How to use postman for testing express application
Last Updated :
20 Dec, 2023
Testing an Express app is very important to ensure its capability and reliability in different use cases. There are many options available like Thunder client, PAW, etc but we will use Postman here for the testing of the Express application. It provides a great user interface and numerous tools which makes API testing very easy.
Postman is an API(utility programming interface) development device that enables construction, takes a look at, and alters APIs. It could make numerous varieties of HTTP requests(GET, POST, PUT, PATCH), store environments for later use, and convert the API to code for various languages(like JavaScript, and Python). In this article, we will learn How to use postman for testing express application
Prerequisites:
Steps to create Express Application and Postman testing
Step 1: Create a directory in which the express application will store
mkdir express-postman
cd express-postman
Step 2: Initialize the project with the following command
npm init -y
Step 3: Install the required dependencies.
npm i express nodemon mongoose
Project Structure:
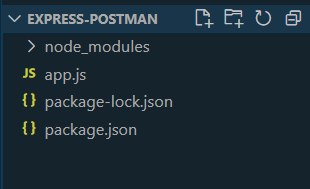
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"mongoose": "^8.0.3",
"nodemon": "^3.0.2"
}
Example: Creating a simple Express application for POST and GET userData.
Javascript
const express = require( "express" );
const mongoose = require( "mongoose" );
const app = express();
app.use(express.json());
mongoose.connect(
"Your connection string" ,
{
useNewUrlParser: true ,
useUnifiedTopology: true ,
}
);
const userSchema = new mongoose.Schema({
name: String,
email: String,
mobile: Number,
});
const User = mongoose.model( "Userdata" , userSchema);
app.get( "/" , (req, res) => {
res.send( "API testing" );
});
app.post( "/users" , async (req, res) => {
try {
const { name, email, mobile } = req.body;
const newUser = new User({ name, email, mobile });
await newUser.save();
res.status(201).json(newUser);
} catch (error) {
res.status(500).json({ message: error.message });
}
});
app.get( "/users" , async (req, res) => {
try {
const users = await User.find();
res.json(users);
} catch (error) {
res.status(500).json({ message: error.message });
}
});
app.listen(3000, () => {
console.log( "App is running on port 3000" );
});
|
Steps to run the application:
nodemon app.js
Output:
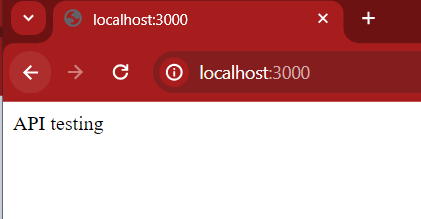
Browser Output.
API testing with Postman
Step 1: Open the Postman App and create a new collection by using the “+” icon -> Blank Collection.
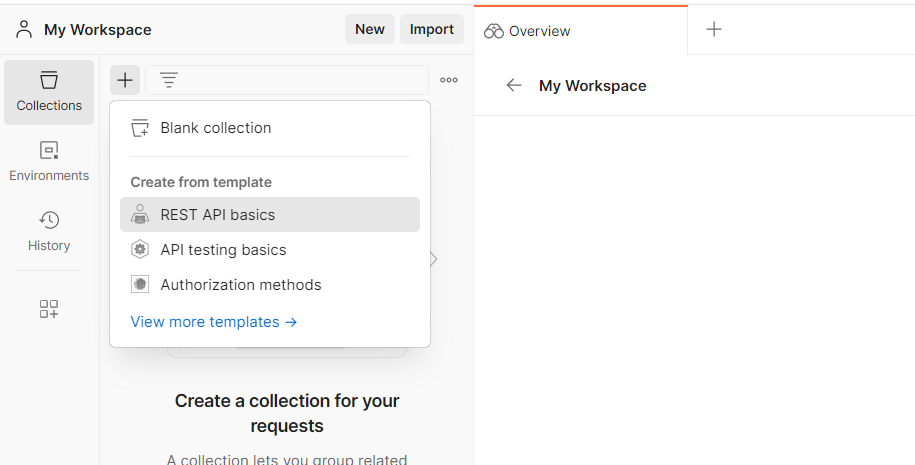
Step 2: Add a new request by clicking on the 3 dots on the collection then click on the “Add request” option.
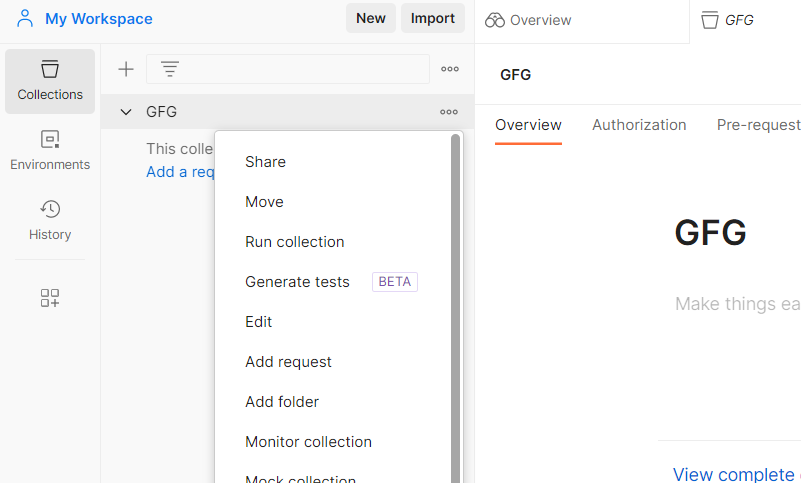
Step 3: Now make a POST request to add data in the MongoDB collection. Add 3-4 sample data using this method.
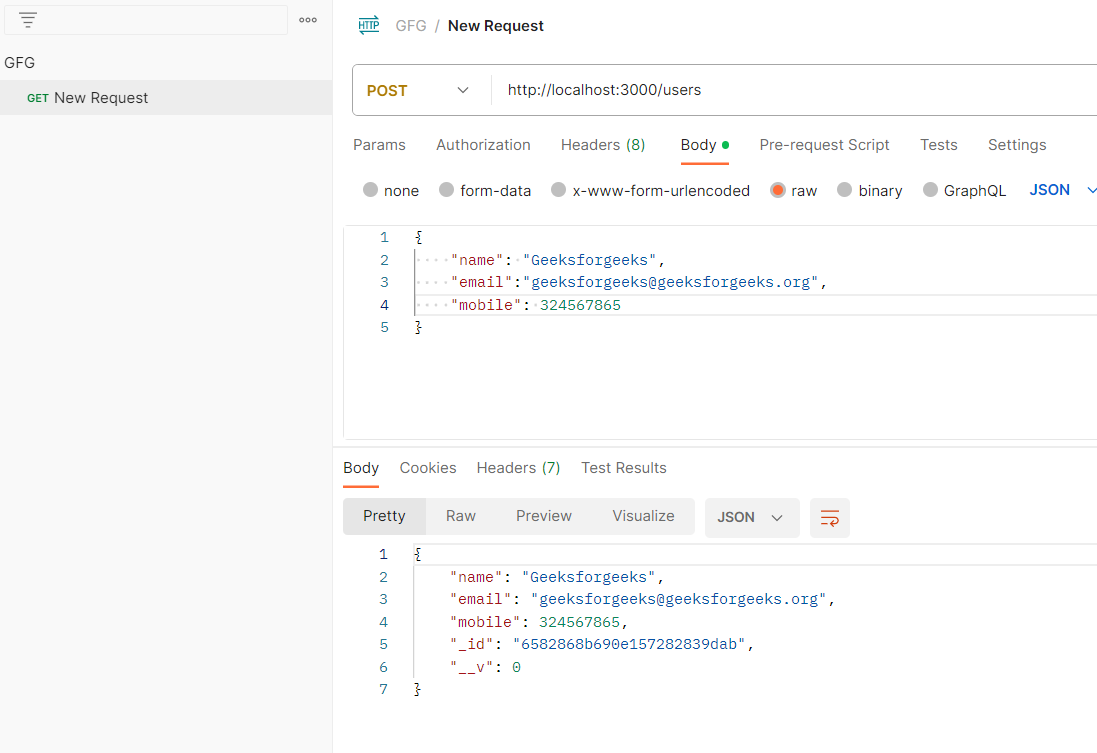
POST method
Step 4: Make a GET request in the API to get all the user data.
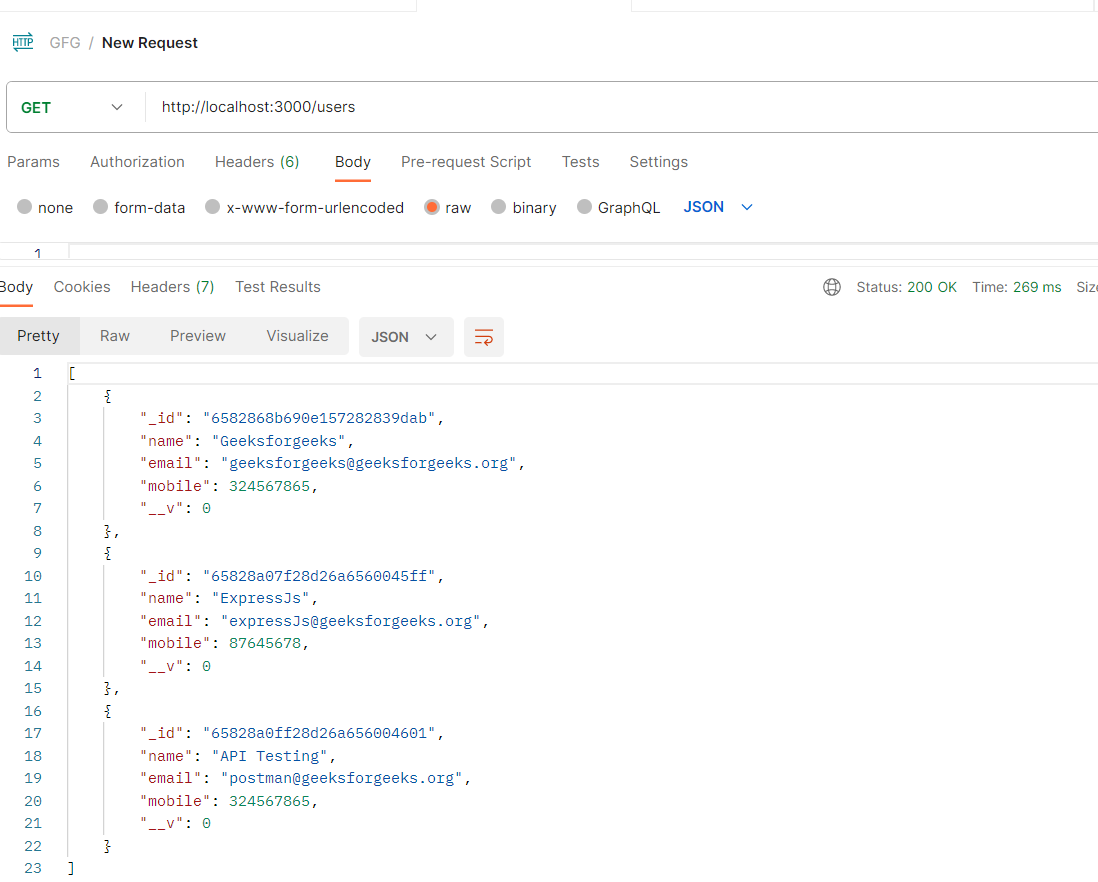
GET request
Step 5: Now we will use ‘Tests’ tool of Postman to test the data available in our collection. We will check few conditions to verify the data stored in the collection. You can also use test scripts from Snippet section available on the right part.
- Test to check if the response is successful (status code 200)
- Test to check if the response body is a JSON array
- Test to check if each object in the response array has name, email, and mobile fields
Javascript
pm.test( "Status code is 200" , function () {
pm.response.to.have.status(200);
});
pm.test( "Response is a JSON array" , function () {
pm.response.to.be.json;
pm.response.to.be.ok;
pm.expect(pm.response.json()).to.be.an( "array" );
});
pm.test( "Users have required fields" , function () {
const users = pm.response.json();
pm.expect(users).to.be.an( "array" ).that.is.not.empty;
users.forEach( function (user) {
pm.expect(user).to.have.property( "name" );
pm.expect(user).to.have.property( "email" );
pm.expect(user).to.have.property( "mobile" );
});
});
|
Output:
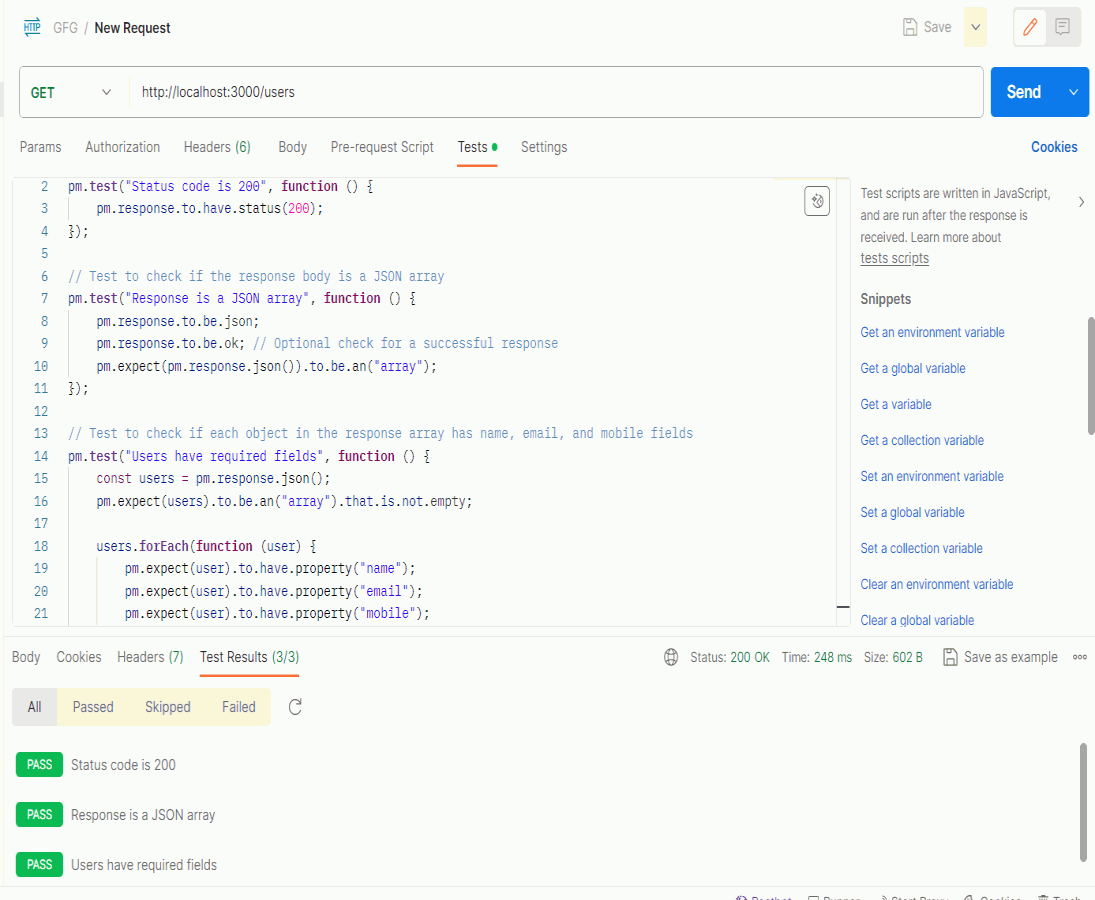
Tests
Share your thoughts in the comments
Please Login to comment...