React Redux Hooks: useSelector and useDispatch.
Last Updated :
19 Mar, 2024
State management is a major aspect of building React applications, allowing users to maintain and update application state predictably. With the introduction of React Hooks, managing state has become even more streamlined and efficient.
Among the most commonly used hooks for state management in React Redux are useSelector and useDispatch. In this article, we’ll explore how these hooks work and how they can simplify your React Redux development process.
Prerequisites
Understanding the concept of useSelector
useSelector is a hook provided by React Redux that allows functional components to extract and access data from the Redux store. It subscribes to the Redux store, and whenever the store is updated, the component re-renders to reflect the changes. Here’s a breakdown of how to use useSelector:
- Import the useSelector hook from the ‘react-redux’ library.
import {useSelector} from "react-redux";
- Call useSelector within your functional component, passing in a selector function.
const counter = useSelector(state => state.counter);
- The selector function defines which part of the Redux store state you want to extract and use within your component.
- useSelector returns the selected data from the Redux store, which you can then use within your component.
Understanding the concept of useDispatch
useDispatch is another hook provided by React Redux, which allows functional components to dispatch actions to the Redux store. It returns a reference to the dispatch function from the Redux store, enabling components to trigger state changes. Here’s how to use useDispatch:
- Import the useDispatch hook from the ‘react-redux’ library.
import { useDispatch } from "react-redux";
- Call useDispatch within your functional component to get a reference to the dispatch function.
const dispatch = useDispatch();
- Use the dispatch function to dispatch actions to the Redux store, which will update the state accordingly.
Example: Below is the example to show the concept of useSelector().
- Install the necessary package in your application using the following command.
npm install react-redux
Javascript
// App.jsx
import "./App.css";
import Example from "./components/Example";
function App() {
return (
<div className="App">
<h1>Counter Using useSelector</h1>
<Example/>
</div>
);
}
export default App;
Javascript
// actions.js
export const increment = () => {
return {
type: 'INCREMENT'
};
};
Javascript
// reducers.js
const initialState = {
counter: 0
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state,
counter: state.counter + 1
};
default:
return state;
}
};
export default rootReducer;
Javascript
// store.js
import { createStore } from 'redux';
import rootReducer from "../src/reducers/reducer"
const store = createStore(rootReducer);
export default store;
Javascript
// Example.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment } from '../actions/actions';
const Example = () => {
const counter = useSelector(state => state.counter);
const dispatch = useDispatch();
const handleIncrement = () => {
dispatch(increment());
};
return (
<div>
<p>Counter: {counter}</p>
<button onClick={handleIncrement}>Increment</button>
</div>
);
};
export default Example;
Start your application using the following command.
npm start
Output:
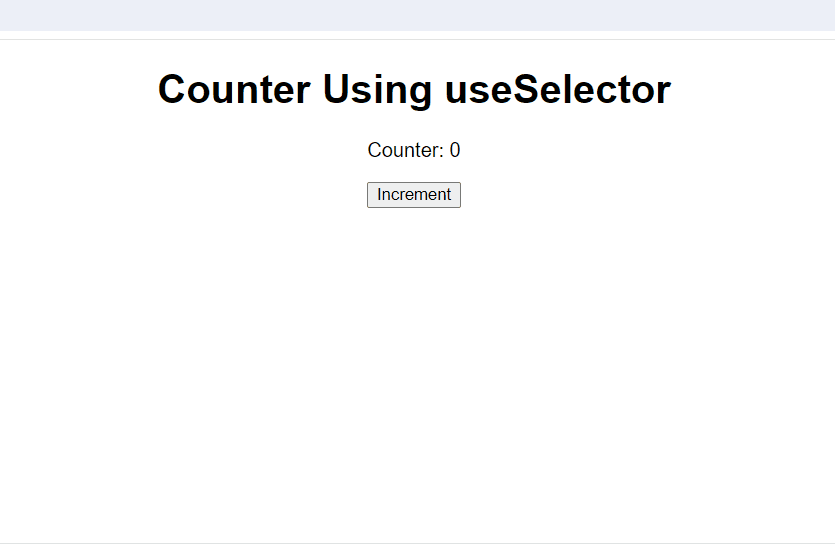
Output
Advantages of useSelector and useDispatch
- Simplified Syntax: The hooks provide a more concise and cleaner syntax compared to traditional methods of connecting components to the Redux store, such as using
connect
in class components. - Functional Components: Since hooks are designed for functional components, they align well with the modern React paradigm. This allows developers to write more functional and composable code.
- Better Performance: The
useSelector
hook automatically subscribes the component to the Redux store, but it only re-renders when the selected state value it depends on changes. This can lead to better performance by preventing unnecessary re-renders. - Improved Code Organization: Using hooks allows you to colocate your Redux-related logic directly within your functional components, making it easier to understand and maintain.
Disadvantges of useSelector and useDispatch
- Learning Curve: Switching from older methods to hooks might take some time to get used to, especially for developers who are not familiar with React hooks. It may require learning new concepts and patterns.
- Dependency on Hooks: Hooks are a newer feature in React, so your codebase becomes dependent on them. If you need to support older versions of React or if hooks change in the future, it could impact your codebase’s compatibility.
- Redux Coupling: While hooks provide a cleaner way to access Redux state, they still tightly connect your components to the Redux store. This might make it harder to refactor or reuse components independently from Redux.
- Performance Concerns: While hooks are optimized to only re-render when necessary, incorrect usage or excessive re-renders can still affect performance. users need to be careful, especially in complex applications.
Share your thoughts in the comments
Please Login to comment...