Python | Checkbox widget in Kivy
Last Updated :
19 Oct, 2021
Kivy is a platform independent GUI tool in Python. Kivy applications can be run on Android, IOS, linux, and Windows, etc. It is basically used to develop the Android application, but it does not mean that it can not be used on Desktop applications.
Kivy Tutorial – Learn Kivy with Examples.
Checkbox widget –
CheckBox is a specific two-state button that can be either checked or unchecked. Checkboxes have an accompanying label that describes the purpose of the checkbox. Checkboxes can be grouped together to form radio buttons. Checkboxes are used to convey whether a setting is to be applied or not.
To work with the Checkbox, you first have to import Checkbox from the module which consists all features, functions of the slider i.e.
from kivy.uix.checkbox import CheckBox
Basic Approach to follow while creating Slider :
1) import kivy
2) import kivy App
3) import gridlayout
4) import Label
5) import Checkbox
6) import Widget
7) set minimum version(optional)
8) Extend the class
9) Add widget in the class
10) Create the App class
11) run the instance of the class
Now the program of How to create Checkbox in Kivy:
Python3
import kivy
from kivy.app import App
from kivy.uix.widget import Widget
from kivy.uix.label import Label
from kivy.uix.checkbox import CheckBox
from kivy.uix.gridlayout import GridLayout
class check_box(GridLayout):
def __init__( self , * * kwargs):
super (check_box, self ).__init__( * * kwargs)
self .cols = 2
self .add_widget(Label(text = 'Male' ))
self .active = CheckBox(active = True )
self .add_widget( self .active)
self .add_widget(Label(text = 'Female' ))
self .active = CheckBox(active = True )
self .add_widget( self .active)
self .add_widget(Label(text = 'Other' ))
self .active = CheckBox(active = True )
self .add_widget( self .active)
class CheckBoxApp(App):
def build( self ):
return check_box()
if __name__ = = '__main__' :
CheckBoxApp().run()
|
Output:
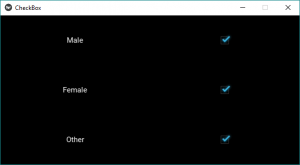
Now Question is that How can we bind or attach callback to Checkbox?
So the simple example is given which bind Checkbox with the click i.e when it clicked it print “Checkbox Checked” else it will print “Checkbox unchecked”.
Now program to arrange a callback to Checkbox i.e whether checkbox is checked or not.
Python3
import kivy
from kivy.app import App
from kivy.uix.widget import Widget
from kivy.uix.label import Label
from kivy.uix.checkbox import CheckBox
from kivy.uix.gridlayout import GridLayout
class check_box(GridLayout):
def __init__( self , * * kwargs):
super (check_box, self ).__init__( * * kwargs)
self .cols = 2
self .add_widget(Label(text = 'Male' ))
self .active = CheckBox(active = True )
self .add_widget( self .active)
self .lbl_active = Label(text = 'Checkbox is on' )
self .add_widget( self .lbl_active)
self .active.bind(active = self .on_checkbox_Active)
def on_checkbox_Active( self , checkboxInstance, isActive):
if isActive:
self .lbl_active.text = "Checkbox is ON"
print ( "Checkbox Checked" )
else :
self .lbl_active.text = "Checkbox is OFF"
print ( "Checkbox unchecked" )
class CheckBoxApp(App):
def build( self ):
return check_box()
if __name__ = = '__main__' :
CheckBoxApp().run()
|
Output:
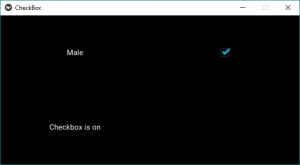
Video Output:
Reference: https://kivy.org/doc/stable/api-kivy.uix.checkbox.html.
Share your thoughts in the comments
Please Login to comment...