Difference between application level and router level middleware in Express?
Last Updated :
09 Jan, 2024
If you’ve used Express even a little, you know how important middleware is. Those little functions run on every request and let you do stuff like logging, authentication, file uploads, and more. In this article, we will see what is Application-level middleware and Router-level middleware and what are the key differences between them.
Application-Level Middleware:
Application-level middleware is like a personal assistant that helps out with every incoming request to your Express app. No matter which route a client hits, this middleware jumps in to work its magic. For example, you can use app-level middleware to log every request that comes in for debugging. Or to check authentication for your entire application. Or even set global headers that you want on every response.
Syntax:
app.use(params...);
Key Features:
- Global Scope: It operates on all routes within the application.
- Initialization Order: It’s defined before any specific route handlers.
- Common Use Cases: Logging, setting headers, authentication checks, error handling, and serving static files.
Example:
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use((req, res, next) => {
console.log(`Request received at: ${ new Date()}`);
next();
});
app.use(express.json());
app.get( '/' , (req, res) => {
res.send( 'Hello from the root route!' );
});
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});
|
Output:
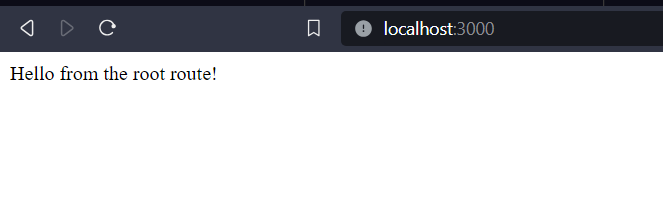
Console Output:

Router-Level Middleware:
Router-level middleware is more specialized – it only applies to particular routing paths you choose. Think of it like a special helper that comes in to handle requests for certain routes. For example, you could set up some router-level middleware that checks for authentication on your /account routes or validation middlewares that check data before your /purchase or /signup routes.
Syntax:
router.use(params...);
Key Features:
- Specific Scope: It operates on specific routes or groups of routes.
- Initialization Order: It’s defined closer to route handlers, affecting only those routes.
- Common Use Cases: Route-specific authentication, input validation, handling specific routes’ behaviors.
Example:
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
const router = express.Router();
router.use((req, res, next) => {
console.log(`Middleware specific to this router`);
next();
});
router.get( '/route' , (req, res) => {
res.send( 'Response from the router-level route' );
});
app.use( '/api' , router);
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});
|
Output:

Console Output:

Difference between Application and Router level Middleware:
Application middleware is better for global concerns like logging, while router middleware is better for things you only want to apply to certain routes, like authentication.
Where it’s applied ?
|
Applies globally to the whole app.
|
Applied to a router.
|
How it’s applied ?
|
App.use() or app.METHOD().
|
Router.use() or router.METHOD()
|
Paths affected
|
Affects all routes and paths.
|
Only affects routes defined in router.
|
Common uses
|
Logging, authentication, error handling
|
Authentication for parts of app validation.
|
Share your thoughts in the comments
Please Login to comment...