Chakra UI Other Close Button
Last Updated :
13 Feb, 2024
Chakra UI Close Button is an icon button with a close icon. It provides a consistent way to show close buttons across our web application. The close button triggers the close functionality in other components. It comes in three sizes: small, medium and large.
Prerequisites:
Approach:
We will create a simple alert and we will use the CloseButton to show a close icon which will close the alert on clicking upon it. We can pass a click handler by using the onClick property of the CloseButton component of Chakra UI.
Setting up React Application and Installing Chakra UI:
Step 1: Create a react application using the create-react-app.
npx create-react-app my-chakraui-app
Step 2: Move to the created project folder (my-chakraui-app).
cd my-chakraui-app
Step 3: After Creating the react app install the needed packages using below command
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
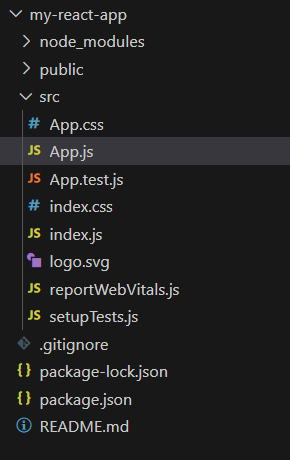
The dependencies in the package.json file are:
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example:Â Below are the code snippets for the respective files.
Javascript
import { ChakraProvider, Text } from "@chakra-ui/react" ;
import "./App.css" ;
import CloseButtonExample from "./CloseButtonExample" ;
function App() {
return (
<ChakraProvider>
<Text
color= "#2F8D46"
fontSize= "2rem"
textAlign= "center"
fontWeight= "600"
mt= "1rem" >
GeeksforGeeks
</Text>
<Text
color= "#000"
fontSize= "1rem"
textAlign= "center"
fontWeight= "500"
mb= "2rem" >
Chakra UI CloseButton
</Text>
<CloseButtonExample />
</ChakraProvider>
);
}
export default App;
|
Javascript
import React from 'react' ;
import {
CloseButton, Box,
Alert, AlertIcon
} from '@chakra-ui/react' ;
export default function CloseButtonExample() {
const [showAlert, setShowAlert] = React.useState( true );
const onAlertClose = () => setShowAlert( false );
return (
<Box display= "flex" justifyContent= "center" >
{
showAlert && (
<Alert status= "info" width= "30%" >
<AlertIcon />
This is an alert!
<CloseButton
position= "absolute"
right= "8px"
top= "8px"
onClick={onAlertClose}
/>
</Alert>
)
}
</Box>
);
}
|
Steps to run the application using the below command:
npm run start
Output: Now go to http://localhost:3000 in your browser to see the live application
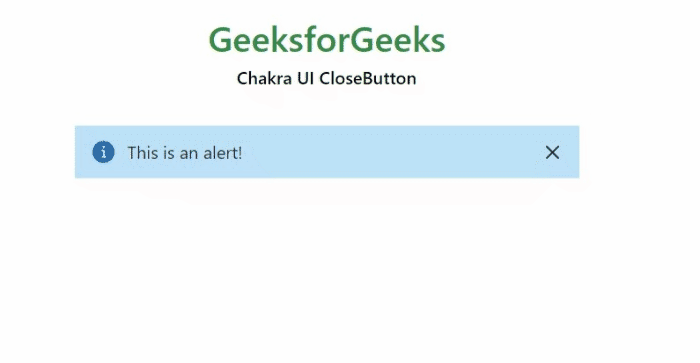
Share your thoughts in the comments
Please Login to comment...