Strict mode in JavaScript
Last Updated :
20 Nov, 2021
Strict Mode was a new feature in ECMAScript 5 that allows you to place a program, or a function, in a “strict” operating context. This strict context prevents certain actions from being taken and throws more exceptions. The statement “use strict”; instructs the browser to use the Strict mode, which is a reduced and safer feature set of JavaScript.
Benefits of using ‘use strict’: Strict mode makes several changes to normal JavaScript semantics.
- Strict mode eliminates some JavaScript silent errors by changing them to throw errors.
- Strict mode fixes mistakes that make it difficult for JavaScript engines to perform optimizations: strict mode code can sometimes be made to run faster than identical code that’s not strict mode.
- Strict mode prohibits some syntax likely to be defined in future versions of ECMAScript.
- It prevents, or throws errors, when relatively “unsafe” actions are taken (such as gaining access to the global object).
- It disables features that are confusing or poorly thought out.
- Strict mode makes it easier to write “secure” JavaScript.
How to use strict mode: Strict mode can be used in two ways, remember strict mode doesn’t work with block statements enclosed in {} braces.
- Used in global scope for the entire script.
- It can be applied to individual functions.
Using Strict mode for the entire script: To invoke strict mode for an entire script, put the exact statement “use strict”; (or ‘use strict’;) before any other statements.
// Whole-script strict mode syntax
'use strict';
let v = "strict mode script!";
This syntax has a flow, it isn’t possible to blindly concatenate non-conflicting scripts. Consider concatenating a strict mode script with a non-strict mode script. The entire concatenation looks strict, the inverse is also true. The non-strict plus strict looks non-strict. The concatenation of strict mode scripts with each other is fine, and concatenation of non-strict mode scripts is fine. Only concatenating strict and non-strict scripts is problematic. It is thus recommended that you enable strict mode on a function-by-function basis (at least during the transition period).
Using Strict mode for a function: Likewise, to invoke strict mode for a function, put the exact statement “use strict”; (or ‘use strict’;) in the function’s body before any other statements.
function strict() {
// Function-level strict mode syntax
'use strict';
function nested() { return 'Javascript on GeeksforGeeks'; }
return "strict mode function! " + nested();
}
function notStrict() { return "non strict function"; }
Examples of using Strict mode:
- Example: In normal JavaScript, mistyping a variable name creates a new global variable. In strict mode, this will throw an error, making it impossible to accidentally create a global variable.
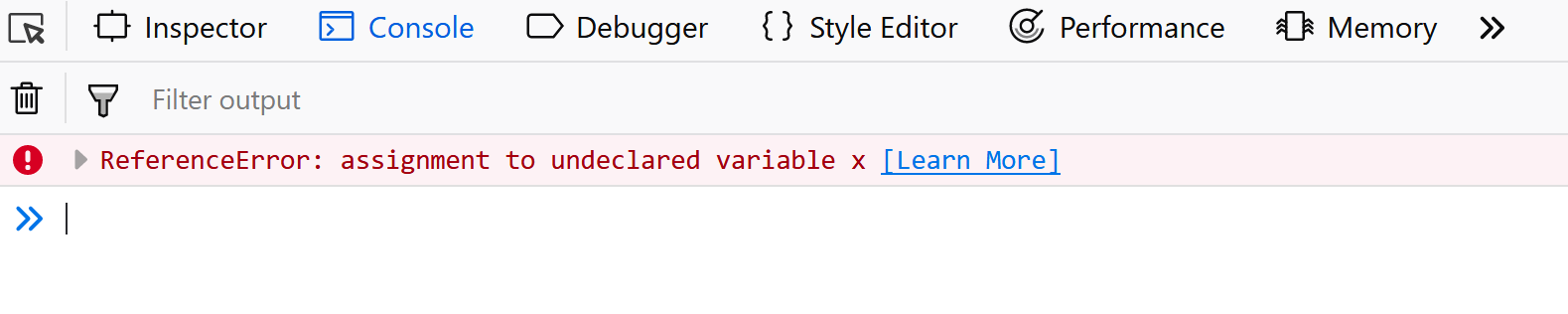
- Example: Using strict mode, don’t allow to use a variable without declaring it.
Javascript
'use strict' ;
x = {p1:10, p2:20};
|
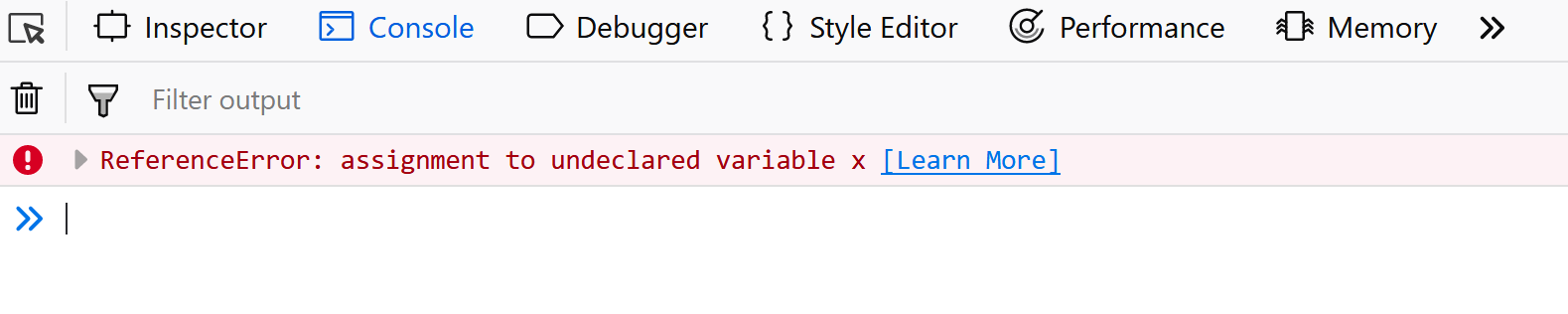
- Example: Deleting a variable (or object) and a function is not allowed.
Javascript
'use strict' ;
let x = 3.14;
'use strict' ;
function x(p1, p2) {};
delete x;
|
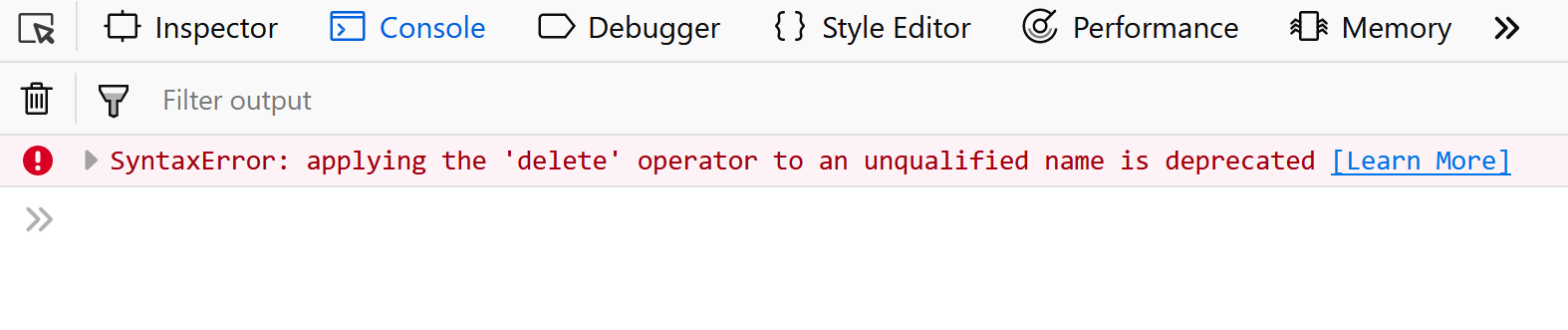
- Example: Duplicating a parameter name is not allowed.
Javascript
'use strict' ;
function x(p1, p1) {};
|
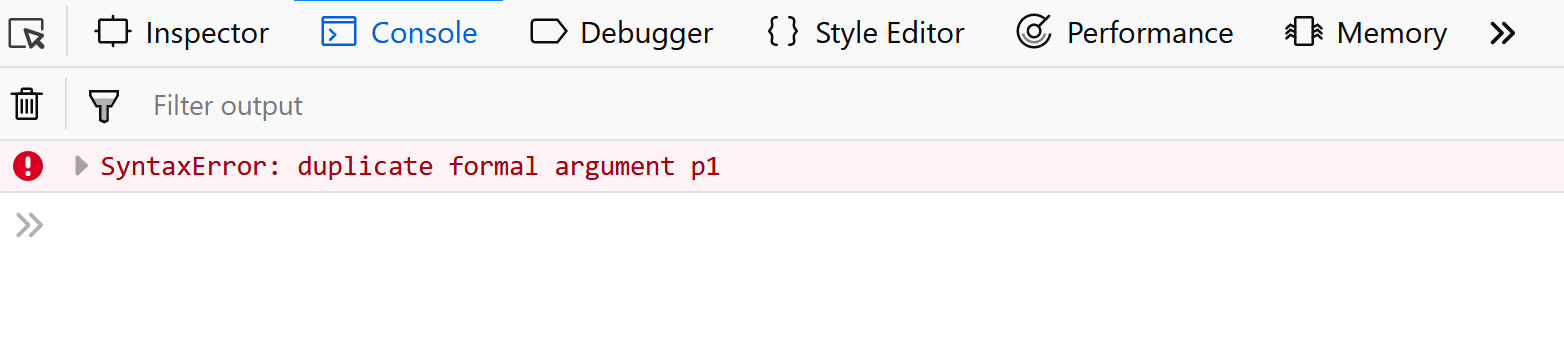
- Example: Octal numeric literals are not allowed.
Javascript
'use strict' ;
let x = 010;
|
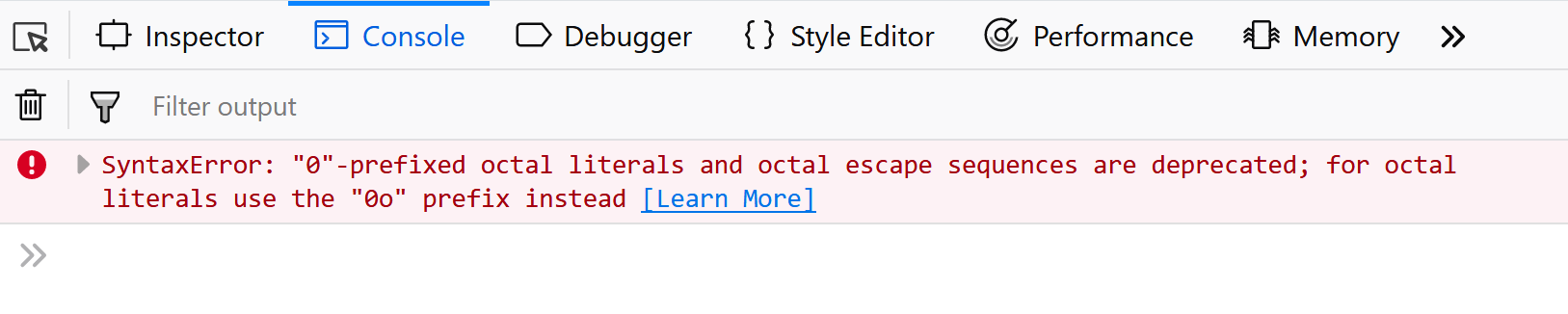
- Example: Escape characters are not allowed.
Javascript
'use strict' ;
let x = \010;
|
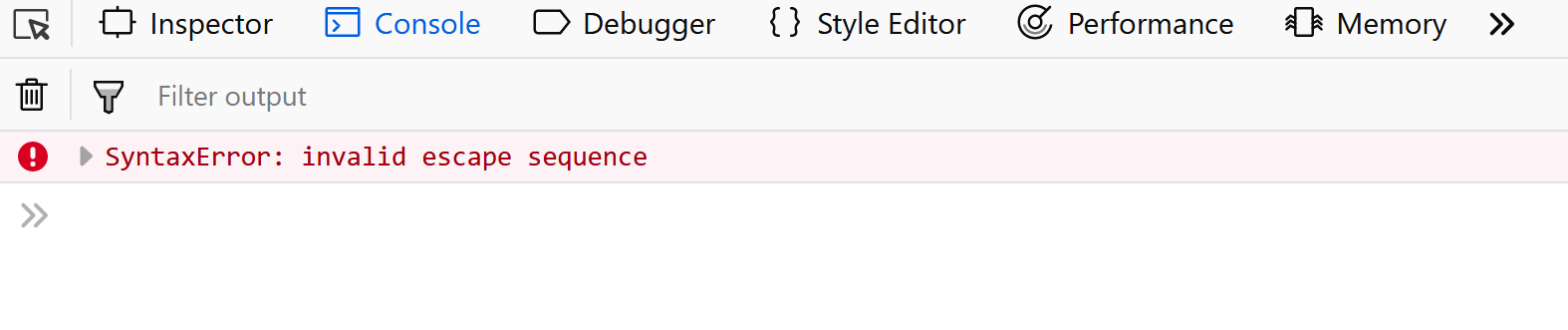
- Example: Writing to a read-only property is not allowed.
Javascript
'use strict' ;
let obj = {};
Object.defineProperty(obj, "x" , {value:0, writable: false });
obj.x = 3.14;
|

- Example: Writing to a get-only property is not allowed.
Javascript
'use strict' ;
let obj = {get x() { return 0} };
obj.x = 3.14;
|
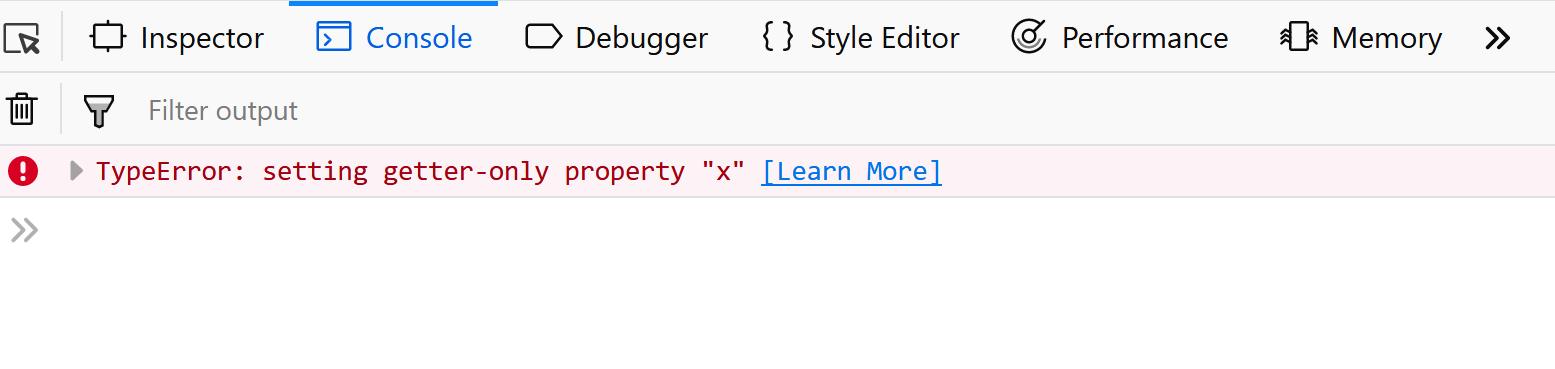
- Example: Deleting an undeletable property is not allowed.
Javascript
'use strict' ;
delete Object.prototype;
|

- Example: The string “eval” cannot be used as a variable.
Javascript
'use strict' ;
let eval = 3.14;
|
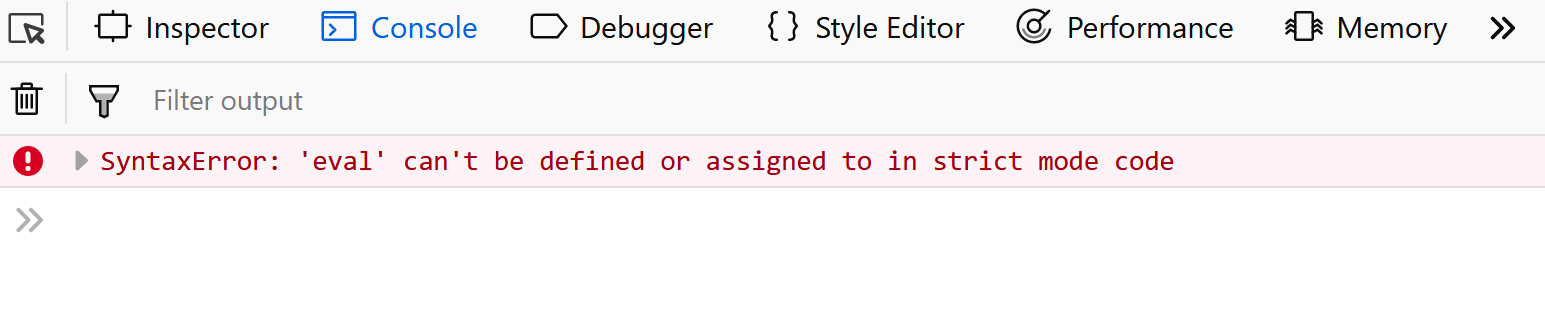
- Example: The string “arguments” cannot be used as a variable.
Javascript
'use strict' ;
let arguments = 3.14;
|

- Example: The with statement is not allowed.
Javascript
'use strict' ;
with (Math){x = cos(2)};
|

Note: In function calls like f(), this value was the global object. In strict mode, it is now undefined. In normal JavaScript, a developer will not receive any error feedback assigning values to non-writable properties.
Share your thoughts in the comments
Please Login to comment...