How to get a react bootstrap card to center vertically?
Last Updated :
03 Nov, 2023
In this article, we will see how to center a React Bootstrap card vertically, you can use various approaches, including CSS flexbox, CSS grid, and CSS positioning.
Steps to create React App And Install Required Module:
Step 1: Create a React application using the following command
npx create-react-app foldername
Step 2: After creating your project folder i.e. folder name, move to it using the following command.
cd foldername
Step 3: After creating the ReactJS application, Install React Bootstrap (if not already installed):
npm install react-bootstrap bootstrap
Step 4: Import Bootstrap CSS in Index.js file.
import 'bootstrap/dist/css/bootstrap.min.css';
The updated dependencies in package.json file will look like:
"dependencies": {
"bootstrap": "5.3.2",
"react": "16.12.0",
"react-bootstrap": "1.0.0-beta.16",
"react-dom": "16.12.0",
"react-scripts": "3.0.1"
},
Approach 1: Using Flexbox
Flexbox is a powerful CSS layout model that makes it easy to vertically center elements. You can use the d-flex and align-items-center classes provided by React Bootstrap to center your card vertically.
Example: Below is the implementation of the above approach.
Javascript
import React from 'react' ;
import Card from 'react-bootstrap/Card' ;
function CenteredCard() {
const redBoxStyle = {
backgroundColor: 'red' ,
padding: '20px' ,
color: 'white' ,
};
return (
<div className= "d-flex align-items-center
justify-content-center vh-100" >
<Card>
<Card.Body>
<div style={redBoxStyle}>
<h5 className= "card-title" >
Red Box with Text
</h5>
<p className= "card-text" >
This is a red box with some text
</p>
</div>
</Card.Body>
</Card>
</div>
);
}
export default CenteredCard;
|
Step to Run Application:
Step 1: Run the application using the following command from the root directory of the project:
npm start
Step 2: Open Browser and search the given URL:
http://localhost:3000/
Output:
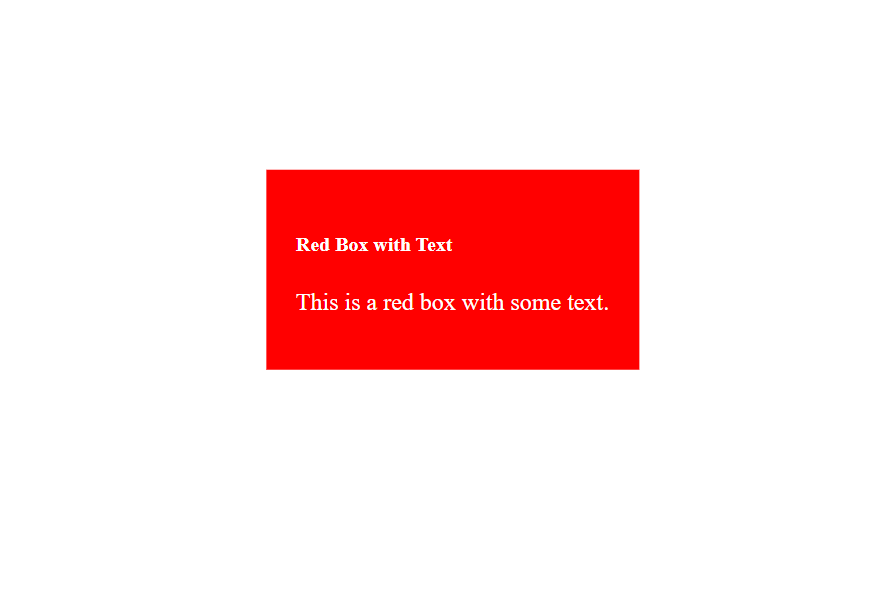
Output
Approach 2: Using CSS Grid
You can use CSS grid to create a grid layout that centers the card both horizontally and vertically.CSS Grid, also known as Grid Layout, is a powerful layout system in CSS for creating two-dimensional grid-based layouts in web design. It allows you to divide a webpage or container into rows and columns, making it easier to position and align elements on the page.
- display: grid; sets the display property of the container to grid, enabling grid layout.
- place-items: center; is a shorthand property that centers the grid items both horizontally and vertically.
- height: 100vh; ensures that the container takes up the full viewport height, which is useful for centering content on the entire screen.
Example: Below is the implementation of the above approach.
Javascript
import React from 'react' ;
import Card from 'react-bootstrap/Card' ;
function CenteredCard() {
const redBoxStyle = {
backgroundColor: 'red' ,
padding: '20px' ,
color: 'white' ,
};
return (
<div style={{
display: 'grid' ,
placeItems: 'center' ,
height: '100vh' ,
}}>
<Card>
<Card.Body style={redBoxStyle}>
<h5 className= "card-title" >
Red Box with Text
</h5>
<p className= "card-text" >
This is a red box with some text.
</p>
</Card.Body>
</Card>
</div>
);
}
export default CenteredCard;
|
Step to Run Application:
Step 1: Run the application using the following command from the root directory of the project:
npm start
Step 2: Open Browser and search the given URL:
http://localhost:3000/
Output:
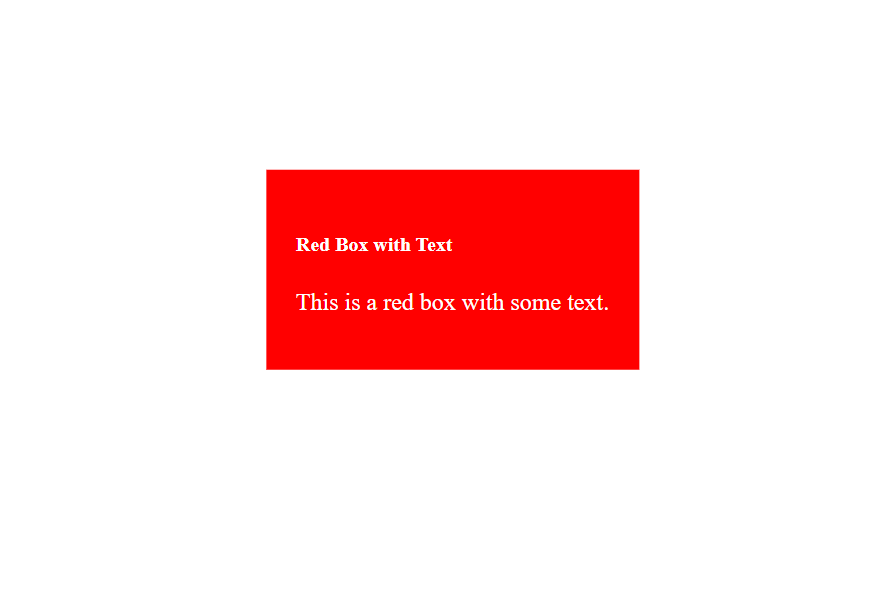
Output
Approach 3: Using Absolute Positioning
Absolute positioning in CSS is a technique used to precisely control the placement of an HTML element within its containing element, usually based on a specific location or coordinates on the webpage.
Example: Below is the implementation of the above approach.
Javascript
import React from 'react' ;
import Card from 'react-bootstrap/Card' ;
function CenteredCard() {
const redBoxStyle = {
backgroundColor: 'red' ,
padding: '20px' ,
color: 'white' ,
position: 'relative' ,
};
const centeredStyle = {
position: 'absolute' ,
top: '50%' ,
left: '50%' ,
transform: 'translate(-50%, -50%)' ,
};
return (
<div style={{
position: 'relative' ,
height: '100vh' ,
}}>
<div style={centeredStyle}>
<Card style={redBoxStyle}>
<Card.Body>
<h5 className= "card-title" >
Red Box with Text
</h5>
<p className= "card-text" >
This is a red box with some text.
</p>
</Card.Body>
</Card>
</div>
</div>
);
}
export default CenteredCard;
|
Step to Run Application:
Step 1: Run the application using the following command from the root directory of the project:
npm start
Step 2: Open Browser and search the given URL:
http://localhost:3000/
Output:

Output
Share your thoughts in the comments
Please Login to comment...