Python | Popup widget in Kivy
Last Updated :
06 Feb, 2020
Kivy is a platform independent GUI tool in Python. As it can be run on Android, IOS, linux and Windows etc. It is basically used to develop the Android application, but it does not mean that it can not be used on Desktops applications.
👉🏽 Kivy Tutorial – Learn Kivy with Examples.
Popup widget :
- The Popup widget is used to create popups. By default, the popup will cover the whole “parent” window. When you are creating a popup, you must at least set a Popup.title and Popup.content.
- Popup dialogs are used ]when we have to convey certain obvious messages to the user. Messages to the user through status bars as well for specific messages which need to be told with emphasis can still be done through popup dialogs.
- Keep one thing in mind that the default size of a widget is size_hint=(1, 1).
- If you don’t want your popup to be on the full screen you must gave either size hints with values less than 1 (for instance size_hint=(.8, .8)) or deactivate the size_hint and use fixed size attributes.
To use popup you must have to import :
from kivy.uix.popup import Popup
Note: Popup is a special widget. Don’t try to add it as a child to any other widget. If you do, Popup will be handled like an ordinary widget and won’t be created hidden in the background.
Basic Approach :
1) import kivy
2) import kivyApp
3) import Label
4) import button
5) import Gridlayout
6) import popup
7) Set minimum version(optional)
8) create App class
9) return Layout/widget/Class(according to requirement)
10) In the App class create the popup
11) Run an instance of the class
Code #1: In the first code the popup will cover the whole “parent” window.
import kivy
from kivy.app import App
kivy.require( '1.9.0' )
from kivy.uix.button import Button
from kivy.uix.gridlayout import GridLayout
from kivy.uix.popup import Popup
from kivy.uix.label import Label
from kivy.config import Config
Config. set ( 'graphics' , 'resizable' , True )
class PopupExample(App):
def build( self ):
self .layout = GridLayout(cols = 1 , padding = 10 )
self .button = Button(text = "Click for pop-up" )
self .layout.add_widget( self .button)
self .button.bind(on_press = self .onButtonPress)
return self .layout
def onButtonPress( self , button):
layout = GridLayout(cols = 1 , padding = 10 )
popupLabel = Label(text = "Click for pop-up" )
closeButton = Button(text = "Close the pop-up" )
layout.add_widget(popupLabel)
layout.add_widget(closeButton)
popup = Popup(title = 'Demo Popup' ,
content = layout)
popup. open ()
closeButton.bind(on_press = popup.dismiss)
if __name__ = = '__main__' :
PopupExample().run()
|
Output:
When click on screen popup will open like this:
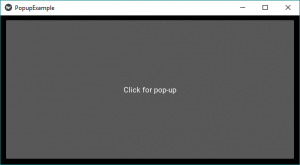
When click on Close the popup it will close.
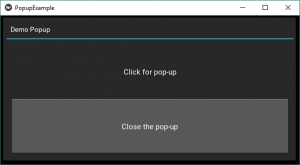
Code #2:
In the second code when we use the size_hint and the size we can give the size accordingly. In this just add something as in the below code in line number 75.
import kivy
from kivy.app import App
kivy.require( '1.9.0' )
from kivy.uix.button import Button
from kivy.uix.gridlayout import GridLayout
from kivy.uix.popup import Popup
from kivy.uix.label import Label
from kivy.config import Config
Config. set ( 'graphics' , 'resizable' , True )
class PopupExample(App):
def build( self ):
self .layout = GridLayout(cols = 1 , padding = 10 )
self .button = Button(text = "Click for pop-up" )
self .layout.add_widget( self .button)
self .button.bind(on_press = self .onButtonPress)
return self .layout
def onButtonPress( self , button):
layout = GridLayout(cols = 1 , padding = 10 )
popupLabel = Label(text = "Click for pop-up" )
closeButton = Button(text = "Close the pop-up" )
layout.add_widget(popupLabel)
layout.add_widget(closeButton)
popup = Popup(title = 'Demo Popup' ,
content = layout,
size_hint = ( None , None ), size = ( 200 , 200 ))
popup. open ()
closeButton.bind(on_press = popup.dismiss)
if __name__ = = '__main__' :
PopupExample().run()
|
Output:
Popup size will be smaller than the window size.
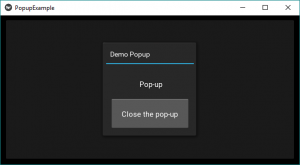
Reference : https://kivy.org/doc/stable/api-kivy.uix.popup.html
Share your thoughts in the comments
Please Login to comment...