How to Add JavaScript in HTML Document?
Last Updated :
09 Apr, 2024
JavasScript code is inserted between <script> and </script> tags when used in an HTML document. Scripts can be placed inside the body or the head section of an HTML page or inside both the head and body. We can also place JavaScript outside the HTML file which can be linked by specifying its source in the script tag.
This basic HTML code includes JavaScript implementations using various approaches.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
Add JavaScript Code inside Head
Section
</title>
</head>
<body>
<h2>
Adding JavaScript in HTML Document
</h2>
<h3 id="demo" style="color: green">
GeeksforGeeks
</h3>
<button type="button" onclick="myFun()">
Click Here
</button>
</body>
</html>
Examples of Adding JavaScript in HTML Document
JavaScript inside <head> Tag
JavaScript code is placed inside the <head> section of an HTML page and uses the <script>
element. This ensures the script is loaded and executed when the page loads.
Example: This example shows the addition of a script file inside the head section.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
Add JavaScript Code inside Head Section
</title>
<script>
function myFun() {
document.getElementById("demo")
.innerHTML = "Content changed!";
}
</script>
</head>
<body>
<h2>
Add JavaScript Code
inside Head Section
</h2>
<h3 id="demo" style="color:green;">
GeeksforGeeks
</h3>
<button type="button" onclick="myFun()">
Click Here
</button>
</body>
</html>
Output:
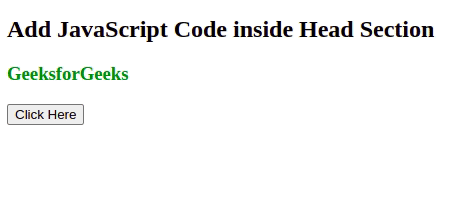
JavaScript inside <body> Tag
JavaScript Code is placed inside the body section of an HTML page and in this also we use <script> </script> tag inside and above the closing tag of </body>.
Example: This example shows showing the addition of a script file inside the body section.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
Add JavaScript Code inside Body Section
</title>
</head>
<body>
<h2>
Add JavaScript Code
inside Body Section
</h2>
<h3 id="demo" style="color:green;">
GeeksforGeeks
</h3>
<button type="button" onclick="myFun()">
Click Here
</button>
<script>
function myFun() {
document.getElementById("demo")
.innerHTML = "Content changed!";
}
</script>
</body>
</html>
Output:
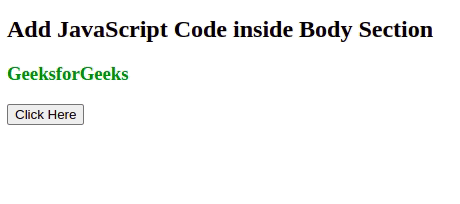
External JavaScript
JavaScript can also be used in external files. The file extension of the JavaScript file will be .js. To use an external script put the name of the script file in the src attribute of a <script> tag. External scripts cannot contain script tags.
Example: This example shows showing the linking of an external script file inside the head section.
HTML
<!DOCTYPE html>
<html>
<head>
<title>
External JavaScript
</title>
<script src="script.js"></script>
</head>
<body>
<h2>
External JavaScript
</h2>
<h3 id="demo" style="color:green;">
GeeksforGeeks
</h3>
<button type="button" onclick="myFun()">
Click Here
</button>
</body>
</html>
JavaScript
/* Filename: script.js*/
function myFun () {
document.getElementById('demo')
.innerHTML = 'Paragraph Changed'
}
Output:
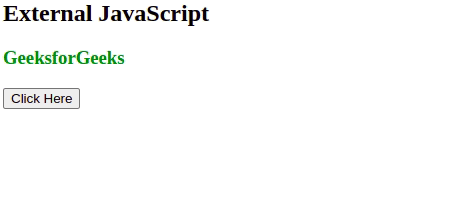
Advantages of External JavaScript
- Cached JavaScript files can speed up page loading.
- It makes JavaScript and HTML easier to read and maintain.
- It separates the HTML and JavaScript code.
- It focuses on code reusability which is one JavaScript Code that can run in various HTML files.
External JavaScript References
We can reference an external script in three ways in javascript:
src = "https://www.geeksforgeek.org/js/script.js"
src = "/js/script.js"
src = "script.js"
Share your thoughts in the comments
Please Login to comment...