Python | BoxLayout widget in Kivy
Last Updated :
19 Oct, 2021
Kivy is a platform independent GUI tool in Python. As it can be run on Android, IOS, Linux and Windows, etc. It is basically used to develop the Android application, but it does not mean that it can not be used on Desktops applications.
???????? Kivy Tutorial – Learn Kivy with Examples.
Now in this article, we will learn about the use of BoxLayout widget in kivy and how to add some features like color, size etc to it.
BoxLayout:
BoxLayout arranges widgets in either in a vertical fashion that is one on top of another or in a horizontal fashion that is one after another. When you will not provide any size-hint then the child widgets divides the size of its parent widget equally or accordingly.
Basic Approach to follow while creating button :
1) import kivy
2) import kivyApp
3) import BoxLayout
4) set minimum version(optional)
5) Add widgets
6) Extend the class
7) Return layout
8) Run an instance of the class
Below is the code to use BoxLayout:
Python3
import kivy
kivy.require( "1.9.1" )
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
class BoxLayoutApp(App):
def build( self ):
superBox = BoxLayout(orientation = 'vertical' )
HB = BoxLayout(orientation = 'horizontal' )
btn1 = Button(text = "One" )
btn2 = Button(text = "Two" )
HB.add_widget(btn1)
HB.add_widget(btn2)
VB = BoxLayout(orientation = 'vertical' )
btn3 = Button(text = "Three" )
btn4 = Button(text = "Four" )
VB.add_widget(btn3)
VB.add_widget(btn4)
superBox.add_widget(HB)
superBox.add_widget(VB)
return superBox
root = BoxLayoutApp()
root.run()
|
Output:
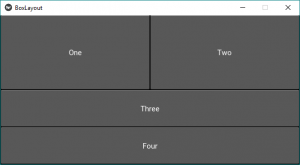
Some modification like add colour, fix size in Box layout widget:
Python3
import kivy
import random
kivy.require( "1.9.1" )
from kivy.app import App
from kivy.uix.button import Button
from kivy.uix.boxlayout import BoxLayout
red = [ 1 , 0 , 0 , 1 ]
green = [ 0 , 1 , 0 , 1 ]
blue = [ 0 , 0 , 1 , 1 ]
purple = [ 1 , 0 , 1 , 1 ]
class BoxLayoutApp(App):
def build( self ):
superBox = BoxLayout(orientation = 'vertical' )
HB = BoxLayout(orientation = 'horizontal' )
colors = [red, green, blue, purple]
btn1 = Button(text = "One" ,
background_color = random.choice(colors),
font_size = 32 ,
size_hint = ( 0.7 , 1 ))
btn2 = Button(text = "Two" ,
background_color = random.choice(colors),
font_size = 32 ,
size_hint = ( 0.7 , 1 ))
HB.add_widget(btn1)
HB.add_widget(btn2)
VB = BoxLayout(orientation = 'vertical' )
btn3 = Button(text = "Three" ,
background_color = random.choice(colors),
font_size = 32 ,
size_hint = ( 1 , 10 ))
btn4 = Button(text = "Four" ,
background_color = random.choice(colors),
font_size = 32 ,
size_hint = ( 1 , 15 ))
VB.add_widget(btn3)
VB.add_widget(btn4)
superBox.add_widget(HB)
superBox.add_widget(VB)
return superBox
root = BoxLayoutApp()
root.run()
|
Output:
Picture1:
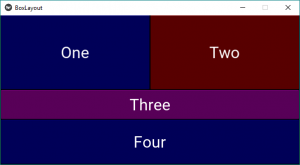
Picture2:
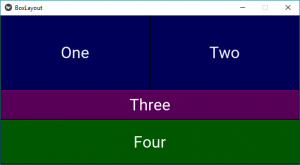
Note: Every time you run the code it provides you the different color outputs as in the pictures.
Share your thoughts in the comments
Please Login to comment...