What are Action’s creators in React Redux?
Last Updated :
06 Feb, 2024
In React Redux, action creators are functions that create and return action objects. An action object is a plain JavaScript object that describes a change that should be made to the application’s state. Action creators help organize and centralize the logic for creating these action objects.
Action Creators:
- Messengers with a Purpose: Action creators are like messengers in your app. They’re responsible for delivering a message about what should happen next.
- Instructions for Change: When something in your app needs to change, an action creator steps in to create a clear set of instructions, known as an “action.”
- Decide and Describe: Action creators help you decide what action to take and describe it in a way that your app can understand.
- Easy Communication: They make communication between different parts of your app smoother by providing a standardized way to express intentions.
- Initiating Change: Action creators as the ones who kickstart the process when your app needs to do something new or update information.
Action’s creators in React Redux:
- Purpose: Action creators simplify creating and dispatching actions in React Redux.
- Action Object: They generate action objects, which describe changes in the application.
- Simplifies Dispatch: Action creators make it easier to dispatch actions without manually crafting every action object.
- Improves Maintainability: Centralizing action creation in action creators enhances code maintainability, especially when action structures change.
- Encourages Consistency: Action creators promote a standardized approach to action creation and dispatching throughout the application.
Example : Below are the example of Action’s creators in React Redux.
- Install the following packages in your react application using the following command.
npm install react-redux
npm install redux
- Create the folder inside the src folder i.e. actions, reducers and constants.
- Inside the actions folder create file name todeAction.js.
- Inside the reducers folder create file name todoReducer.js.
- Inside the constants folder create file name TodoActionTypes.js.
- In the src folder create store.js file.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import { Provider } from 'react-redux' ;
import { createStore } from 'redux' ;
import App from './App' ;
import todoReducer from './reducers/todoReducer' ;
const store = createStore(todoReducer);
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById( 'root' )
);
|
Javascript
import React from "react" ;
import "./App.css" ;
import TodoList from "./components/TodoList" ;
const App = () => {
return (
<>
<TodoList/>
</>
);
};
export default App;
|
Javascript
export const ADD_TODO = 'ADD_TODO' ;
export const REMOVE_TODO = 'REMOVE_TODO' ;
|
Javascript
import { ADD_TODO, REMOVE_TODO }
from "../constants/TodoActionTypes" ;
const initialState = {
todos: []
};
const todoReducer = (state = initialState, action) => {
switch (action.type) {
case ADD_TODO:
return {
...state,
todos: [...state.todos,
{ id: Date.now(), text: action.payload.text }]
};
case REMOVE_TODO:
return {
...state,
todos: state.todos.filter
(todo => todo.id !== action.payload.id)
};
default :
return state;
}
};
export default todoReducer;
|
Javascript
import { ADD_TODO, REMOVE_TODO }
from "../constants/TodoActionTypes" ;
export const addTodo = (text) => ({
type: ADD_TODO,
payload: { text }
});
export const removeTodo = (id) => ({
type: REMOVE_TODO,
payload: { id }
});
|
Javascript
import React from 'react' ;
import { useDispatch, useSelector }
from 'react-redux' ;
import { addTodo, removeTodo }
from '../actions/todoActions' ;
import './TodoList.css' ;
const TodoList = () => {
const dispatch = useDispatch();
const todos = useSelector(state => state.todos);
const handleAddTodo = () => {
const text = prompt( 'Enter todo:' );
if (text) {
dispatch(addTodo(text));
}
};
const handleRemoveTodo = (id) => {
dispatch(removeTodo(id));
};
return (
<div className= "container" >
<div className= "todo-list" >
<h1>To-Do List</h1>
<button onClick={handleAddTodo}>
Add Todo
</button>
<ul>
{todos.map(todo => (
<li key={todo.id}>
{todo.text}
<button onClick={() =>
handleRemoveTodo(todo.id)}>
Remove
</button>
</li>
))}
</ul>
</div>
</div>
);
};
export default TodoList;
|
Javascript
import { createStore } from 'redux' ;
import todoReducer from './reducers/todoReducer' ;
const store = createStore(todoReducer);
export default store;
|
CSS
.container {
display : flex;
justify- content : center ;
align-items: center ;
height : 100 vh;
}
.todo-list {
max-width : 400px ;
width : 100% ;
}
button {
margin-top : 5px ;
background-color : blueviolet;
color : white ;
padding : 5px ;
border : 2px solid grey;
border-radius: 5px ;
}
|
Start your application using the following command.
npm start
Output:
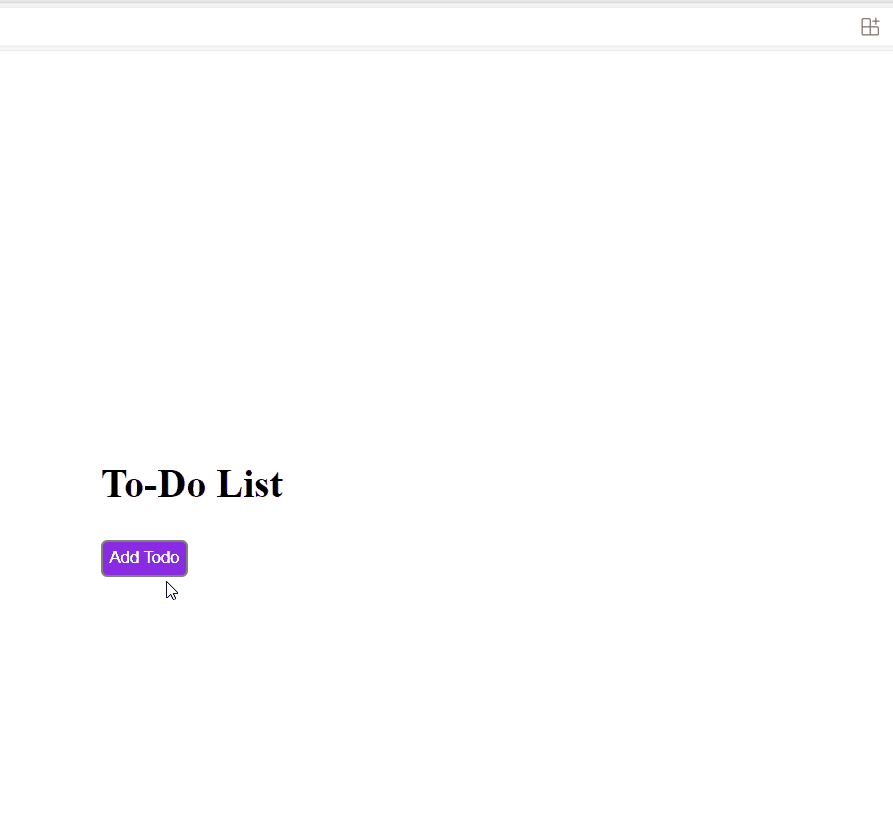
Output
Share your thoughts in the comments
Please Login to comment...