How to Call an Action From Within Another Action in VueJS ?
Last Updated :
03 Jan, 2024
In Vue.JS applications, we can call one action from within another as per the requirement for managing complex workflows. In this article, we will see the two different approaches with their practical implementation, through which we can perform this calling action.
Approach 1: Using Methods
In this approach, we are using the Vue.js Methods, where, we have the showMessage method which is triggered when the Show Message button is clicked. It also updates the displayed message by checking and incorporating the value that is entered in the input field. This demonstrates how one action can trigger another action.
Syntax:
new Vue({
el: '#app',
data: {
// data properties
},
methods: {
yourMethodName() {
// method logic
}
}
});
Example: The below code example practically explains the methods approach to call action from within another action.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
< script src =
</ script >
< style >
#app {
text-align: center;
}
#app h1 {
color: green;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 >GeeksforGeeks</ h1 >
< h4 >
Approach 1: Using Methods
</ h4 >
< h2 >{{ msg }}</ h2 >
< input type = "text"
v-model = "newMsg"
placeholder = "Enter new msg" >
< button @ click = "showMessage" >
Show Message
</ button >
</ div >
< script >
new Vue({
el: '#app',
data:
{
msg: 'Hello, GFG!',
newMsg: ''
},
methods: {
showMessage() {
if (this.
newMsg.trim()
!== '') {
this.msg = this.newMsg;
alert(
'Updated Message: ' +
this.msg);
} else {
alert(
'Please enter a new msg.');
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
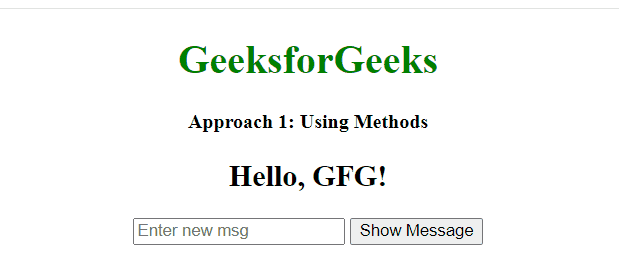
Approach 2: Using Event Bus
In this approach, we are using the Event Bus for communication between various actions. When we click on the Increment Counter button, that event is been triggered, where the counter value is updated and also notified to other components. When the Show Message button is clicked, a separate event is triggered, which demonstrate s how actions can invoked directly through the events.
Syntax:
// event Bus
const bus = new Vue();
// emitting an event from a component
bus.$emit('custom-event');
// listening for the event in another component
bus.$on('custom-event' => {
// handle the event in the component
});
Example: The below example practically implements the Event Bus method to call an action within another action.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 2</ title >
< script src =
</ script >
< style >
#app {
text-align: center;
}
#app h1 {
color: green;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 >GeeksforGeeks</ h1 >
< h4 >
Approach 2: Using Event Bus
</ h4 >
< h4 >
Counter: {{ count }}
</ h4 >
< button @ click = "increaseCnt" >
Increment Counter
</ button >
< button @ click = "showMsg" >
Show Message
</ button >
</ div >
< script >
const bus = new Vue();
new Vue({
el: '#app',
data: {
count: 0
},
methods: {
increaseCnt() {
this.count++;
bus.$emit
('counterUpdated',
this.count);
},
showMsg() {
bus.$emit('showMsgEvent');
}
},
mounted() {
bus.$on('showMsgEvent', () => {
alert('Hello from Vue!');
});
bus.$on('counterUpdated',
(value) => {
alert(
'Counter Updated! New Value: '
+ value);
});
}
});
</ script >
</ body >
</ html >
|
Output:
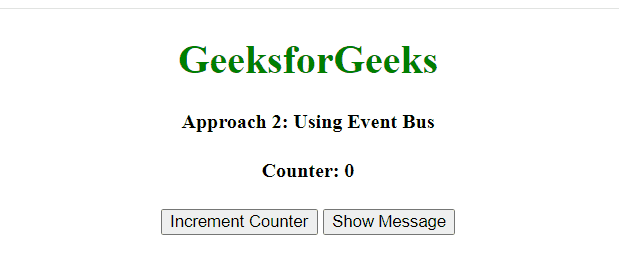
Share your thoughts in the comments
Please Login to comment...