How to pass data to a React Bootstrap modal?
Last Updated :
02 Nov, 2023
In React Bootstrap, we have the styling and interactive components of Modal. In simple terms, Modal is nothing but the popup box that is opened when some action has been performed. To make the modal customizable in terms of its behavior, we can pass the custom data to the React Bootstrap modal using state variables and also by using the props in ReactJS.
In this article, we will learn how we can pass the user-defined or provided data to the bootstrap modal.
Prerequisite
Steps to create React Application and installing required modules:
Step 1: Create a React application using the following command:
npx create-react-app modal-data
Step 2: After creating your project folder(i.e. modal-data), move to it by using the following command:
cd modal-data
Step 3: Now install react-bootstrap in your working directory i.e. modal-data by executing the below command in the VScode terminal:
npm install react-bootstrap bootstrap
Step 4: Now we need to Add Bootstrap CSS to the index.js file:
import 'bootstrap/dist/css/bootstrap.min.css';
Approach 1: Using State Variables
Import the Modal component from the React Bootstrap library. Here we have the App component, in which there is a state variable like showM and modalData to hold or store the data. Here, when the user enters the data in the text field and clicks on the button, the input value gets stored in the modalData, and the modal that is opened is displayed with this data. Here we are using the state variable to dynamically change and update the data, which is passed to the Modal component.
Example 1: This example implements the above-mentioned approach.
Javascript
import React, { useState } from "react" ;
import {
Button, Modal, Form,
Container, Row, Col,
} from "react-bootstrap" ;
function App() {
const [showM, set_Show_M] =
useState( false );
const [modalData, set_Modal_Data] =
useState( "" );
const [input, set_Input_Val] =
useState( "" );
const modalShow = () => {
set_Show_M( true );};
const closeModal = () => {
set_Show_M( false );};
const inputChange = (e) => {
set_Input_Val(e.target.value);};
const openModalHandle = () => {
set_Modal_Data(input);
modalShow();};
return (
<div className= "App text-center" >
<h1 className= "text-success mb-4" >
GeeksforGeeks
</h1>
<h3>
Passing Data Using State Variable
</h3>
<Container>
<Row className= "justify-content-center" >
<Col md={6}>
<Form>
<Form.Control
type= "text"
placeholder= "Enter Data"
value={
input}
onChange={
inputChange}
className= "mb-3" />
</Form>
<Button
onClick={
openModalHandle}
variant= "success" >
Open Modal
</Button>
</Col>
</Row>
</Container>
<Modal
show={showM}
onHide={closeModal}>
<Modal.Header
closeButton
className= "bg-primary text-white" >
<Modal.Title>
Data in Modal
</Modal.Title>
</Modal.Header>
<Modal.Body>
<h1 className= "text-primary" >
{modalData}
</h1>
</Modal.Body>
<Modal.Footer>
<Button
variant= "secondary"
onClick={
closeModal}
className= "text-danger" >
Close
</Button>
</Modal.Footer>
</Modal>
</div>
);
}
export default App;
|
Output:
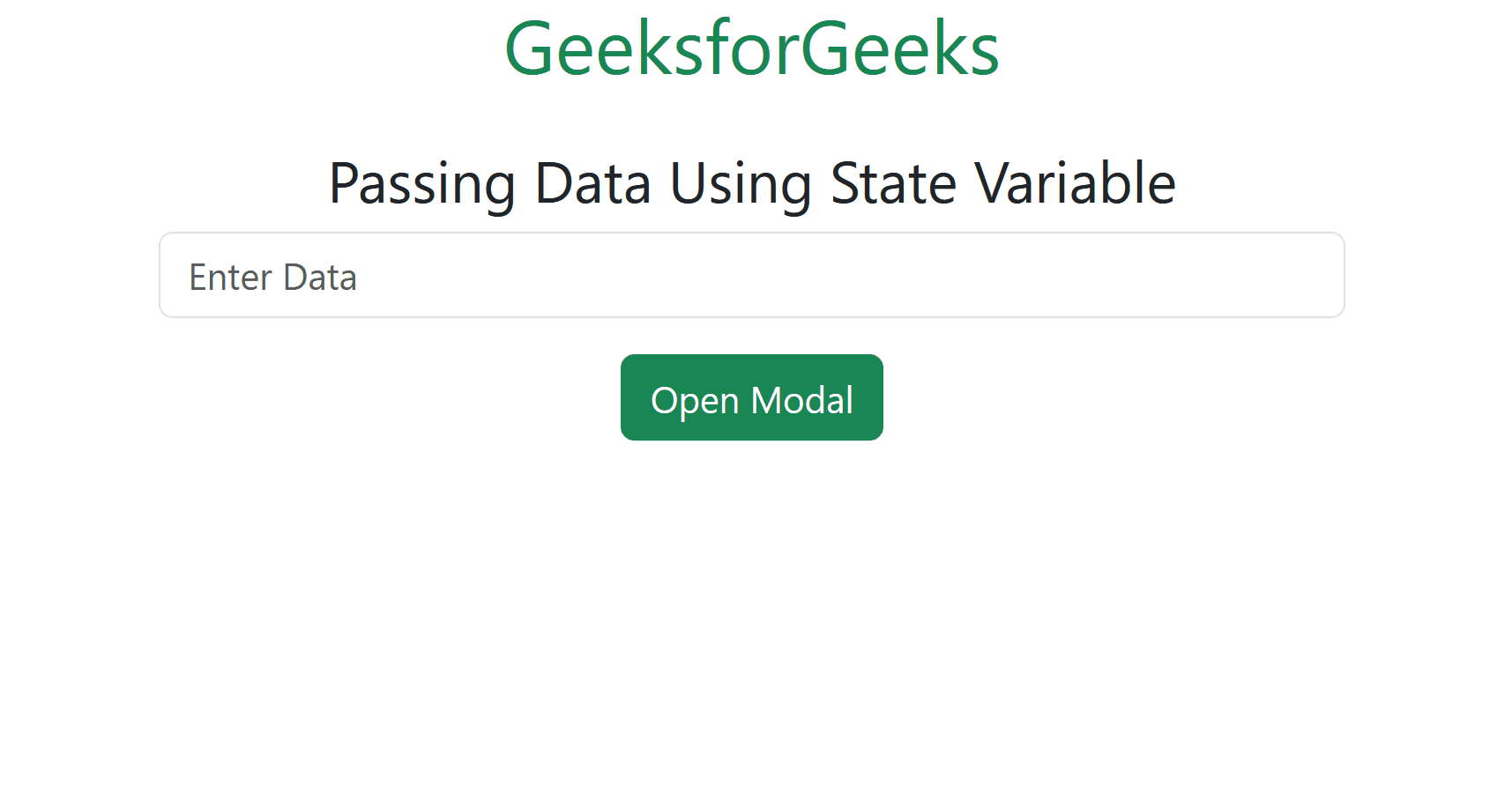
Approach 2: Using Props
Import the modal component and defined the two modal components. There is ModalDataFunction, which revives the props as showModal, handleClose, modalData, and modalTitle. When the user enters the data into the input fields, then the data that is entered is passed as the modalData props to the particulate modal component. This method is more efficient for passing the data and displaying it within the modular component.
Example: This example implements the above-mentioned approach.
Javascript
import React, { useState } from "react" ;
import {
Button, Modal, Form,
Container, Row, Col,
} from "react-bootstrap" ;
function ModalDataFunction({
showModal, handleClose,
modalData, modalTitle,
}) {
return (
<Modal
show={showModal}
onHide={handleClose}>
<Modal.Header
closeButton
className= "bg-success text-white" >
<Modal.Title>
{modalTitle}
</Modal.Title>
</Modal.Header>
<Modal.Body>
<h1 className= "text-success" >
{modalData}
</h1>
</Modal.Body>
<Modal.Footer>
<Button
variant= "secondary"
onClick={
handleClose}
className= "text-success" >
Close
</Button>
</Modal.Footer>
</Modal>
);
}
function App() {
const [m1Show, set_Show_M1] =
useState( false );
const [m2Show, set_Show_M2] =
useState( false );
const [m1Data, set_Modal_D1] =
useState( "" );
const [m2Data, set_Modal_D2] =
useState( "" );
const [input1, set_Input_Val1] =
useState( "" );
const [input2, set_Input_Val2] =
useState( "" );
const openM1 = () => {
set_Show_M1( true );};
const closeM1 = () => {
set_Show_M1( false );};
const openM2 = () => {
set_Show_M2( true );};
const closeM2 = () => {
set_Show_M2( false );};
const handleInputChange1 = (e) => {
set_Input_Val1(e.target.value);};
const input2Change = (e) => {
set_Input_Val2(e.target.value);};
const openM1Handle = () => {
set_Modal_D1(input1);
openM1();};
const openM2Handle = () => {
set_Modal_D2(input2);
openM2();};
return (
<Container className= "App text-center" >
<h1 className= "text-success mb-4" >
GeeksforGeeks
</h1>
<h3>
Passing Data Using Props
</h3>
<Row className= "justify-content-center mb-4" >
<Col md={6}>
<Form>
<Form.Control
type= "text"
placeholder= "Enter Data for Modal 1"
value={
input1}
onChange={
handleInputChange1}
className= "mb-3" />
</Form>
<Button
onClick={
openM1Handle}
variant= "success" >
Open Modal 1
</Button>
</Col>
</Row>
<Row className= "justify-content-center mb-4" >
<Col md={6}>
<Form>
<Form.Control
type= "text"
placeholder= "Enter Data for Modal 2"
value={
input2}
onChange={
input2Change}
className= "mb-3" />
</Form>
<Button
onClick={
openM2Handle}
variant= "primary" >
Open Modal 2
</Button>
</Col>
</Row>
<ModalDataFunction
showModal={m1Show}
handleClose={closeM1}
modalData={m1Data}
modalTitle= "Modal 1" />
<ModalDataFunction
showModal={m2Show}
handleClose={closeM2}
modalData={m2Data}
modalTitle= "Modal 2" />
</Container>
);
}
export default App;
|
Output:
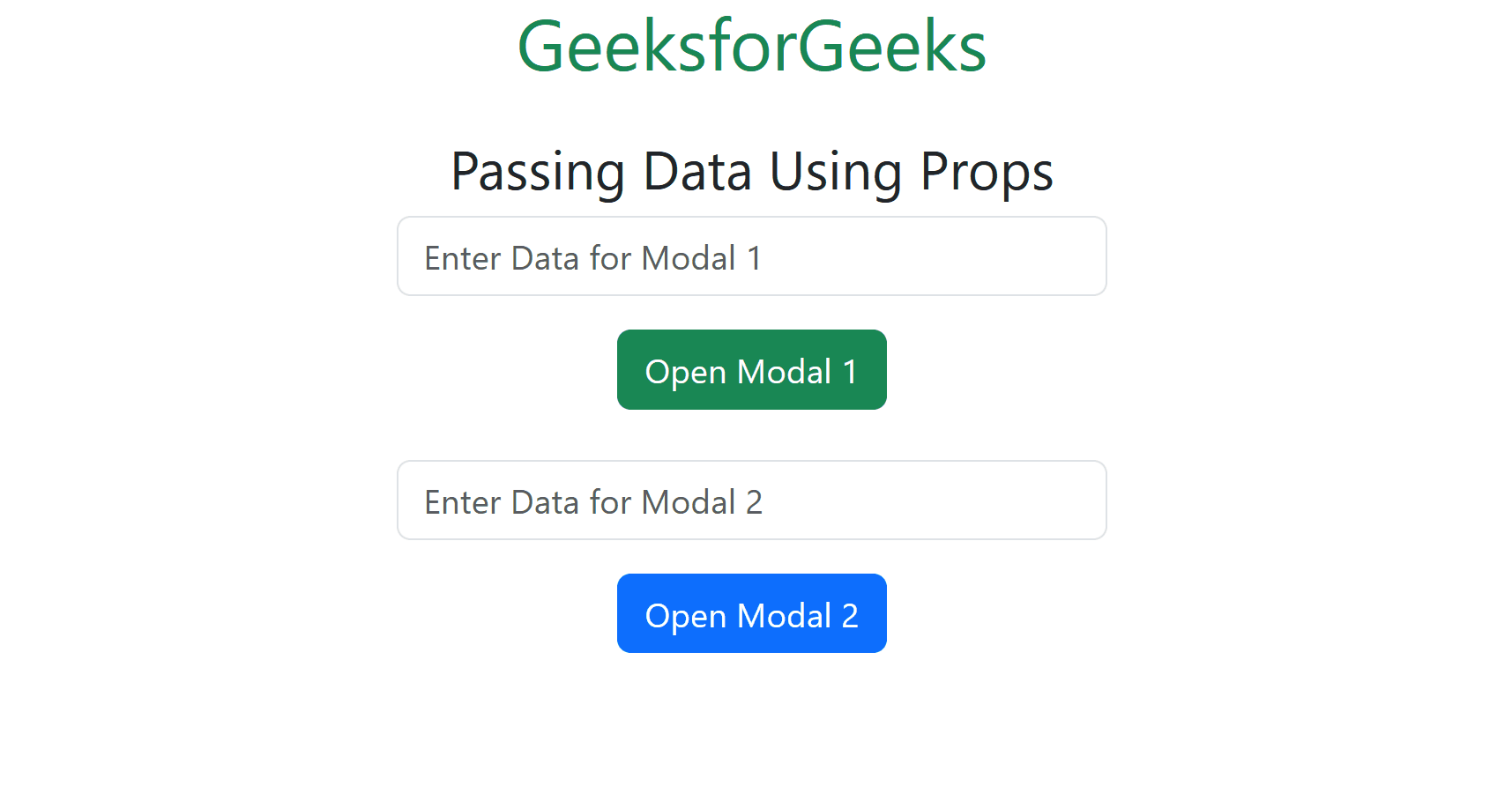
Share your thoughts in the comments
Please Login to comment...