Difference between PUT and POST HTTP Request in Express and Postman
Last Updated :
13 Jan, 2024
Both PUT and POST are request methods used in the HTTP protocol. In this article, we will introduce the HTTP methods such as PUT and POST in detail, and we will also discuss in detail when to use PUT and when to use POST.
PUT method
PUT is one of the HTTP request methods and is used to create or update a resource at the specified URI location. In other words, the PUT method essentially saves the body content sent in the request as a resource at the location of the URI path. RESTful APIs often use PUT to update resources.
Features of the PUT method
- The request URI is used as the resource identifier
- The request body contains the entire updated resource
- It has idempotency – repeating the same request yields the same result
- If the existing resource does not exist, a new one will be created
- If an existing resource exists, it will be completely replaced with the contents of the body
Example: Write the code example here having sample data that will be updated.
Javascript
const express = require( "express" );
const app = express();
app.use(express.json());
let users = [
{ id: 1, name: "John Doe" , email: "john.doe@example.com" },
{ id: 2, name: "Jane Smith" , email: "jane.smith@example.com" },
];
app.put( "/users/:id" , (req, res) => {
const id = parseInt(req.params.id);
const { name, email } = req.body;
let userFound = false ;
users = users.map((user) => {
if (user.id === id) {
userFound = true ;
return { ...user, name, email };
}
return user;
});
if (userFound) {
res.status(200).json({ message: "User updated successfully" , users });
} else {
res.status(404).json({ message: "User not found" });
}
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
|
Output:
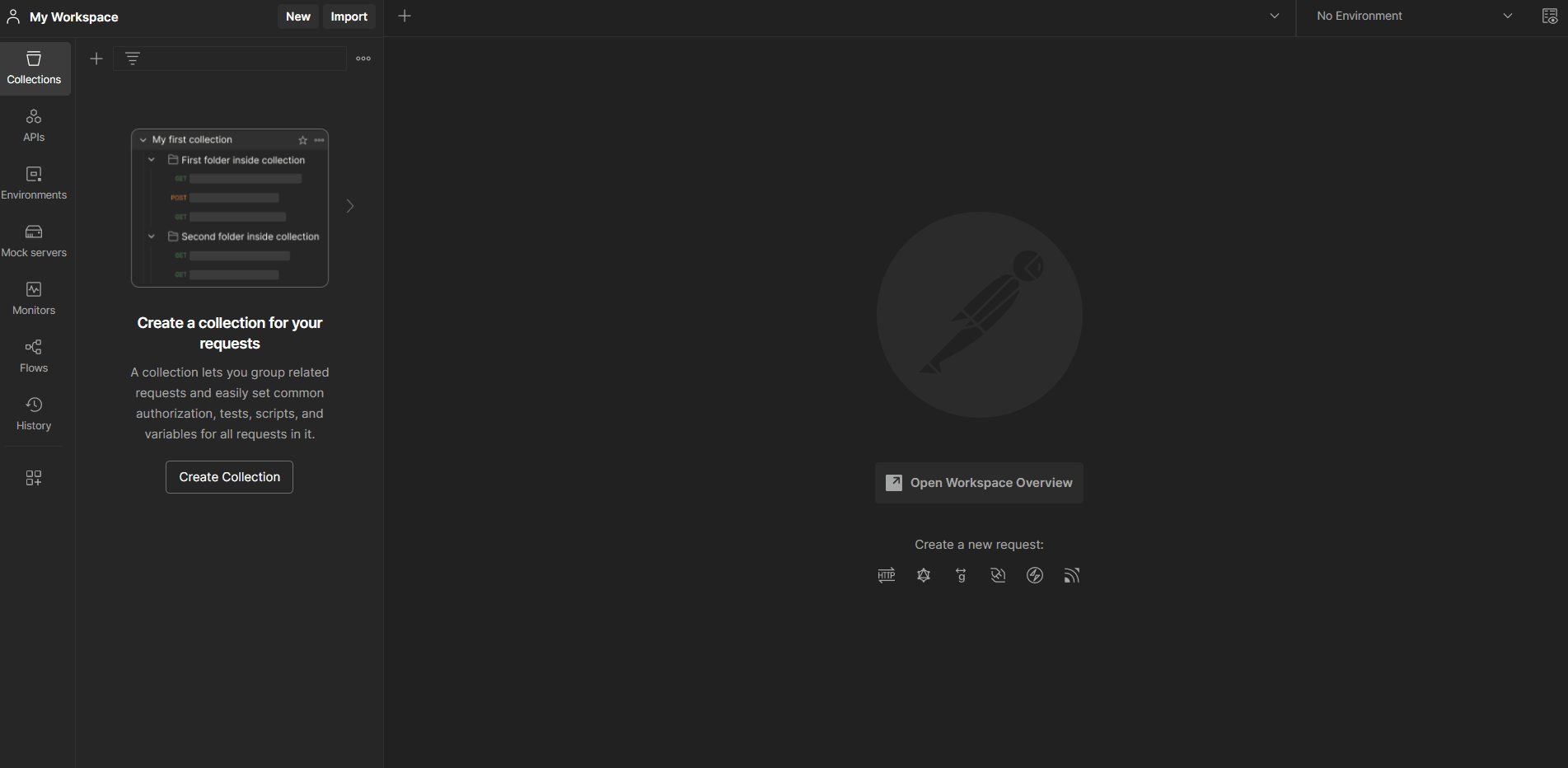
Advantages of PUT Method
- It helps you to store the supplied entity under the supplied URI.
- If the supplied entity already exists, then you can perform the update operation, or you can create with that URI.
- You can create a resource as many times as you like.
- Creating a resource with PUT method is very easy.
- You do not need to check whether the user has clicked the submit button multiple times or not.
- It can identify the entity enclosed with the request.
POST Method
POST is one of the HTTP request methods which is used to submit data to be processed to a specified resource. When you make a POST request, you’re typically sending data to the server in the body of the request, and the server processes that data based on the resource identified in the URL.
Unlike PUT, POST is not idempotent, meaning duplicate requests can produce different outcomes. The URI points to the processing application rather than the resource itself. POST can support empty request bodies and creation of more than one resource type.
Features of the POST method
- The URI indicates the location of the resource that will handle the request
- The request body contains data for creating the new resource
- It does not have idempotency – repeating the same request may produce different results
- Often used to create new resources
- An empty request body may still be valid
Example:
Javascript
const express = require( 'express' );
const app = express();
app.use(express.json());
let users = [
{ id: 1, name: 'John Doe' , email: 'john.doe@example.com' },
{ id: 2, name: 'Jane Smith' , email: 'jane.smith@example.com' }
];
app.post( '/users' , (req, res) => {
const { name, email } = req.body;
const newUser = {
id: users.length + 1,
name,
email
};
users.push(newUser);
res.status(201).json({ message: 'User created successfully' , newUser });
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
|
Output :
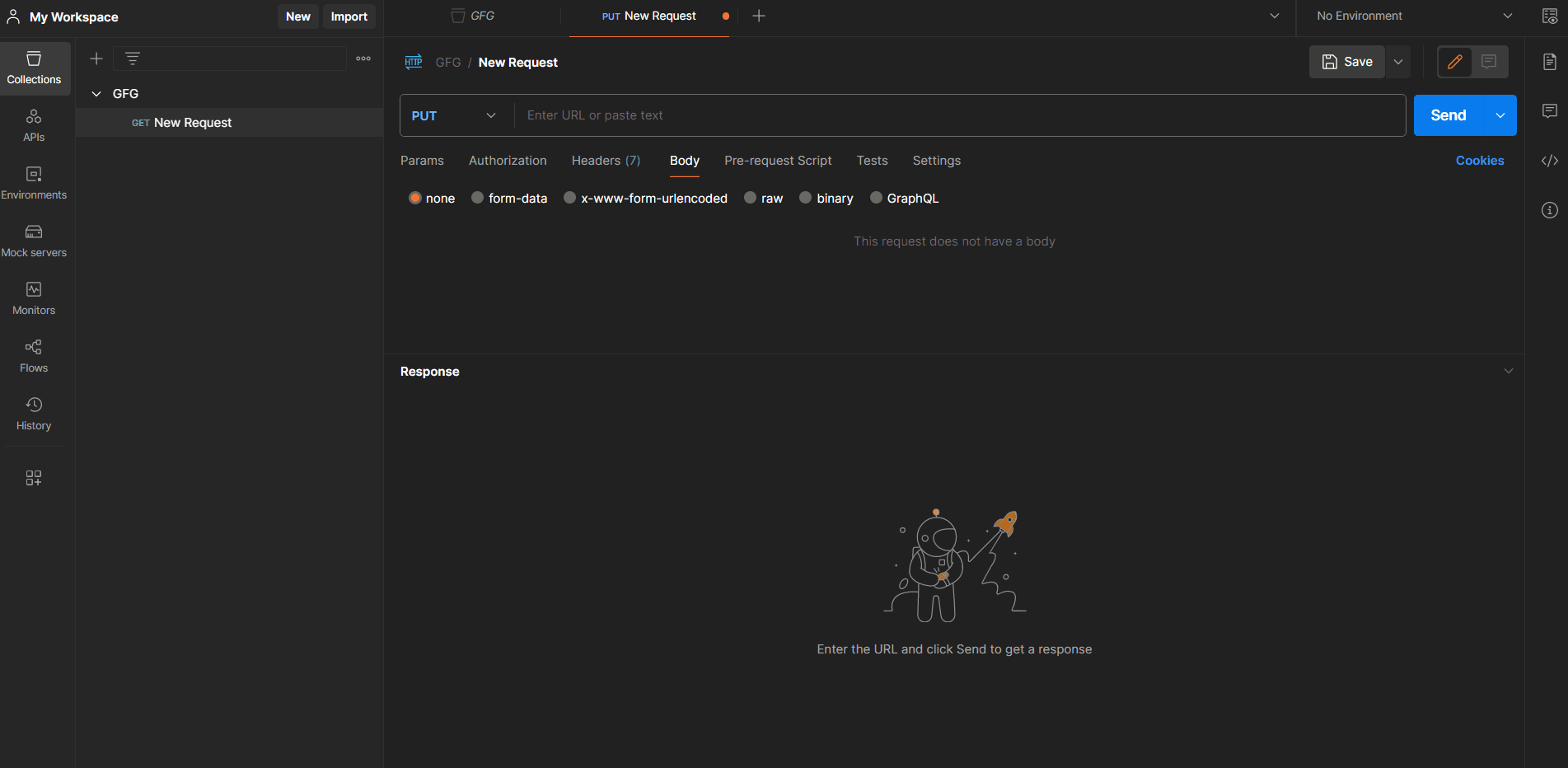
Advantages of POST Method
- This method helps you to determine resource URI.
- Specifying a new resource location header is very easy using location header.
- You can send a request to accept the entity as a new subordinate of the resource, which is identified by the URI.
- You can send user-generated data to the web server.
- It is very useful when you do not know URL to keep any resource.
- Use POST when you need the server, which controls URL generation of your resources.
- POST is a secure method as its requests do not remain in browser history.
- You can effortlessly transmit a large amount of data using post.
- You can keep the data private.
- This method can be used to send binary as well as ASCII data.
Difference between PUT and POST method
This method is idempotent.
|
This method is not idempotent.
|
PUT method is call when you have to modify a single resource, which is already a part of resource collection.
|
POST method is call when you have to add a child resource under resources collection.
|
RFC-2616 depicts that the PUT method sends a request for an enclosed entity stored in the supplied request URI.
|
This method requests the server to accept the entity which is enclosed in the request.
|
PUT method syntax is PUT /questions/{question-id}
|
POST method syntax is POST /questions
|
You can not cache PUT method responses.
|
POST method answer can be cached.
|
PUT /vi/juice/orders/1234 indicates that you are updating a resource which is identified by “1234”.
|
POST /vi/juice/orders indicates that you are creating a new resource and return an identifier to describe the resource.
|
If you send the same request multiple times, the result will remain the same.
|
If you send the same POST request more than one time, you will receive different results.
|
PUT works as specific.
|
POST work as abstract.
|
We use UPDATE query in PUT.
|
We use create query in POST.
|
In PUT method, the client decides which URI resource should have.
|
In POST method, the server decides which URI resource should have.
|
Conclusion
PUT and POST are similar in that they are both REST API requests, and they both modify data, but they differ in what they are used for, and how they modify data. Use PUT to modify existing data and POST to add a new record. Remember that using the same POST request repeatedly can have unintended consequences because it is non-idempotent, while using the same PUT request repeatedly will have the same effect.
Share your thoughts in the comments
Please Login to comment...