar.Brand>
<Navbar.Toggle
aria-controls= "basic-navbar-nav" />
<Navbar.Collapse
id= "basic-navbar-nav" >
<Nav className= "ml-auto" >
<Nav.Link href= "#pick" >
Pick Article
</Nav.Link>
<Nav.Link href= "#suggest" >
Suggest Article
</Nav.Link>
<Nav.Link href= "#write" >
Write an Article
</Nav.Link>
</Nav>
</Navbar.Collapse>
</Navbar>
);
}
export default App;
|
Steps to run: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
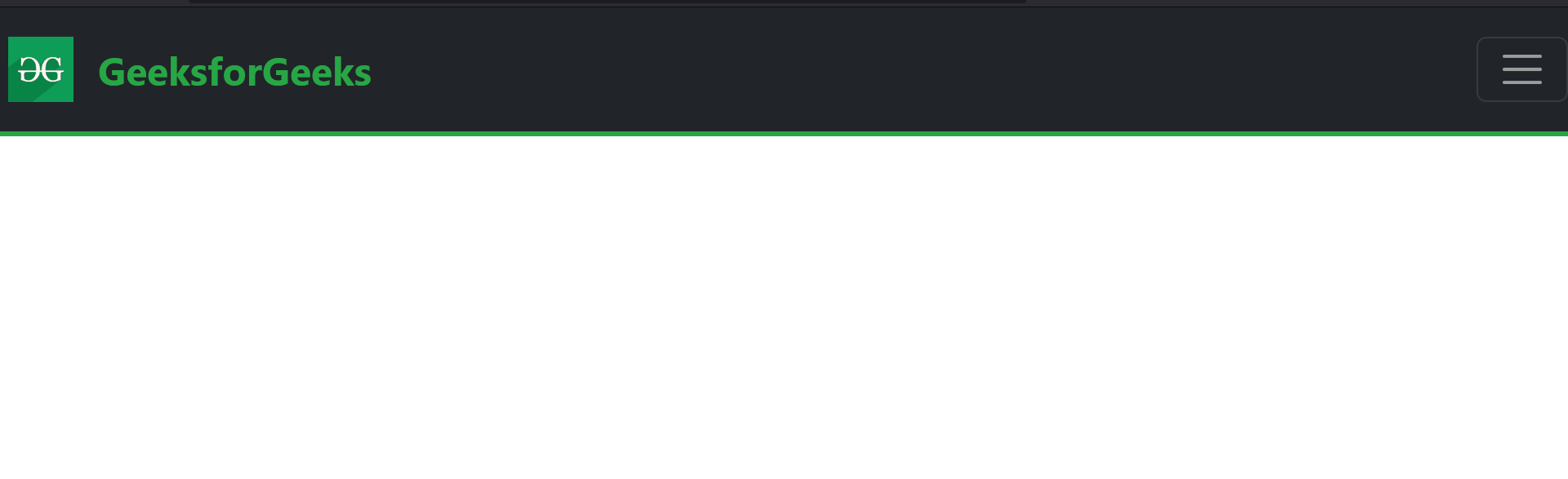
Example 2: In this example, we are showing the GeeksforGeeks icon on the right side of the navigation bar.
Javascript
import React from 'react' ;
import { Navbar, Nav } from 'react-bootstrap' ;
function App() {
const logoUrl =
return (
<Navbar bg= "dark" expand= "lg"
variant= "dark"
className= "container-fluid" >
<Navbar.Toggle aria-controls= "basic-navbar-nav" />
<Navbar.Collapse id= "basic-navbar-nav" >
<Nav className= "mr-auto" >
<Nav.Link href= "#pick" >
Pick Article
</Nav.Link>
<Nav.Link href= "#suggest" >
Suggest Article
</Nav.Link>
<Nav.Link href= "#write" >
Write an Article
</Nav.Link>
</Nav>
</Navbar.Collapse>
<div className= "logo-container" >
<img
src={logoUrl}
className= "logo img-fluid"
alt= "Logo"
width= "50" height= "50"
/>
</div>
</Navbar>
);
}
export default App;
|
Steps to run: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
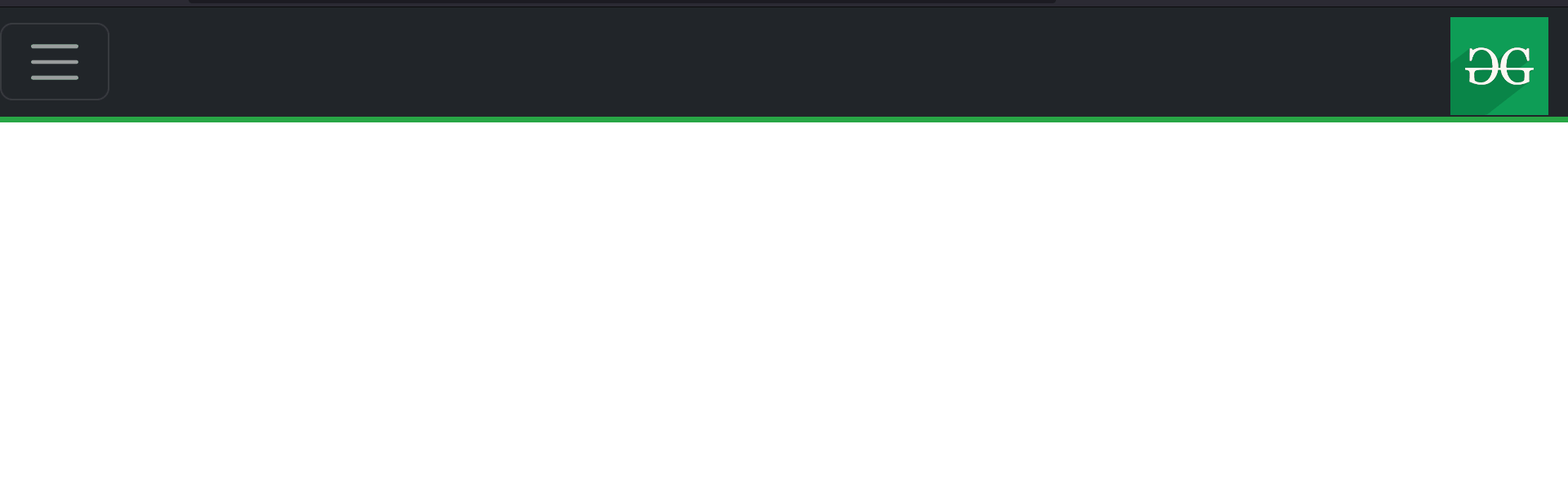
Share your thoughts in the comments
Please Login to comment...