How to test DELETE Method of Express with Postman ?
Last Updated :
11 Jan, 2024
The DELETE method in web development plays a crucial role in handling resource deletions on the server. As one of the HTTP methods, its primary purpose is to request the removal of a resource identified by a specific URI. In this article, we will see how we can use the Delete method in different scenarios efficiently.
Prerequisites:
We will use the following approaches to test delete method:
Approach 1: Direct Usage in Express JS:
In this approach, we leverage Express JS to handle nested routes and implement the DELETE method, providing a clear and straightforward organization of code.
Syntax:
app.delete('base URL', (req, res) => {
// DELETE method logic for the base URL
})
app.delete('base URL/Nested URL', (req, res) => {
// DELETE method logic for the nested URL
})
Example: Write the following code in server.js file.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app. delete ( "/resource" , (req, res) => {
console.log( "DELETE Request Successful!" );
res.send( "Resource Deleted Successfully" );
});
app. delete ( "/resource/:id" , (req, res) => {
const resourceId = req.params.id;
console.log(`Deleting resource with ID: ${resourceId}`);
res.send(`Resource with ID ${resourceId} Deleted Successfully`);
});
app.listen(PORT, () => {
console.log(`Server established at ${PORT}`);
});
|
Approach 2: Usage with Express JS Router:
This approach involves creating a base URL using Express JS Router, allowing for a cleaner structure and improved code organization.
Syntax:
const router = express.Router();
router.delete('/', (req, res) => {
// DELETE method logic for the base URL
})
router.delete('/nested', (req, res) => {
// DELETE method logic for the nested URL
})
Example: Write the following code in server.js file.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
const router = express.Router();
router. delete ( '/' , (req, res) => {
res.send( "Deleting Resource" );
});
router. delete ( '/api' , (req, res) => {
res.send( "Deleting from /api endpoint" );
});
app.use( '/resource' , router);
app.listen(PORT, () => {
console.log(`Server established at ${PORT}`);
});
|
Implementation:
In this example, we’ll create a basic API for managing a list of books.
Step 1: Initialize a Express Js project and install the required dependencies.
mkdir express-delete-example
cd express-delete-example
npm init -y
npm install express
Project Structure:
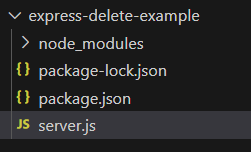
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Step 2: Create the main server file (e.g., server.js)
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use(express.json());
let books = [
{ id: 1, title: 'Introduction to Express' , author: 'John Doe' },
{ id: 2, title: 'Node.js Basics' , author: 'Jane Smith' },
];
app.get( '/books' , (req, res) => {
res.json(books);
});
app.post( '/books' , (req, res) => {
const newBook = req.body;
books.push(newBook);
res.status(201).json(newBook);
});
app. delete ( '/books/:id' , (req, res) => {
const bookId = parseInt(req.params.id);
const index = books.findIndex(book => book.id === bookId);
if (index !== -1) {
const deletedBook = books.splice(index, 1)[0];
res.json({ message: 'Book deleted successfully' , deletedBook });
} else {
res.status(404).json({ message: 'Book not found' });
}
});
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
To start the server run the following command.
node server.js
Step 3: You can test the DELETE method using Postman, for testing using postman:
- login to your postman account.
- Create New request and paste the url i.e http://localhost:3000/books/1 in request.
- Choose Delete from the methods section.
- click on SEND.
- Now, you can see the Output in the Postman.
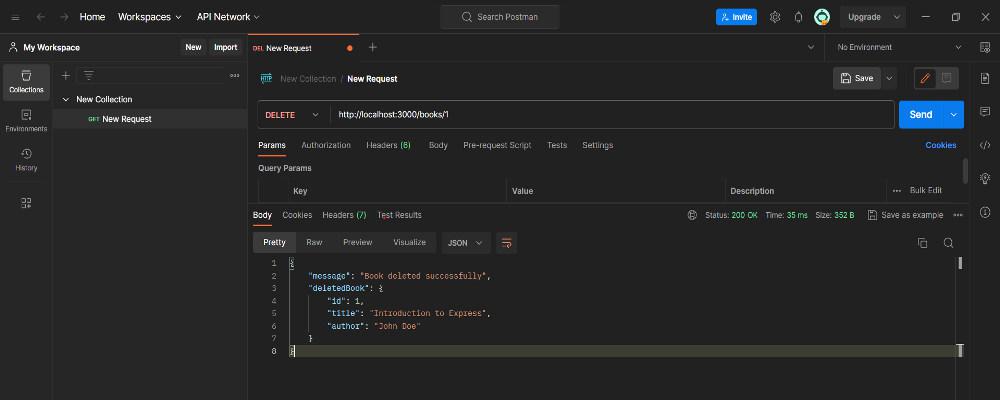
Output:.gif)
Share your thoughts in the comments
Please Login to comment...