Chakra UI Overlay Modal
Last Updated :
04 Feb, 2024
Chakra UI Overlay Modal is a component used to create modal dialogs in React applications. It is part of the Chakra UI library, offering a set of components for building consistent and accessible user interfaces. This component is used for creating interactive and visually appealing modal dialogs. In this article, we will see the practical demonstration of the Chakra UI Overlay Modal in terms of example and output.
Prerequisites:
Approach:
We have created the Chakra UI Overlay Modal component with the props of isOpen, onClose and size. We can also customize the Modal component by adding styling properties to it like color, fontSize, mt, etc. This component opens the dialog with the user clicks on the modal button displayed on the screen.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app my-app
Step 2: After creating your project folder(i.e. my-app), move to it by using the following command:
cd chakra
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
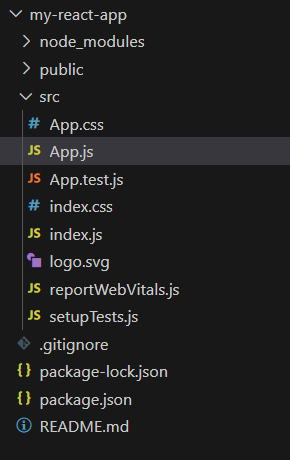
The updated dependencies are in the package.json file.
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is the practical implementation of the Chakra UI Overlay Modal.
Javascript
import React from 'react' ;
import {
ChakraProvider,
CSSReset,
Box,
Button,
Modal,
ModalOverlay,
ModalContent,
ModalHeader,
ModalCloseButton,
ModalBody,
ModalFooter,
useDisclosure,
Heading,
} from '@chakra-ui/react' ;
function App() {
const {
isOpen, onOpen,
onClose
} = useDisclosure();
return (
<ChakraProvider>
<CSSReset />
<Box p={5}>
<Button onClick={onOpen}>
Open GeeksforGeeks
Courses Modal
</Button>
<Modal isOpen={isOpen}
onClose={onClose} size= "lg" >
<ModalOverlay />
<ModalContent borderRadius= "md" >
<ModalHeader>
<Heading as= "h1" color= "green.500" >
GeeksforGeeks
</Heading>
<Heading as= "h3" fontSize= "xl"
mt={2} color= "gray.500" >
Chakra UI Overlay Modal
</Heading>
</ModalHeader>
<ModalCloseButton />
<ModalBody>
<p>
Explore a variety of courses
on GeeksforGeeks to enhance your
skills in JavaScript, React, and
many more technologies.
</p>
<ul>
<li>JavaScript Fundamentals</li>
<li>React Essentials</li>
<li>Data Structures and Algorithms</li>
</ul>
</ModalBody>
<ModalFooter>
<Button colorScheme= "blue"
mr={3} onClick={onClose}>
Close
</Button>
</ModalFooter>
</ModalContent>
</Modal>
</Box>
</ChakraProvider>
);
}
export default App;
|
Step to run the Application:
npm start
Output: Now go to http://localhost:3000 in your browser:
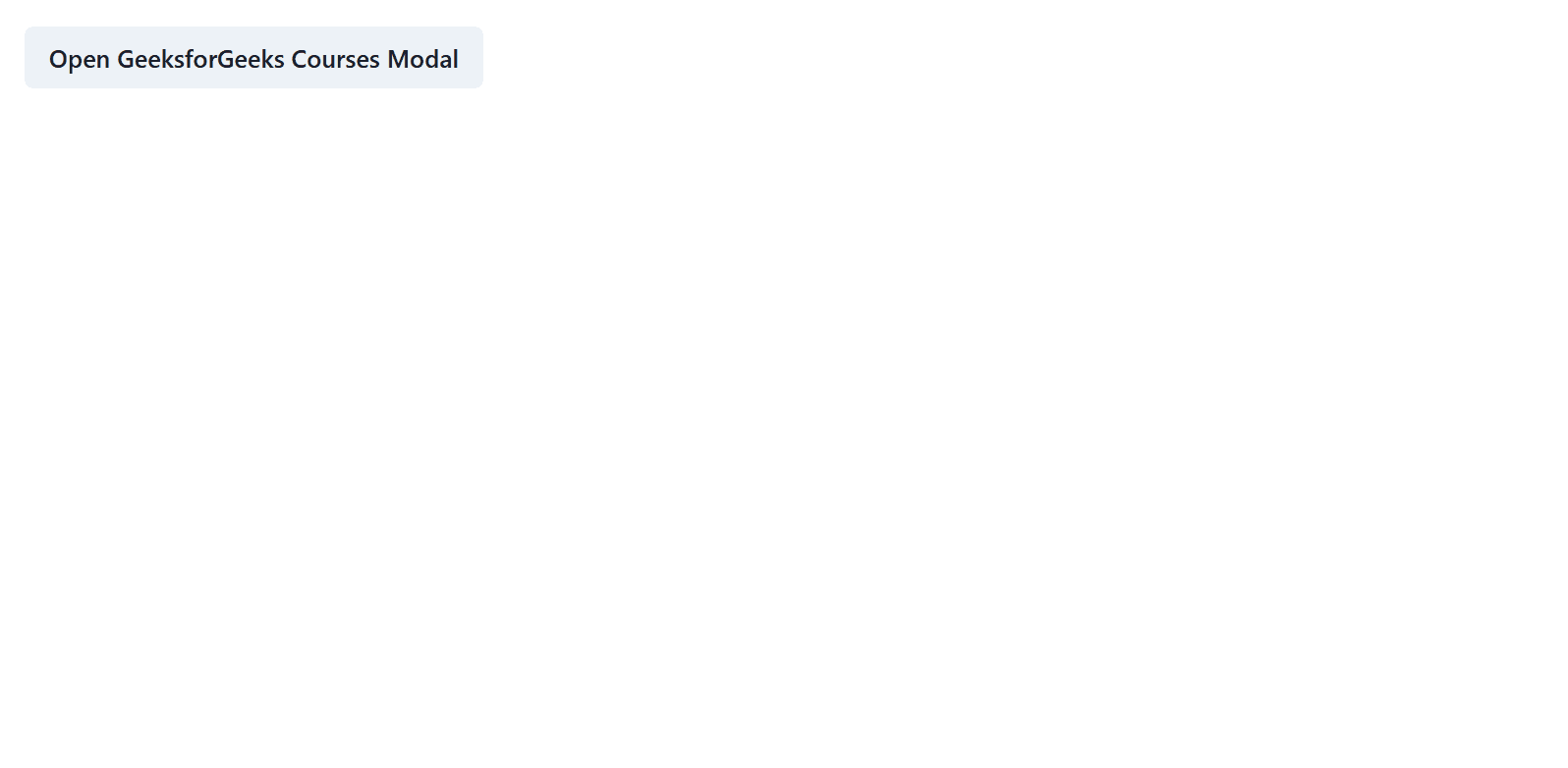
Share your thoughts in the comments
Please Login to comment...