How to enable file downloads from Express Routes ?
Last Updated :
14 Jan, 2024
In web development, lets users to download files from the server through an Express route is a common requirement. Express provides straightforward methods to do this process efficiently. In this article, you will see how can you enable file downloads from an Express route.
Prerequisites:
Approach to enable file download:
- For fulfilling the requirement we will use res.download() method. It handles headers and triggers a download prompt in the user’s browser.
- We will create a dynamic API path that can be used to download the specific file.
- We will use the path module to successfully merge path directory and file name.
- After that, we will use res.download method to let the process complete.
- We will also handle the errors in this method.
Steps to create Application:
Step 1: Create a Node project using following command and install express:
npm init -y
npm install express
Step 2: Create a folder named files in your current directory and move a file which you want to download inside it.
Project Structure:
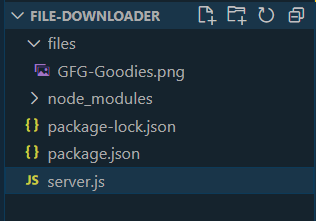
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Example: Create an app.js where we’ll implement the downloading functionality. Insert the below code in it:
Javascript
const express = require( 'express' );
const path = require( 'path' );
const app = express();
app.get( '/download/:file' , (req, res) => {
const filePath = path.join(__dirname, 'files' , req.params.file);
res.download(filePath, (err) => {
if (err) {
res.status(404).send( 'File not found' );
}
});
});
app.use(express.static(path.join(__dirname, 'public' )));
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
|
Step 3: To run this example, run the following command:
node app.js
Step 4: Open http://localhost:3000/download/<file_name> and you will get an option to download the file.
Output:
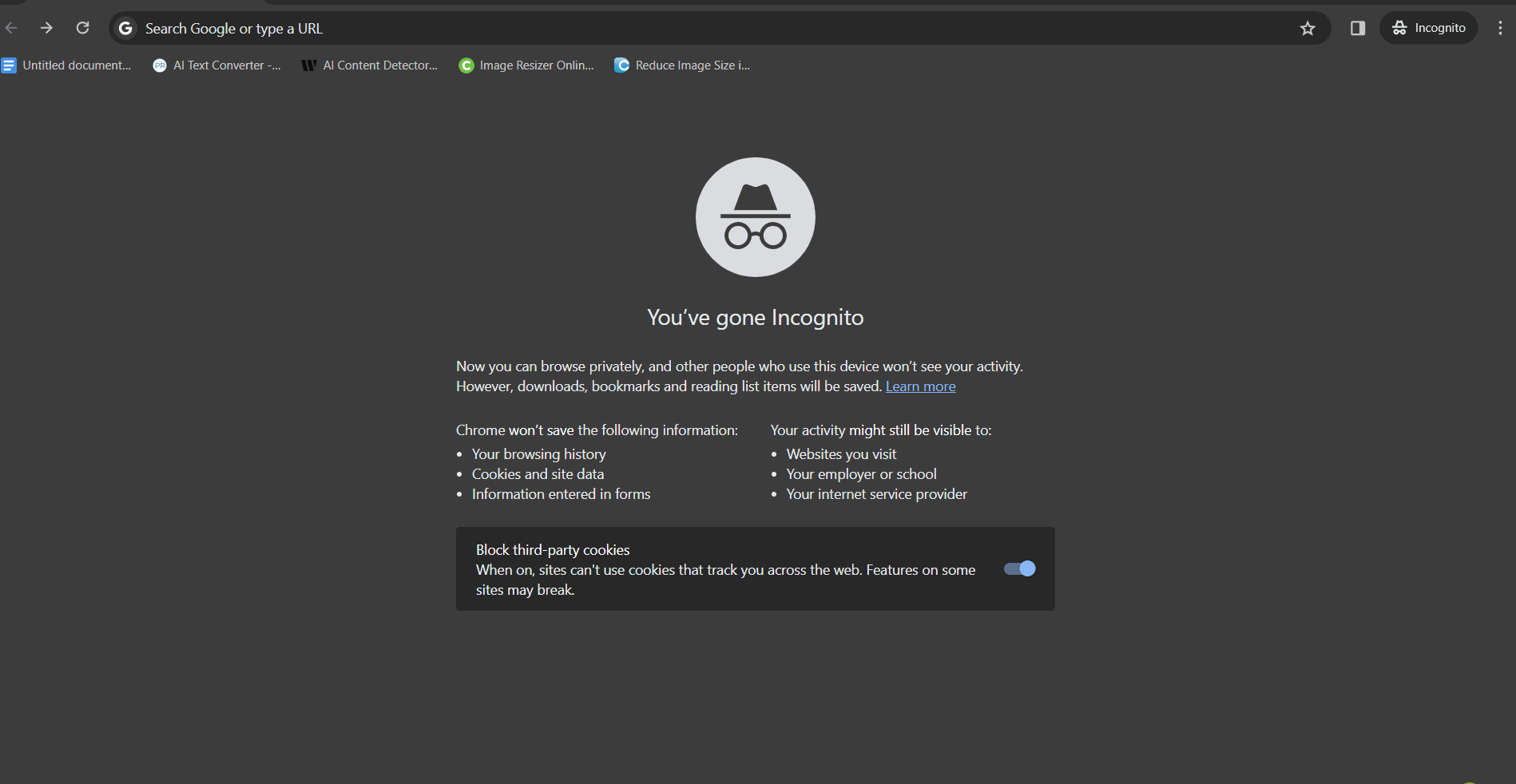
Final Output
Share your thoughts in the comments
Please Login to comment...