How to create routes using Express and Postman?
Last Updated :
21 Nov, 2023
In this article we are going to implement different HTTP routes using Express JS and Postman. Server side routes are different endpoints of a application that are used to exchange data from client side to server side.
Express.js is a framework that works on top of Node.js server to simplify its APIs and add new features. It makes it easier to organize application’s functionality with middleware and routing. It adds helpful utilities to Node.js HTTP objects and allows easy rendering of dynamic HTTP objects.
Postman is an API(application programming interface) development tool which helps to build, test and modify APIs. Almost any functionality that could be needed by any developer is encapsulated in this tool. It has the ability to make various types of HTTP requests(GET, POST, PUT, PATCH), saving environments for later use, converting the API to code for various languages(like JavaScript, Python).
Prerequisites
Approach
We are establishing different HTTP routes using Express JS. First, we are establishing a server using an app. listen() method of express js Now in this application, we are going to perform endpoints for CRUD operations, which means create, read, update, and delete. There are different methods for each of them, like POST, GET, PUT, and DELETE, respectively. Now we are going to use these different methods in server-side code to setup routes.
We created different routes using app methods(), and to parse data, we applied the middleware express.json() create, read, update, delete. There are different methods for each of them like POST, GET, PUT, DELETE respectively. Now we are going to use these different methods in server side code to setup routes.
We created different routes using app methods() and to parse data we applied a middleware express.json().
After successful implementation of all endpoints we are testing them in postman.
Steps to create application and install required tools and modules
Step 1: Create a directory for backend via following command.
mkdir server
Step 2: Initiate Package.json through following command.
npm init -y
Step 3: Install express JS to setup routes.
npm i express body-parser
Step 4: Create a file for server side code.
touch server.js
Step 5: Download & install postman through given link.
https://www.postman.com/postman
To learn in depth explanation of how to install Postman on Windows, please refer to this How to Download and Install Postman on Windows? article.
Project Structure:
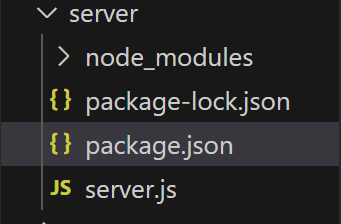
The updated dependencies in package.json file will look like:
"dependencies": {
"body-parser": "^1.20.2",
"express": "^4.18.2"
}
Example: Implementation of above approach.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use(express.json());
app.get( "/" , (req, res) => {
console.log( "GET Request Successfull!" );
res.send( "Get Req Successfully initiated" );
})
app.put( "/put" , (req, res) => {
console.log( "PUT REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data Update Request Recieved`);
})
app.post( "/post" , (req, res) => {
console.log( "POST REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data POSt Request Recieved`);
})
app. delete ( "/delete" , (req, res) => {
console.log( "DELETE REQUEST SUCCESSFUL" );
console.log(req.body);
res.send(`Data DELETE Request Recieved`);
})
app.listen(PORT, () => {
console.log(`Server established at ${PORT}`);
})
|
Steps to run application:
Step 1: Run application via given command.
node server
Step 2: Locate the given URL in postman with different HTTP methods.
Endpoints
localhost:3000/
localhost:3000/put
localhost:3000/post
localhost:3000/delete
Output:
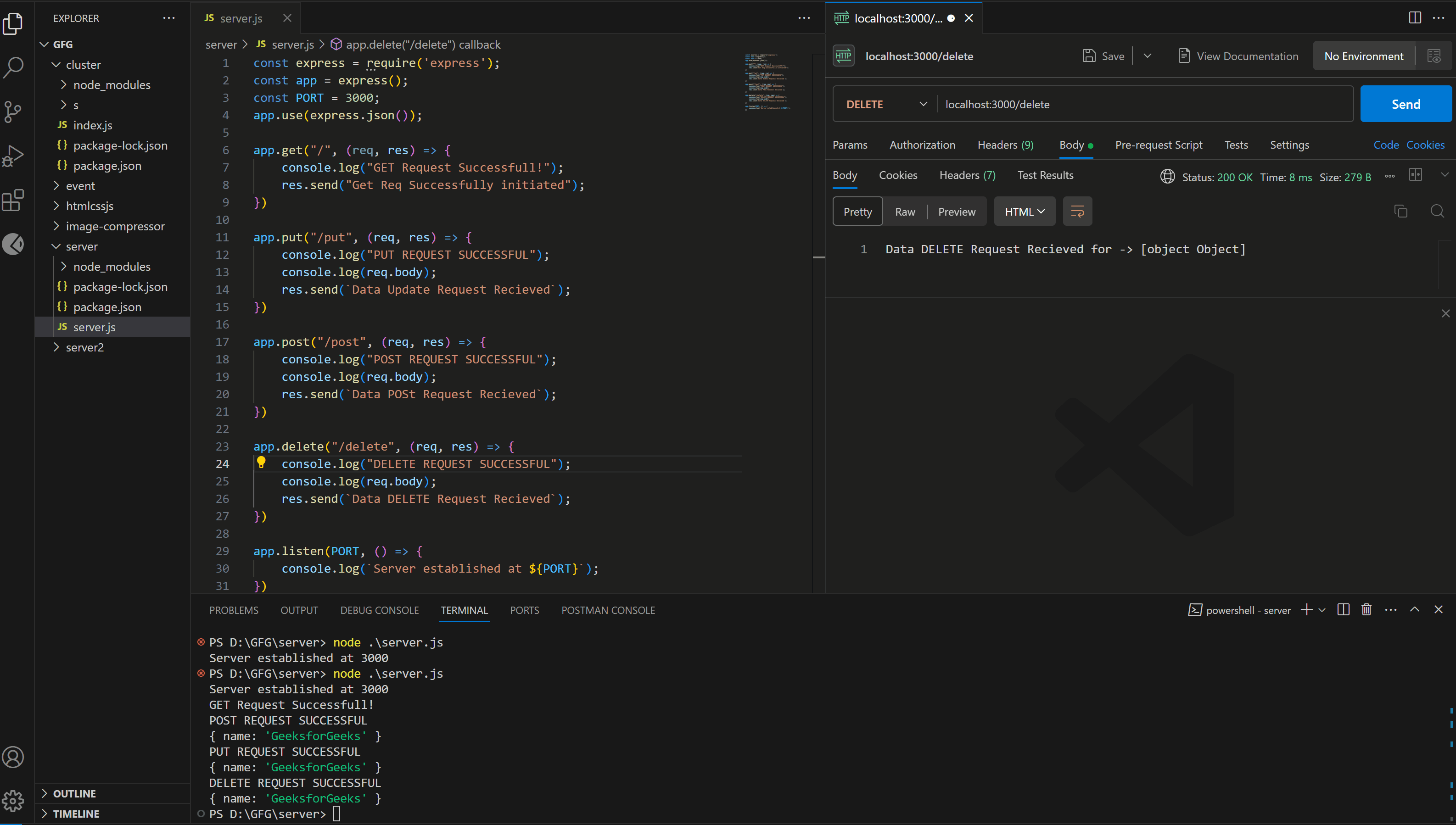
Share your thoughts in the comments
Please Login to comment...