Python| AnchorLayout in Kivy
Last Updated :
06 Feb, 2020
Kivy is a platform independent GUI tool in Python. As it can be run on Android, IOS, linux and Windows etc. It is basically used to develop the Android application, but it does not mean that it can not be used on Desktops applications.
👉🏽 Kivy Tutorial – Learn Kivy with Examples.
AnchorLayout:
The AnchorLayout aligns its children to a border (top, bottom, left, right) or center. The class given below is used to implement the anchor layout.
kivy.uix.anchorlayout.AnchorLayout
AnchorLayout can be initialized with parameters:
anchor_x
Parameters can be passed: “left”, “right” and “center”.
anchor_y
Parameters can be passed:“top”, “bottom” and “center”.
to select the place where the widgets are placed in the parent container.
There are 9 different layout regions where the Anchorlayout
can be placed for effect:
Top-left, top-center, top-right, center-left, center-center, center-right, bottom-left, bottom-center and bottom-right.
Note: Remember adding multiple widgets to an anchor layout, only positions the widgets at the same location.
Basic Approach:
1) import kivy
2) import kivyApp
4) import Anchorlayout
5) Set minimum version(optional)
6) create App class
7) return Layout/widget/Class(according to requirement)
8) Run an instance of the class
Implementation of the Approach (with some Styling):
1) anchor_x=’right’, anchor_y=’bottom’:
from kivy.app import App
from kivy.uix.anchorlayout import AnchorLayout
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.button import Button
class AnchorLayoutApp(App):
def build( self ):
layout = AnchorLayout(
anchor_x = 'right' , anchor_y = 'bottom' )
btn = Button(text = 'Hello World' ,
size_hint = (. 3 , . 3 ),
background_color = ( 1.0 , 0.0 , 0.0 , 1.0 ))
layout.add_widget(btn)
return layout
root = AnchorLayoutApp()
root.run()
|
Output:
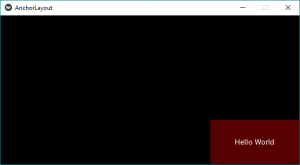
If you want to change the positions of the AnchorLayouts
then just replace the class code in the above code with below or you can change the anchor_x
and anchor_y with any of the parameters to make any 9 combinations as described above.
2) anchor_x=’right’, anchor_y=’top’:
class AnchorLayoutApp(App):
def build( self ):
layout = AnchorLayout(
anchor_x = 'right' , anchor_y = 'top' )
btn = Button(text = 'Hello World' ,
size_hint = (. 3 , . 3 ),
background_color = ( 1.0 , 0.0 , 1.0 , 1.0 ))
layout.add_widget(btn)
return layout
|
Output:
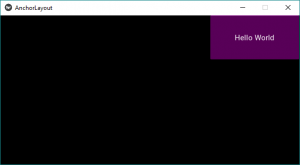
3) anchor_x=’center’, anchor_y=’top’:
Output:
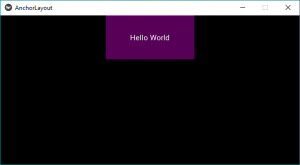
4) anchor_x=’left’, anchor_y=’top’:
Output:
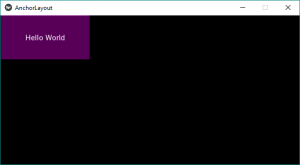
5) anchor_x=’left’, anchor_y=’bottom’:
Output:
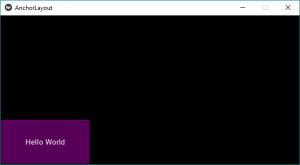
6) anchor_x=’left’, anchor_y=’center’:
Output:
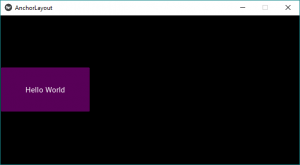
7) anchor_x=’center’, anchor_y=’center’:
Output:
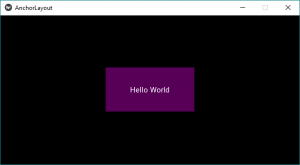
8) anchor_x=’center’, anchor_y=’bottom’:
Output:
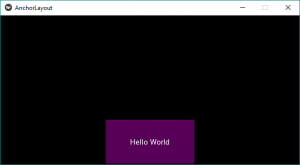
9) anchor_x=’right’, anchor_y=’center’:
Output:
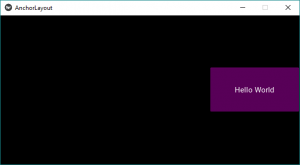
Share your thoughts in the comments
Please Login to comment...